
Library (as yet untested) for use with the Mikroelectronics tft_proto screens
Dependencies: SPI_TFT_ILI9341 TFT_fonts mbed
Fork of TFT_banggood by
main.cpp
00001 // example to test the TFT Display from Mikroelectronika 00002 00003 #include "stdio.h" 00004 #include "mbed.h" 00005 #include "SPI_TFT_ILI9341.h" 00006 #include "string" 00007 #include "Arial12x12.h" 00008 #include "Arial24x23.h" 00009 #include "Arial28x28.h" 00010 #include "font_big.h" 00011 00012 00013 Serial pc (USBTX,USBRX); 00014 00015 00016 00017 00018 // the display has a backlight switch on board 00019 DigitalOut LCD_LED(PTA13); // may not be needed on mikroelectronika board 00020 DigitalOut pwr(PTD7); // ditto 00021 00022 // the TFT is connected to SPI pin 5-7 00023 //SPI_TFT_ILI9341 TFT(p5, p6, p7, p8, p9, p10,"TFT"); // mosi, miso, sclk, cs, reset, dc for lpc1768 00024 SPI_TFT_ILI9341 TFT(PTD2, PTD3, PTD1, PTD5, PTD0, PTA13,"TFT"); // mosi, miso, sclk, cs, reset, dc for frdmkl25z 00025 //NB better combination to use a coherent 2x4 block for lcd 00026 // SPI_TFT_ILI9341 TFT(PTD2, PTD3, PTD1, PTA16, PTA17, PTC16,"TFT"); // mosi, miso, sclk, cs, reset, dc for frdmkl25z 00027 // DigitalOut LCD_LED(PTC17); 00028 00029 00030 // Subroutine to read the x location of the touch point 00031 // need to set x+ to 3V and ground x- then read analogue voltage on ym 00032 //nb need to add a check for actual touch as opposed to random crap 00033 int readX() 00034 { 00035 AnalogIn yp(PTB2); 00036 AnalogIn ym(PTB3); 00037 DigitalOut xp(PTB0); 00038 DigitalOut xm(PTB1); 00039 00040 xp=1; // set positive sdie of x high 00041 xm=0; 00042 // dont need to do anyhting to set low side as it should be fine. 00043 // but do need to disconnect yp 00044 //yp.PinMode(PullNone) 00045 int xval=(int)ym.read_u16(); // get value 00046 return(xval); 00047 } 00048 // subroutine to read y values - has different pin functions .. 00049 int readY() 00050 { 00051 DigitalOut yp(PTB2); 00052 DigitalOut ym(PTB3); 00053 AnalogIn xp(PTB0); 00054 AnalogIn xm(PTB1); 00055 00056 yp=1; // set positive sdie of x high 00057 ym=0; 00058 // dont need to do anyhting to set low side as it should be fine. 00059 // but do need to disconnect yp 00060 //yp.PinMode(PullNone) 00061 int yval=(int)xm.read_u16(); // get value 00062 return(yval); 00063 } 00064 00065 00066 00067 int main() 00068 { 00069 pc.baud(115200); 00070 00071 int xpos=0,ypos=0,xp=0,yp=0; 00072 pwr=1; 00073 wait(0.2); 00074 00075 int i; 00076 LCD_LED = 1; // backlight on 00077 00078 TFT.claim(stdout); // send stdout to the TFT display 00079 TFT.set_orientation(1); 00080 TFT.background(Black); // set background to black 00081 TFT.foreground(White); // set chars to white 00082 TFT.cls(); // clear the screen 00083 00084 //first show the 4 directions 00085 TFT.set_orientation(0); 00086 TFT.background(Black); 00087 TFT.cls(); 00088 00089 TFT.set_font((unsigned char*) Arial12x12); 00090 TFT.locate(0,0); 00091 printf(" 0 Hello Mbed 0"); 00092 TFT.set_orientation(1); 00093 TFT.locate(0,0); 00094 printf(" 1 Hello Mbed 1"); 00095 TFT.set_orientation(2); 00096 TFT.locate(0,0); 00097 printf(" 2 Hello Mbed 2"); 00098 TFT.set_orientation(3); 00099 TFT.locate(0,0); 00100 printf(" 3 Hello Mbed 3"); 00101 TFT.set_orientation(3); 00102 TFT.set_font((unsigned char*) Arial24x23); 00103 TFT.locate(50,100); 00104 TFT.printf("TFT orientation 3"); 00105 TFT.set_orientation(1); 00106 for(i=0; i<10; i++) { 00107 wait(1); // wait one seconds 00108 TFT.locate(50,160); 00109 TFT.printf("count %d",i); 00110 } 00111 TFT.set_orientation(1); 00112 TFT.cls(); 00113 // LCD_LED = 1; 00114 TFT.circle(120,120,10,0xffff); 00115 while(1==1) { 00116 00117 xpos=readX(); 00118 ypos=readY(); 00119 pc.printf("xpos=%d\t,\typo=%d",xpos,ypos); 00120 xp=(240*xpos)/60000; 00121 yp=(320*ypos)/60000; 00122 pc.printf("\txp=%d\t,\typo=%d\n\r",xp,yp); 00123 if(xp>5 && yp>5) TFT.circle(xp,yp,5,Yellow); 00124 wait(0.1); 00125 00126 } 00127 00128 }
Generated on Mon Jul 18 2022 21:54:38 by
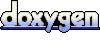