Library for LoRa communication using MultiTech MDOT.
Dependents: mDot_test_rx adc_sensor_lora mDotEVBM2X mDot_AT_firmware ... more
MTSText.h
00001 #ifndef MTSTEXT_H 00002 #define MTSTEXT_H 00003 00004 #include <string> 00005 #include <vector> 00006 #include <stddef.h> 00007 #include <stdint.h> 00008 #include <stdio.h> 00009 #include <string.h> 00010 00011 namespace mts 00012 { 00013 00014 /** This class contains a number of static methods for manipulating strings and other 00015 * text data. 00016 */ 00017 class Text 00018 { 00019 public: 00020 /** This static method can be used to pull out a string at the next line break. A 00021 * break can either be a newline '\n', carriage return '\r' or both. 00022 * 00023 * @param source the source string to look for the line break on. 00024 * @param start the start postion within the string to begin looking for the line 00025 * break. 00026 * @param cursor this value will be updated with the index for the next available character 00027 * after the line break. If a line break is not found returns -1. 00028 * @returns the string beginning with the start index up to including the line breaks. 00029 */ 00030 static std::string getLine(const std::string& source, const size_t& start, size_t& cursor); 00031 00032 /** This is a static method for splitting strings using a delimeter value. 00033 * 00034 * @param str the string to try and split. 00035 * @param delimiter the delimeter value to split on as a character. 00036 * @param limit the maximum number of splits. If equal to 0 it splits as amny times as possible. 00037 * The default is 0. 00038 * @returns an ordered vector of strings conatining the splits of the original string. 00039 */ 00040 static std::vector<std::string> split(const std::string& str, char delimiter, int limit = 0); 00041 00042 /** This is a static method for splitting strings using a delimeter value. 00043 * 00044 * @param str the string to try and split. 00045 * @param delimiter the delimeter value to split on as a string. 00046 * @param limit the maximum number of splits. If equal to 0 it splits as amny times as possible. 00047 * The default is 0. 00048 * @returns an ordered vector of strings conatining the splits of the original string. 00049 */ 00050 static std::vector<std::string> split(const std::string& str, const std::string& delimiter, int limit = 0); 00051 00052 static std::string readString(char* index, int length); 00053 00054 static std::string toUpper(const std::string str); 00055 00056 static std::string float2String(double val, int precision); 00057 00058 static std::string bin2hexString(const std::vector<uint8_t>& data, const char* delim = "", bool leadingZeros = false, bool bytePadding = true); 00059 00060 static std::string bin2hexString(const uint8_t* data, const uint32_t len, const char* delim = "", bool leadingZeros = false, bool bytePadding = true); 00061 00062 static std::string bin2base64(const std::vector<uint8_t>& data); 00063 00064 static std::string bin2base64(const uint8_t* data, size_t size); 00065 00066 static bool base642bin(const std::string in, std::vector<uint8_t>& out); 00067 00068 static void ltrim(std::string& str, const char* args); 00069 00070 static void rtrim(std::string& str, const char* args); 00071 00072 static void trim(std::string& str, const char* args); 00073 00074 private: 00075 // Safety for class with only static methods 00076 Text(); 00077 Text(const Text& other); 00078 Text& operator=(const Text& other); 00079 }; 00080 00081 } 00082 00083 #endif
Generated on Wed Jul 13 2022 11:18:29 by
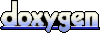