
plays a fucking annoying sound for 10 seconds and loops.
Dependencies: mbed
Fork of song_demo_PWM by
SongPlayer.h
00001 #include "mbed.h" 00002 bool keepplaying = true; 00003 // new class to play a note on Speaker based on PwmOut class 00004 class SongPlayer 00005 { 00006 public: 00007 SongPlayer(PinName pin) : _pin(pin) { 00008 // _pin(pin) means pass pin to the constructor 00009 } 00010 // class method to play a note based on PwmOut class 00011 void PlaySong(float frequency[], float duration[], float volume=1.0) { 00012 vol = volume; 00013 notecount = 0; 00014 _pin.period(1.0/frequency[notecount]); 00015 _pin = volume/2.0; 00016 noteduration.attach(this,&SongPlayer::nextnote, duration[notecount]); 00017 // setup timer to interrupt for next note to play 00018 frequencyptr = frequency; 00019 durationptr = duration; 00020 //returns after first note starts to play 00021 } 00022 void nextnote(); 00023 private: 00024 Timeout noteduration; 00025 PwmOut _pin; 00026 int notecount; 00027 float vol; 00028 float * frequencyptr; 00029 float * durationptr; 00030 }; 00031 //Interrupt Routine to play next note 00032 void SongPlayer::nextnote() 00033 { 00034 if(keepplaying==true){ 00035 _pin = 0.0; 00036 notecount++; //setup next note in song 00037 if (durationptr[notecount]!=0.0) { 00038 _pin.period(1.0/frequencyptr[notecount]); 00039 noteduration.attach(this,&SongPlayer::nextnote, durationptr[notecount]); 00040 _pin = vol/2.0; 00041 } else 00042 _pin = 0.0; //turn off on last note 00043 } 00044 }
Generated on Thu Jul 14 2022 21:01:01 by
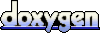