Library for LoRa communication using MultiTech MDOT.
Dependents: mDot_test_rx adc_sensor_lora mDotEVBM2X mDot_AT_firmware ... more
mDot.h
00001 // TODO: add license header 00002 00003 #ifndef MDOT_H 00004 #define MDOT_H 00005 00006 #include "mbed.h" 00007 #include "rtos.h" 00008 #include <vector> 00009 #include <map> 00010 #include <string> 00011 00012 class mDotEvent; 00013 class LoRaMacEvent ; 00014 class LoRaConfig; 00015 class LoRaMac; 00016 class MdotRadio; 00017 00018 class mDot { 00019 00020 private: 00021 00022 mDot(); 00023 ~mDot(); 00024 00025 static void idle(void const* args) { 00026 while (1) 00027 __WFI(); 00028 } 00029 00030 void setLastError(const std::string& str); 00031 00032 static bool validateBaudRate(const uint32_t& baud); 00033 static bool validateFrequencySubBand(const uint8_t& band); 00034 bool validateDataRate(const uint8_t& dr); 00035 00036 int32_t joinBase(const uint32_t& retries); 00037 int32_t sendBase(const std::vector<uint8_t>& data, const bool& confirmed = false, const bool& blocking = true, const bool& highBw = false); 00038 void waitForPacket(); 00039 void waitForLinkCheck(); 00040 00041 void setActivityLedState(const uint8_t& state); 00042 uint8_t getActivityLedState(); 00043 00044 void blinkActivityLed(void) { 00045 if (_activity_led) { 00046 int val = _activity_led->read(); 00047 _activity_led->write(!val); 00048 } 00049 } 00050 00051 mDot(const mDot&); 00052 mDot& operator=(const mDot&); 00053 00054 uint32_t RTC_ReadBackupRegister(uint32_t RTC_BKP_DR); 00055 void RTC_WriteBackupRegister(uint32_t RTC_BKP_DR, uint32_t Data); 00056 00057 void wakeup(); 00058 00059 void enterStopMode(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM); 00060 void enterStandbyMode(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM); 00061 00062 static mDot* _instance; 00063 00064 LoRaMac* _mac; 00065 MdotRadio* _radio; 00066 LoRaMacEvent * _events; 00067 LoRaConfig* _config; 00068 Thread _idle_thread; 00069 std::string _last_error; 00070 static const uint32_t _baud_rates[]; 00071 uint8_t _activity_led_state; 00072 Ticker _tick; 00073 DigitalOut* _activity_led; 00074 bool _activity_led_enable; 00075 PinName _activity_led_pin; 00076 bool _activity_led_external; 00077 uint8_t _linkFailCount; 00078 uint8_t _class; 00079 InterruptIn* _wakeup; 00080 PinName _wakeup_pin; 00081 00082 typedef enum { 00083 OFF, 00084 ON, 00085 BLINK, 00086 } state; 00087 00088 public: 00089 00090 typedef enum { 00091 FM_APPEND = (1 << 0), 00092 FM_TRUNC = (1 << 1), 00093 FM_CREAT = (1 << 2), 00094 FM_RDONLY = (1 << 3), 00095 FM_WRONLY = (1 << 4), 00096 FM_RDWR = (FM_RDONLY | FM_WRONLY), 00097 FM_DIRECT = (1 << 5) 00098 } FileMode; 00099 00100 typedef enum { 00101 MDOT_OK = 0, 00102 MDOT_INVALID_PARAM = -1, 00103 MDOT_TX_ERROR = -2, 00104 MDOT_RX_ERROR = -3, 00105 MDOT_JOIN_ERROR = -4, 00106 MDOT_TIMEOUT = -5, 00107 MDOT_NOT_JOINED = -6, 00108 MDOT_ENCRYPTION_DISABLED = -7, 00109 MDOT_NO_FREE_CHAN = -8, 00110 MDOT_TEST_MODE = -9, 00111 MDOT_NO_ENABLED_CHAN = -10, 00112 MDOT_AGGREGATED_DUTY_CYCLE = -11, 00113 MDOT_ERROR = -1024, 00114 } mdot_ret_code; 00115 00116 enum JoinMode { 00117 MANUAL, 00118 OTA, 00119 AUTO_OTA, 00120 PEER_TO_PEER 00121 }; 00122 00123 enum Mode { 00124 COMMAND_MODE, 00125 SERIAL_MODE 00126 }; 00127 00128 enum RX_Output { 00129 HEXADECIMAL, 00130 BINARY 00131 }; 00132 00133 enum DataRates { 00134 DR0, 00135 DR1, 00136 DR2, 00137 DR3, 00138 DR4, 00139 DR5, 00140 DR6, 00141 DR7, 00142 DR8, 00143 DR9, 00144 DR10, 00145 DR11, 00146 DR12, 00147 DR13, 00148 DR14, 00149 DR15, 00150 SF_12 = 16, 00151 SF_11, 00152 SF_10, 00153 SF_9, 00154 SF_8, 00155 SF_7, 00156 SF_7H, 00157 SF_FSK 00158 }; 00159 00160 enum FrequencyBands { 00161 FB_868, // EU868 00162 FB_915 // US915 00163 }; 00164 00165 enum FrequencySubBands { 00166 FSB_ALL, 00167 FSB_1, 00168 FSB_2, 00169 FSB_3, 00170 FSB_4, 00171 FSB_5, 00172 FSB_6, 00173 FSB_7, 00174 FSB_8 00175 }; 00176 00177 enum JoinByteOrder { 00178 LSB, 00179 MSB 00180 }; 00181 00182 enum wakeup_mode { 00183 RTC_ALARM, 00184 INTERRUPT, 00185 RTC_ALARM_OR_INTERRUPT 00186 }; 00187 00188 enum UserBackupRegs { 00189 UBR0, 00190 UBR1, 00191 UBR2, 00192 UBR3, 00193 UBR4, 00194 UBR5, 00195 UBR6, 00196 UBR7, 00197 UBR8, 00198 UBR9 00199 }; 00200 00201 typedef struct { 00202 int16_t fd; 00203 char name[30]; 00204 uint32_t size; 00205 } mdot_file; 00206 00207 typedef struct { 00208 uint32_t Up; 00209 uint32_t Down; 00210 uint32_t Joins; 00211 uint32_t JoinFails; 00212 uint32_t MissedAcks; 00213 } mdot_stats; 00214 00215 typedef struct { 00216 int16_t last; 00217 int16_t min; 00218 int16_t max; 00219 int16_t avg; 00220 } rssi_stats; 00221 00222 typedef struct { 00223 int16_t last; 00224 int16_t min; 00225 int16_t max; 00226 int16_t avg; 00227 } snr_stats; 00228 00229 typedef struct { 00230 bool status; 00231 int32_t dBm; 00232 uint32_t gateways; 00233 std::vector<uint8_t> payload; 00234 } link_check; 00235 00236 typedef struct { 00237 int32_t status; 00238 int16_t rssi; 00239 int16_t snr; 00240 } ping_response; 00241 00242 static const uint8_t MaxLengths_915[]; 00243 static const uint8_t MaxLengths_868[]; 00244 00245 static std::string JoinModeStr(uint8_t mode); 00246 static std::string ModeStr(uint8_t mode); 00247 static std::string RxOutputStr(uint8_t format); 00248 static std::string DataRateStr(uint8_t rate); 00249 static std::string FrequencyBandStr(uint8_t band); 00250 static std::string FrequencySubBandStr(uint8_t band); 00251 00252 uint32_t UserRegisters[10]; 00253 00254 /** Get a handle to the singleton object 00255 * @returns pointer to mDot object 00256 */ 00257 static mDot* getInstance(); 00258 00259 void setEvents(mDotEvent* events); 00260 00261 /** Get library version information 00262 * @returns string containing library version information 00263 */ 00264 std::string getId(); 00265 00266 /** Perform a soft reset of the system 00267 */ 00268 void resetCpu(); 00269 00270 /** Reset config to factory default 00271 */ 00272 void resetConfig(); 00273 00274 /** Save config data to non volatile memory 00275 * @returns true if success, false if failure 00276 */ 00277 bool saveConfig(); 00278 00279 /** Set the log level for the library 00280 * options are: 00281 * NONE_LEVEL - logging is off at this level 00282 * FATAL_LEVEL - only critical errors will be reported 00283 * ERROR_LEVEL 00284 * WARNING_LEVEL 00285 * INFO_LEVEL 00286 * DEBUG_LEVEL 00287 * TRACE_LEVEL - every detail will be reported 00288 * @param level the level to log at 00289 * @returns MDOT_OK if success 00290 */ 00291 int32_t setLogLevel(const uint8_t& level); 00292 00293 /** Get the current log level for the library 00294 * @returns current log level 00295 */ 00296 uint8_t getLogLevel(); 00297 00298 /** Seed pseudo RNG in LoRaMac layer, uses random value from radio RSSI reading by default 00299 * @param seed for RNG 00300 */ 00301 void seedRandom(uint32_t seed); 00302 00303 /** Enable or disable the activity LED. 00304 * @param enable true to enable the LED, false to disable 00305 */ 00306 void setActivityLedEnable(const bool& enable); 00307 00308 /** Find out if the activity LED is enabled 00309 * @returns true if activity LED is enabled, false if disabled 00310 */ 00311 bool getActivityLedEnable(); 00312 00313 /** Use a different pin for the activity LED. 00314 * The default is XBEE_RSSI. 00315 * @param pin the new pin to use 00316 */ 00317 void setActivityLedPin(const PinName& pin); 00318 00319 /** Use an external DigitalOut object for the activity LED. 00320 * The pointer must stay valid! 00321 * @param pin the DigitalOut object to use 00322 */ 00323 void setActivityLedPin(DigitalOut* pin); 00324 00325 /** Find out what pin the activity LED is on 00326 * @returns the pin the activity LED is using 00327 */ 00328 PinName getActivityLedPin(); 00329 00330 /** Returns boolean indicative of start-up from standby mode 00331 * @returns true if dot woke from standby 00332 */ 00333 bool getStandbyFlag(); 00334 00335 /** Add a channel frequencies currently in use 00336 * @returns MDOT_OK 00337 */ 00338 int32_t addChannel(uint8_t index, uint32_t frequency, uint8_t datarateRange); 00339 00340 /** Get list of channel frequencies currently in use 00341 * @returns vector of channels currently in use 00342 */ 00343 std::vector<uint32_t> getChannels(); 00344 00345 /** Get list of channel datarate ranges currently in use 00346 * @returns vector of datarate ranges currently in use 00347 */ 00348 std::vector<uint8_t> getChannelRanges(); 00349 00350 /** Get list of channel frequencies in config file to be used as session defaults 00351 * @returns vector of channels in config file 00352 */ 00353 std::vector<uint32_t> getConfigChannels(); 00354 00355 /** Get list of channel datarate ranges in config file to be used as session defaults 00356 * @returns vector of datarate ranges in config file 00357 */ 00358 std::vector<uint8_t> getConfigChannelRanges(); 00359 00360 /** Get frequency band 00361 * @returns FB_915 if configured for United States, FB_868 if configured for Europe 00362 */ 00363 uint8_t getFrequencyBand(); 00364 00365 /** Set frequency sub band 00366 * only applicable if frequency band is set for United States (FB_915) 00367 * sub band 0 will allow the radio to use all 64 channels 00368 * sub band 1 - 8 will allow the radio to use the 8 channels in that sub band 00369 * for use with Conduit gateway and MTAC_LORA, use sub bands 1 - 8, not sub band 0 00370 * @param band the sub band to use (0 - 8) 00371 * @returns MDOT_OK if success 00372 */ 00373 int32_t setFrequencySubBand(const uint8_t& band); 00374 00375 /** Get frequency sub band 00376 * @returns frequency sub band currently in use 00377 */ 00378 uint8_t getFrequencySubBand(); 00379 00380 /** Get the datarate currently in use within the MAC layer 00381 * returns 0-15 00382 */ 00383 uint8_t getSessionDataRate(); 00384 00385 /** Enable/disable public network mode 00386 * JoinDelay will be set to (public: 5s, private: 1s) and 00387 * RxDelay will be set to 1s both can be adjusted afterwards 00388 * @param on should be true to enable public network mode 00389 * @returns MDOT_OK if success 00390 */ 00391 int32_t setPublicNetwork(const bool& on); 00392 00393 /** Get public network mode 00394 * @returns true if public network mode is enabled 00395 */ 00396 bool getPublicNetwork(); 00397 00398 /** Get the device ID 00399 * @returns vector containing the device ID (size 8) 00400 */ 00401 std::vector<uint8_t> getDeviceId(); 00402 00403 /** Get the device port to be used for lora application data (1-223) 00404 * @returns port 00405 */ 00406 uint8_t getAppPort(); 00407 00408 /** Set the device port to be used for lora application data (1-223) 00409 * @returns MDOT_OK if success 00410 */ 00411 int32_t setAppPort(uint8_t port); 00412 00413 /** Set the device class A, B or C 00414 * @returns MDOT_OK if success 00415 */ 00416 int32_t setClass(std::string newClass); 00417 00418 /** Get the device class A, B or C 00419 * @returns MDOT_OK if success 00420 */ 00421 std::string getClass(); 00422 00423 /** Get the max packet length with current settings 00424 * @returns max packet length 00425 */ 00426 uint8_t getMaxPacketLength(); 00427 00428 /** Set network address 00429 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00430 * @param addr a vector of 4 bytes 00431 * @returns MDOT_OK if success 00432 */ 00433 int32_t setNetworkAddress(const std::vector<uint8_t>& addr); 00434 00435 /** Get network address 00436 * @returns vector containing network address (size 4) 00437 */ 00438 std::vector<uint8_t> getNetworkAddress(); 00439 00440 /** Set network session key 00441 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00442 * @param key a vector of 16 bytes 00443 * @returns MDOT_OK if success 00444 */ 00445 int32_t setNetworkSessionKey(const std::vector<uint8_t>& key); 00446 00447 /** Get network session key 00448 * @returns vector containing network session key (size 16) 00449 */ 00450 std::vector<uint8_t> getNetworkSessionKey(); 00451 00452 /** Set data session key 00453 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00454 * @param key a vector of 16 bytes 00455 * @returns MDOT_OK if success 00456 */ 00457 int32_t setDataSessionKey(const std::vector<uint8_t>& key); 00458 00459 /** Get data session key 00460 * @returns vector containing data session key (size 16) 00461 */ 00462 std::vector<uint8_t> getDataSessionKey(); 00463 00464 /** Set network name 00465 * for use with OTA & AUTO_OTA network join modes 00466 * generates network ID (crc64 of name) automatically 00467 * @param name a string of of at least 8 bytes and no more than 128 bytes 00468 * @return MDOT_OK if success 00469 */ 00470 int32_t setNetworkName(const std::string& name); 00471 00472 /** Get network name 00473 * @return string containing network name (size 8 to 128) 00474 */ 00475 std::string getNetworkName(); 00476 00477 /** Set network ID 00478 * for use with OTA & AUTO_OTA network join modes 00479 * setting network ID via this function sets network name to empty 00480 * @param id a vector of 8 bytes 00481 * @returns MDOT_OK if success 00482 */ 00483 int32_t setNetworkId(const std::vector<uint8_t>& id); 00484 00485 /** Get network ID 00486 * @returns vector containing network ID (size 8) 00487 */ 00488 std::vector<uint8_t> getNetworkId(); 00489 00490 /** Set network passphrase 00491 * for use with OTA & AUTO_OTA network join modes 00492 * generates network key (cmac of passphrase) automatically 00493 * @param name a string of of at least 8 bytes and no more than 128 bytes 00494 * @return MDOT_OK if success 00495 */ 00496 int32_t setNetworkPassphrase(const std::string& passphrase); 00497 00498 /** Get network passphrase 00499 * @return string containing network passphrase (size 8 to 128) 00500 */ 00501 std::string getNetworkPassphrase(); 00502 00503 /** Set network key 00504 * for use with OTA & AUTO_OTA network join modes 00505 * setting network key via this function sets network passphrase to empty 00506 * @param id a vector of 16 bytes 00507 * @returns MDOT_OK if success 00508 */ 00509 int32_t setNetworkKey(const std::vector<uint8_t>& id); 00510 00511 /** Get network key 00512 * @returns a vector containing network key (size 16) 00513 */ 00514 std::vector<uint8_t> getNetworkKey(); 00515 00516 /** Set join byte order 00517 * @param order 0:LSB 1:MSB 00518 */ 00519 uint32_t setJoinByteOrder(uint8_t order); 00520 00521 /** Get join byte order 00522 * @returns byte order to use in joins 0:LSB 1:MSB 00523 */ 00524 uint8_t getJoinByteOrder(); 00525 00526 /** Attempt to join network 00527 * each attempt will be made with a random datarate up to the configured datarate 00528 * JoinRequest backoff between tries is enforced to 1% for 1st hour, 0.1% for 1-10 hours and 0.01% after 10 hours 00529 * Check getNextTxMs() for time until next join attempt can be made 00530 * @returns MDOT_OK if success 00531 */ 00532 int32_t joinNetwork(); 00533 00534 /** Attempts to join network once 00535 * @returns MDOT_OK if success 00536 */ 00537 int32_t joinNetworkOnce(); 00538 00539 /** Resets current network session, essentially disconnecting from the network 00540 * has no effect for MANUAL network join mode 00541 */ 00542 void resetNetworkSession(); 00543 00544 /** Restore saved network session from flash 00545 * has no effect for MANUAL network join mode 00546 */ 00547 void restoreNetworkSession(); 00548 00549 /** Save current network session to flash 00550 * has no effect for MANUAL network join mode 00551 */ 00552 void saveNetworkSession(); 00553 00554 /** Set number of times joining will retry before giving up 00555 * @param retries must be between 0 - 255 00556 * @returns MDOT_OK if success 00557 */ 00558 int32_t setJoinRetries(const uint8_t& retries); 00559 00560 /** Set number of times joining will retry before giving up 00561 * @returns join retries (0 - 255) 00562 */ 00563 uint8_t getJoinRetries(); 00564 00565 /** Set network join mode 00566 * MANUAL: set network address and session keys manually 00567 * OTA: User sets network name and passphrase, then attempts to join 00568 * AUTO_OTA: same as OTA, but network sessions can be saved and restored 00569 * @param mode MANUAL, OTA, or AUTO_OTA 00570 * @returns MDOT_OK if success 00571 */ 00572 int32_t setJoinMode(const uint8_t& mode); 00573 00574 /** Get network join mode 00575 * @returns MANUAL, OTA, or AUTO_OTA 00576 */ 00577 uint8_t getJoinMode(); 00578 00579 /** Get network join status 00580 * @returns true if currently joined to network 00581 */ 00582 bool getNetworkJoinStatus(); 00583 00584 /** Do a network link check 00585 * application data may be returned in response to a network link check command 00586 * @returns link_check structure containing success, dBm above noise floor, gateways in range, and packet payload 00587 */ 00588 link_check networkLinkCheck(); 00589 00590 /** Set network link check count to perform automatic link checks every count packets 00591 * only applicable if ACKs are disabled 00592 * @param count must be between 0 - 255 00593 * @returns MDOT_OK if success 00594 */ 00595 int32_t setLinkCheckCount(const uint8_t& count); 00596 00597 /** Get network link check count 00598 * @returns count (0 - 255) 00599 */ 00600 uint8_t getLinkCheckCount(); 00601 00602 /** Set network link check threshold, number of link check failures or missed acks to tolerate 00603 * before considering network connection lost 00604 * @pararm count must be between 0 - 255 00605 * @returns MDOT_OK if success 00606 */ 00607 int32_t setLinkCheckThreshold(const uint8_t& count); 00608 00609 /** Get network link check threshold 00610 * @returns threshold (0 - 255) 00611 */ 00612 uint8_t getLinkCheckThreshold(); 00613 00614 /** Get/set number of failed link checks in the current session 00615 * @returns count (0 - 255) 00616 */ 00617 uint8_t getLinkFailCount(); 00618 int32_t setLinkFailCount(uint8_t count); 00619 00620 /** Set UpLinkCounter number of packets sent to the gateway during this network session (sequence number) 00621 * @returns MDOT_OK 00622 */ 00623 int32_t setUpLinkCounter(uint32_t count); 00624 00625 /** Get UpLinkCounter 00626 * @returns number of packets sent to the gateway during this network session (sequence number) 00627 */ 00628 uint32_t getUpLinkCounter(); 00629 00630 /** Set UpLinkCounter number of packets sent by the gateway during this network session (sequence number) 00631 * @returns MDOT_OK 00632 */ 00633 int32_t setDownLinkCounter(uint32_t count); 00634 00635 /** Get DownLinkCounter 00636 * @returns number of packets sent by the gateway during this network session (sequence number) 00637 */ 00638 uint32_t getDownLinkCounter(); 00639 00640 /** Enable/disable AES encryption 00641 * AES encryption must be enabled for use with Conduit gateway and MTAC_LORA card 00642 * @param on true for AES encryption to be enabled 00643 * @returns MDOT_OK if success 00644 */ 00645 int32_t setAesEncryption(const bool& on); 00646 00647 /** Get AES encryption 00648 * @returns true if AES encryption is enabled 00649 */ 00650 bool getAesEncryption(); 00651 00652 /** Get RSSI stats 00653 * @returns rssi_stats struct containing last, min, max, and avg RSSI in dB 00654 */ 00655 rssi_stats getRssiStats(); 00656 00657 /** Get SNR stats 00658 * @returns snr_stats struct containing last, min, max, and avg SNR in cB 00659 */ 00660 snr_stats getSnrStats(); 00661 00662 /** Get ms until next free channel 00663 * only applicable for European models, US models return 0 00664 * @returns time (ms) until a channel is free to use for transmitting 00665 */ 00666 uint32_t getNextTxMs(); 00667 00668 /** Get join delay in seconds 00669 * Public network defaults to 5 seconds 00670 * Private network defaults to 1 second 00671 * @returns number of seconds before join accept message is expected 00672 */ 00673 uint8_t getJoinDelay(); 00674 00675 /** Set join delay in seconds 00676 * Public network defaults to 5 seconds 00677 * Private network defaults to 1 second 00678 * @param delay number of seconds before join accept message is expected 00679 * @return MDOT_OK if success 00680 */ 00681 uint32_t setJoinDelay(uint8_t delay); 00682 00683 /** Get rx delay in seconds 00684 * Defaults to 1 second 00685 * @returns number of seconds before response message is expected 00686 */ 00687 uint8_t getRxDelay(); 00688 00689 /** Set rx delay in seconds 00690 * Defaults to 1 second 00691 * @param delay number of seconds before response message is expected 00692 * @return MDOT_OK if success 00693 */ 00694 uint32_t setRxDelay(uint8_t delay); 00695 00696 /** Get preserve session to save network session info through reset or power down in AUTO_OTA mode 00697 * Defaults to off 00698 * @returns true if enabled 00699 */ 00700 bool getPreserveSession(); 00701 00702 /** Set preserve session to save network session info through reset or power down in AUTO_OTA mode 00703 * Defaults to off 00704 * @param enable 00705 * @return MDOT_OK if success 00706 */ 00707 uint32_t setPreserveSession(bool enable); 00708 00709 /** Get data pending 00710 * only valid after sending data to the gateway 00711 * @returns true if server has available packet(s) 00712 */ 00713 bool getDataPending(); 00714 00715 /** Get transmitting 00716 * @returns true if currently transmitting 00717 */ 00718 bool getIsTransmitting(); 00719 00720 /** Set TX data rate 00721 * data rates affect maximum payload size 00722 * @param dr SF_7 - SF_12|DR0-DR7 for Europe, SF_7 - SF_10 | DR0-DR4 for United States 00723 * @returns MDOT_OK if success 00724 */ 00725 int32_t setTxDataRate(const uint8_t& dr); 00726 00727 /** Get TX data rate 00728 * @returns current TX data rate (DR0-DR15) 00729 */ 00730 uint8_t getTxDataRate(); 00731 00732 /** Get a random value from the radio based on RSSI 00733 * @returns randome value 00734 */ 00735 uint32_t getRadioRandom(); 00736 00737 00738 /** Get data rate spreading factor and bandwidth 00739 * EU868 Datarates 00740 * --------------- 00741 * DR0 - SF12BW125 00742 * DR1 - SF11BW125 00743 * DR2 - SF10BW125 00744 * DR3 - SF9BW125 00745 * DR4 - SF8BW125 00746 * DR5 - SF7BW125 00747 * DR6 - SF7BW250 00748 * DR7 - FSK 00749 * 00750 * US915 Datarates 00751 * --------------- 00752 * DR0 - SF10BW125 00753 * DR1 - SF9BW125 00754 * DR2 - SF8BW125 00755 * DR3 - SF7BW125 00756 * DR4 - SF8BW500 00757 * 00758 * @returns spreading factor and bandwidth 00759 */ 00760 std::string getDateRateDetails(uint8_t rate); 00761 00762 /** Set TX power output of radio before antenna gain, default: 14 dBm 00763 * actual output power may be limited by local regulations for the chosen frequency 00764 * power affects maximum range 00765 * @param power 2 dBm - 20 dBm 00766 * @returns MDOT_OK if success 00767 */ 00768 int32_t setTxPower(const uint32_t& power); 00769 00770 /** Get TX power 00771 * @returns TX power (2 dBm - 20 dBm) 00772 */ 00773 uint32_t getTxPower(); 00774 00775 /** Get configured gain of installed antenna, default: +3 dBi 00776 * @returns gain of antenna in dBi 00777 */ 00778 int8_t getAntennaGain(); 00779 00780 /** Set configured gain of installed antenna, default: +3 dBi 00781 * @param gain -127 dBi - 128 dBi 00782 * @returns MDOT_OK if success 00783 */ 00784 int32_t setAntennaGain(int8_t gain); 00785 00786 /** Enable/disable TX waiting for rx windows 00787 * when enabled, send calls will block until a packet is received or RX timeout 00788 * @param enable set to true if expecting responses to transmitted packets 00789 * @returns MDOT_OK if success 00790 */ 00791 int32_t setTxWait(const bool& enable); 00792 00793 /** Get TX wait 00794 * @returns true if TX wait is enabled 00795 */ 00796 bool getTxWait(); 00797 00798 /** Get time on air 00799 * @returns the amount of time (in ms) it would take to send bytes bytes based on current configuration 00800 */ 00801 uint32_t getTimeOnAir(uint8_t bytes); 00802 00803 /** Get min frequency 00804 * @returns minimum frequency based on current configuration 00805 */ 00806 uint32_t getMinFrequency(); 00807 00808 /** Get max frequency 00809 * @returns maximum frequency based on current configuration 00810 */ 00811 uint32_t getMaxFrequency(); 00812 00813 // get/set adaptive data rate 00814 // configure data rates and power levels based on signal to noise of packets received at gateway 00815 // true == adaptive data rate is on 00816 // set function returns MDOT_OK if success 00817 int32_t setAdr(const bool& on); 00818 bool getAdr(); 00819 00820 /** Set forward error correction bytes 00821 * @param bytes 1 - 4 bytes 00822 * @returns MDOT_OK if success 00823 */ 00824 int32_t setFec(const uint8_t& bytes); 00825 00826 /** Get forward error correction bytes 00827 * @returns bytes (1 - 4) 00828 */ 00829 uint8_t getFec(); 00830 00831 /** Enable/disable CRC checking of packets 00832 * CRC checking must be enabled for use with Conduit gateway and MTAC_LORA card 00833 * @param on set to true to enable CRC checking 00834 * @returns MDOT_OK if success 00835 */ 00836 int32_t setCrc(const bool& on); 00837 00838 /** Get CRC checking 00839 * @returns true if CRC checking is enabled 00840 */ 00841 bool getCrc(); 00842 00843 /** Set ack 00844 * @param retries 0 to disable acks, otherwise 1 - 8 00845 * @returns MDOT_OK if success 00846 */ 00847 int32_t setAck(const uint8_t& retries); 00848 00849 /** Get ack 00850 * @returns 0 if acks are disabled, otherwise retries (1 - 8) 00851 */ 00852 uint8_t getAck(); 00853 00854 /** Set number of packet repeats for unconfirmed frames 00855 * @param repeat 0 or 1 for no repeats, otherwise 2-15 00856 * @returns MDOT_OK if success 00857 */ 00858 int32_t setRepeat(const uint8_t& repeat); 00859 00860 /** Get number of packet repeats for unconfirmed frames 00861 * @returns 0 or 1 if no repeats, otherwise 2-15 00862 */ 00863 uint8_t getRepeat(); 00864 00865 /** Send data to the gateway 00866 * validates data size (based on spreading factor) 00867 * @param data a vector of up to 242 bytes (may be less based on spreading factor) 00868 * @returns MDOT_OK if packet was sent successfully (ACKs disabled), or if an ACK was received (ACKs enabled) 00869 */ 00870 int32_t send(const std::vector<uint8_t>& data, const bool& blocking = true, const bool& highBw = false); 00871 00872 /** Fetch data received from the gateway 00873 * this function only checks to see if a packet has been received - it does not open a receive window 00874 * send() must be called before recv() 00875 * @param data a vector to put the received data into 00876 * @returns MDOT_OK if packet was successfully received 00877 */ 00878 int32_t recv(std::vector<uint8_t>& data); 00879 00880 /** Ping 00881 * status will be MDOT_OK if ping succeeded 00882 * @returns ping_response struct containing status, RSSI, and SNR 00883 */ 00884 ping_response ping(); 00885 00886 /** Get return code string 00887 * @returns string containing a description of the given error code 00888 */ 00889 static std::string getReturnCodeString(const int32_t& code); 00890 00891 /** Get last error 00892 * @returns string explaining the last error that occured 00893 */ 00894 std::string getLastError(); 00895 00896 /** Go to sleep 00897 * @param interval the number of seconds to sleep before waking up if wakeup_mode == RTC_ALARM or RTC_ALARM_OR_INTERRUPT, else ignored 00898 * @param wakeup_mode RTC_ALARM, INTERRUPT, RTC_ALARM_OR_INTERRUPT 00899 * if RTC_ALARM the real time clock is configured to wake the device up after the specified interval 00900 * if INTERRUPT the device will wake up on the rising edge of the interrupt pin 00901 * if RTC_ALARM_OR_INTERRUPT the device will wake on the first event to occur 00902 * @param deepsleep if true go into deep sleep mode (lowest power, all memory and registers are lost, peripherals turned off) 00903 * else go into sleep mode (low power, memory and registers are maintained, peripherals stay on) 00904 * 00905 * in sleep mode, the device can be woken up on an XBEE_DI (2-8) pin or by the RTC alarm 00906 * in deepsleep mode, the device can only be woken up using the WKUP pin (PA0, XBEE_DIO7) or by the RTC alarm 00907 */ 00908 void sleep(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM, const bool& deepsleep = true); 00909 00910 /** Set wake pin 00911 * @param pin the pin to use to wake the device from sleep mode XBEE_DI (2-8) 00912 */ 00913 void setWakePin(const PinName& pin); 00914 00915 /** Get wake pin 00916 * @returns the pin to use to wake the device from sleep mode XBEE_DI (2-8) 00917 */ 00918 PinName getWakePin(); 00919 00920 /** Write data in a user backup register 00921 * @param register one of UBR0 through UBR9 00922 * @param data user data to back up 00923 * @returns true if success 00924 */ 00925 bool writeUserBackupRegister(uint32_t reg, uint32_t data); 00926 00927 /** Read data in a user backup register 00928 * @param register one of UBR0 through UBR9 00929 * @param data gets set to content of register 00930 * @returns true if success 00931 */ 00932 bool readUserBackupRegister(uint32_t reg, uint32_t& data); 00933 00934 // Save user file data to flash 00935 // file - name of file max 30 chars 00936 // data - data of file 00937 // size - size of file 00938 bool saveUserFile(const char* file, void* data, uint32_t size); 00939 00940 // Append user file data to flash 00941 // file - name of file max 30 chars 00942 // data - data of file 00943 // size - size of file 00944 bool appendUserFile(const char* file, void* data, uint32_t size); 00945 00946 // Read user file data from flash 00947 // file - name of file max 30 chars 00948 // data - data of file 00949 // size - size of file 00950 bool readUserFile(const char* file, void* data, uint32_t size); 00951 00952 // Move a user file in flash 00953 // file - name of file 00954 // new_name - new name of file 00955 bool moveUserFile(const char* file, const char* new_name); 00956 00957 // Delete user file data from flash 00958 // file - name of file max 30 chars 00959 bool deleteUserFile(const char* file); 00960 00961 // Open user file in flash, max of 4 files open concurrently 00962 // file - name of file max 30 chars 00963 // mode - combination of FM_APPEND | FM_TRUNC | FM_CREAT | 00964 // FM_RDONLY | FM_WRONLY | FM_RDWR | FM_DIRECT 00965 // returns - mdot_file struct, fd field will be a negative value if file could not be opened 00966 mDot::mdot_file openUserFile(const char* file, int mode); 00967 00968 // Seek an open file 00969 // file - mdot file struct 00970 // offset - offset in bytes 00971 // whence - where offset is based SEEK_SET, SEEK_CUR, SEEK_END 00972 bool seekUserFile(mDot::mdot_file& file, size_t offset, int whence); 00973 00974 // Read bytes from open file 00975 // file - mdot file struct 00976 // data - mem location to store data 00977 // length - number of bytes to read 00978 // returns - number of bytes written 00979 int readUserFile(mDot::mdot_file& file, void* data, size_t length); 00980 00981 // Write bytes to open file 00982 // file - mdot file struct 00983 // data - data to write 00984 // length - number of bytes to write 00985 // returns - number of bytes written 00986 int writeUserFile(mDot::mdot_file& file, void* data, size_t length); 00987 00988 // Close open file 00989 // file - mdot file struct 00990 bool closeUserFile(mDot::mdot_file& file); 00991 00992 // List user files stored in flash 00993 std::vector<mDot::mdot_file> listUserFiles(); 00994 00995 // Move file into the firmware upgrade path to be flashed on next boot 00996 // file - name of file 00997 bool moveUserFileToFirmwareUpgrade(const char* file); 00998 00999 // get current statistics 01000 // Join Attempts, Join Fails, Up Packets, Down Packets, Missed Acks 01001 mdot_stats getStats(); 01002 01003 // reset statistics 01004 // Join Attempts, Join Fails, Up Packets, Down Packets, Missed Acks 01005 void resetStats(); 01006 01007 // Convert pin number 2-8 to pin name DIO2-DI8 01008 static PinName pinNum2Name(uint8_t num); 01009 01010 // Convert pin name DIO2-DI8 to pin number 2-8 01011 static uint8_t pinName2Num(PinName name); 01012 01013 // Convert pin name DIO2-DI8 to string 01014 static std::string pinName2Str(PinName name); 01015 01016 uint64_t crc64(uint64_t crc, const unsigned char *s, uint64_t l); 01017 01018 /************************************************************************* 01019 * The following functions are only used by the AT command application and 01020 * should not be used by standard applications consuming the mDot library 01021 ************************************************************************/ 01022 01023 // set/get configured baud rate for command port 01024 // only for use in conjunction with AT interface 01025 // set function returns MDOT_OK if success 01026 int32_t setBaud(const uint32_t& baud); 01027 uint32_t getBaud(); 01028 01029 // set/get baud rate for debug port 01030 // set function returns MDOT_OK if success 01031 int32_t setDebugBaud(const uint32_t& baud); 01032 uint32_t getDebugBaud(); 01033 01034 // set/get command terminal echo 01035 // set function returns MDOT_OK if success 01036 int32_t setEcho(const bool& on); 01037 bool getEcho(); 01038 01039 // set/get command terminal verbose mode 01040 // set function returns MDOT_OK if success 01041 int32_t setVerbose(const bool& on); 01042 bool getVerbose(); 01043 01044 // set/get startup mode 01045 // COMMAND_MODE (default), starts up ready to accept AT commands 01046 // SERIAL_MODE, read serial data and send it as LoRa packets 01047 // set function returns MDOT_OK if success 01048 int32_t setStartUpMode(const uint8_t& mode); 01049 uint8_t getStartUpMode(); 01050 01051 int32_t setRxDataRate(const uint8_t& dr); 01052 uint8_t getRxDataRate(); 01053 01054 // get/set TX/RX frequency 01055 // if frequency band == FB_868 (Europe), must be between 863000000 - 870000000 01056 // if frequency band == FB_915 (United States), must be between 902000000-928000000 01057 // if set to 0, device will hop frequencies 01058 // set function returns MDOT_OK if success 01059 int32_t setTxFrequency(const uint32_t& freq); 01060 uint32_t getTxFrequency(); 01061 int32_t setRxFrequency(const uint32_t& freq); 01062 uint32_t getRxFrequency(); 01063 01064 // get/set TX/RX inverted 01065 // true == signal is inverted 01066 // set function returns MDOT_OK if success 01067 int32_t setTxInverted(const bool& on); 01068 bool getTxInverted(); 01069 int32_t setRxInverted(const bool& on); 01070 bool getRxInverted(); 01071 01072 // get/set RX output mode 01073 // valid options are HEXADECIMAL and BINARY 01074 // set function returns MDOT_OK if success 01075 int32_t setRxOutput(const uint8_t& mode); 01076 uint8_t getRxOutput(); 01077 01078 // get/set serial wake interval 01079 // valid values are 2 s - INT_MAX (2147483647) s 01080 // set function returns MDOT_OK if success 01081 int32_t setWakeInterval(const uint32_t& interval); 01082 uint32_t getWakeInterval(); 01083 01084 // get/set serial wake delay 01085 // valid values are 2 ms - INT_MAX (2147483647) ms 01086 // set function returns MDOT_OK if success 01087 int32_t setWakeDelay(const uint32_t& delay); 01088 uint32_t getWakeDelay(); 01089 01090 // get/set serial receive timeout 01091 // valid values are 0 ms - 65000 ms 01092 // set function returns MDOT_OK if success 01093 int32_t setWakeTimeout(const uint16_t& timeout); 01094 uint16_t getWakeTimeout(); 01095 01096 // get/set serial wake mode 01097 // valid values are INTERRUPT or RTC_ALARM 01098 // set function returns MDOT_OK if success 01099 int32_t setWakeMode(const uint8_t& delay); 01100 uint8_t getWakeMode(); 01101 01102 // get/set serial flow control enabled 01103 // set function returns MDOT_OK if success 01104 int32_t setFlowControl(const bool& on); 01105 bool getFlowControl(); 01106 01107 01108 // get/set serial clear on error 01109 // if enabled the data read from the serial port will be discarded if it cannot be sent or if the send fails 01110 // set function returns MDOT_OK if success 01111 int32_t setSerialClearOnError(const bool& on); 01112 bool getSerialClearOnError(); 01113 01114 // MTS_RADIO_DEBUG_COMMANDS 01115 01116 void openRxWindow(uint32_t timeout, uint8_t bandwidth = 0); 01117 void sendContinuous(); 01118 int32_t setDeviceId(const std::vector<uint8_t>& id); 01119 int32_t setFrequencyBand(const uint8_t& band); 01120 bool saveProtectedConfig(); 01121 void resetRadio(); 01122 int32_t setRadioMode(const uint8_t& mode); 01123 std::map<uint8_t, uint8_t> dumpRegisters(); 01124 void eraseFlash(); 01125 01126 // deprecated - use setWakeInterval 01127 int32_t setSerialWakeInterval(const uint32_t& interval); 01128 // deprecated - use getWakeInterval 01129 uint32_t getSerialWakeInterval(); 01130 01131 // deprecated - use setWakeDelay 01132 int32_t setSerialWakeDelay(const uint32_t& delay); 01133 // deprecated - use setWakeDelay 01134 uint32_t getSerialWakeDelay(); 01135 01136 // deprecated - use setWakeTimeout 01137 int32_t setSerialReceiveTimeout(const uint16_t& timeout); 01138 // deprecated - use getWakeTimeout 01139 uint16_t getSerialReceiveTimeout(); 01140 01141 void setWakeupCallback(void (*function)(void)); 01142 01143 template<typename T> 01144 void setWakeupCallback(T *object, void (T::*member)(void)) { 01145 _wakeup_callback.attach(object, member); 01146 } 01147 01148 private: 01149 mdot_stats _stats; 01150 01151 FunctionPointer _wakeup_callback; 01152 01153 bool _standbyFlag; 01154 bool _testMode; 01155 uint8_t _savedPort; 01156 void handleTestModePacket(); 01157 01158 }; 01159 01160 #endif
Generated on Wed Jul 13 2022 11:18:29 by
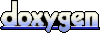