
The preloaded firmware shipped on the Roulette.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 ** This software can be freely used, even comercially, as highlighted in the license. 00003 ** 00004 ** Copyright 2014 GHI Electronics, LLC 00005 ** 00006 ** Licensed under the Apache License, Version 2.0 (the "License"); 00007 ** you may not use this file except in compliance with the License. 00008 ** You may obtain a copy of the License at 00009 ** 00010 ** http://www.apache.org/licenses/LICENSE-2.0 00011 ** 00012 ** Unless required by applicable law or agreed to in writing, software 00013 ** distributed under the License is distributed on an "AS IS" BASIS, 00014 ** WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 ** See the License for the specific language governing permissions and 00016 ** limitations under the License. 00017 ** 00018 **/ 00019 00020 #include "mbed.h" 00021 #include "TextLCD.h" 00022 00023 SPI spi(P0_21, P0_22, P1_15); // mosi, miso, sclk 00024 TextLCD lcd(P0_23, P0_16, P0_14, P0_13, P0_12, P0_11); // RS, E, D4-D7, LCDType=LCD16x2, BL=NC, E2=NC, LCDTCtrl=HD44780 00025 00026 DigitalOut compassLED[] = {(P0_19), (P0_9), (P0_18), (P0_8)}; // The South, West, North, East LEDs 00027 DigitalOut OutputEnable(P0_5); 00028 DigitalOut Latch(P0_4); 00029 DigitalOut Buzzer(P0_22); 00030 00031 DigitalIn userButton1(P0_17, PullUp); 00032 InterruptIn selectButton(P0_1); 00033 00034 enum CompassPoints { SOUTH = 0, WEST = 1, NORTH = 2, EAST = 3 }; 00035 00036 void initilize(); 00037 void printToLCD(const char* text); 00038 void RotateLEDs(float delay); 00039 void SetLED(uint32_t LEDBitMap); 00040 bool ReadPlayerButton(); 00041 bool ReadSelectButton(); 00042 void soundBuzzer(int Hz); 00043 void splash(); 00044 void gameLoop(); 00045 int checkTargetHit(int currentLED); 00046 void displayHighestLevel(); 00047 void score(); 00048 void winner(); 00049 00050 int level; 00051 int highestLevel; 00052 int rotateFactor; 00053 int LEDBitmap; 00054 char debugString [50]; 00055 00056 void initilize() 00057 { 00058 level = 1; 00059 highestLevel = 1; 00060 rotateFactor = 5; 00061 LEDBitmap = 1; 00062 00063 OutputEnable = false; 00064 selectButton.mode(PullUp); 00065 selectButton.rise(&displayHighestLevel); 00066 00067 lcd.putc('R'); 00068 lcd.putc('o'); 00069 lcd.putc('u'); 00070 lcd.putc('l'); 00071 lcd.putc('e'); 00072 lcd.putc('t'); 00073 lcd.putc('t'); 00074 lcd.putc('e'); 00075 00076 spi.format(16,0); // Sets the bits to 16 to write; sets the clock's Polarity and Phase 00077 spi.frequency(10 * 1000 * 1000); // Sets SPI clock to 20,000,000 Hz or 10MHz 00078 } 00079 00080 void printToLCD(const char* text) 00081 { 00082 // Will add a text scrolling function here if the text is 00083 // greater than 8 characters. 00084 if (strlen(text) > 8) { 00085 return; 00086 } 00087 00088 int x = 0; 00089 lcd.cls(); 00090 00091 while (text[x] != '\0') { 00092 lcd.putc(text[x]); 00093 x++; 00094 } 00095 } 00096 00097 void SetLED(uint32_t LEDBitMap) 00098 { 00099 uint16_t shiftMap = 0; 00100 00101 // Ring Segments - all on linked shift registers 00102 shiftMap = (LEDBitMap >> 1) &0xf; 00103 shiftMap |= ((LEDBitMap >> 6) &0xf) << 4; 00104 shiftMap |= ((LEDBitMap >> 11) &0xf) << 8; 00105 shiftMap |= ((LEDBitMap >> 16) &0xf) << 12; 00106 00107 spi.write(shiftMap); 00108 00109 Latch = true; 00110 Latch = false; 00111 00112 // Set the LEDs connected directly to the processor 00113 compassLED[SOUTH] = LEDBitMap &0x1; 00114 compassLED[WEST] = (LEDBitMap >> 5) &0x1; 00115 compassLED[NORTH] = (LEDBitMap >> 10) &0x1; 00116 compassLED[EAST] = (LEDBitMap >> 15) &0x1; 00117 } 00118 00119 bool ReadPlayerButton() 00120 { 00121 // Logic High = True, Logic Low = False. 00122 // userButton1 is set with an internal Pull-up which makes 00123 // the button true by default and false when pressed. 00124 // Must invert to make the read button function true. 00125 return !userButton1; // Logic inverted 00126 } 00127 00128 bool ReadSelectButton() 00129 { 00130 // Logic High = True, Logic Low = False. 00131 // selectButton is set with an internal Pull-up which makes 00132 // the button true by default and false when pressed. 00133 // Must invert to make the read button function true. 00134 return !selectButton; // Logic inverted 00135 } 00136 00137 void soundBuzzer(int Hz) 00138 { 00139 int ticks = Hz/64; 00140 int tickCount = 0; 00141 float frequency = 1/(float)Hz; 00142 00143 while(tickCount < ticks) { 00144 wait(frequency); 00145 Buzzer = true; 00146 wait(frequency); 00147 Buzzer = false; 00148 tickCount++; 00149 } 00150 } 00151 00152 void RotateLEDs(float delay) 00153 { 00154 if (LEDBitmap == (1 << 10)) { 00155 soundBuzzer(300); 00156 } 00157 00158 SetLED(LEDBitmap); 00159 00160 LEDBitmap <<= 1; 00161 wait(delay); 00162 if(LEDBitmap >= (1 << 20)) { 00163 LEDBitmap = 1; 00164 } 00165 } 00166 00167 void splash() 00168 { 00169 int LEDBitmap = 1; 00170 00171 for (int x = 1; x <= 20; x++) { 00172 LEDBitmap <<= 1; 00173 SetLED(LEDBitmap); 00174 soundBuzzer((x * 100) + 500); 00175 wait(0.0078125); 00176 } 00177 00178 for (int y = 0; y < 5; y++) { 00179 SetLED(0xfffff); 00180 soundBuzzer(600); 00181 wait(.03125); 00182 SetLED(0x0); 00183 wait(.03125); 00184 } 00185 } 00186 00187 int checkTargetHit(int currentLED) 00188 { 00189 wait(.5); 00190 if (currentLED == (1 << 11)) { 00191 return 3; 00192 } else if (currentLED == (1 << 10) || currentLED == (1 << 12)) { 00193 return 2; 00194 } else if (currentLED == (1 << 9) || currentLED == (1 << 13)) { 00195 return 1; 00196 } else { 00197 return 0; 00198 } 00199 } 00200 00201 void displayHighestLevel() 00202 { 00203 sprintf (debugString, "H Lev %d",highestLevel); 00204 printToLCD(debugString); 00205 wait(2.5); 00206 sprintf (debugString, "Level %d",level); 00207 printToLCD(debugString); 00208 } 00209 00210 void score() 00211 { 00212 int target = checkTargetHit(LEDBitmap); 00213 00214 switch (target) { 00215 case 3: 00216 level += 3; 00217 break; 00218 case 2: 00219 if(level < 10) { 00220 level += 2; 00221 } else { 00222 level -= 2; 00223 } 00224 break; 00225 case 1: 00226 level += 1; 00227 break; 00228 default: 00229 level--; 00230 if (level <= 1) { 00231 level = 1; 00232 } 00233 } 00234 00235 if (level > highestLevel) { 00236 highestLevel = level; 00237 } 00238 } 00239 00240 void winner() 00241 { 00242 int winCounter = 1; 00243 while (winCounter++ <= 5) { 00244 printToLCD("Winner!!"); 00245 SetLED(0xFFFFF); 00246 wait(.5); 00247 printToLCD(""); 00248 SetLED(0x0); 00249 00250 for (int x = 1; x <= 10; x++) { 00251 soundBuzzer((x * 100) + 500); 00252 wait(0.0078125); 00253 } 00254 00255 } 00256 00257 while (true) { 00258 printToLCD("Winner!!"); 00259 SetLED(0xFFFFF); 00260 wait(.5); 00261 } 00262 00263 } 00264 00265 void gameLoop() 00266 { 00267 while (true) { 00268 sprintf (debugString, "Level %d", level); 00269 printToLCD(debugString); 00270 00271 while (!ReadPlayerButton()) { 00272 RotateLEDs(1/(((float)level * rotateFactor) + 19)); 00273 } 00274 00275 score(); 00276 00277 if (level > 99) { 00278 winner(); 00279 } 00280 00281 while (ReadPlayerButton()) { // Will hold game if hold on to button to prevent cheating 00282 } 00283 } 00284 } 00285 00286 int main() 00287 { 00288 initilize(); 00289 splash(); 00290 gameLoop(); 00291 }
Generated on Mon Jul 18 2022 18:23:25 by
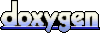