Library for LoRa communication using MultiTech MDOT.
Dependents: mDot_test_rx adc_sensor_lora mDotEVBM2X mDot_AT_firmware ... more
LoRaMacEvent.h
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C)2013 Semtech 00008 00009 Description: Generic radio driver definition 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainer: Miguel Luis and Gregory Cristian 00014 */ 00015 #ifndef __LORAMACEVENT_H__ 00016 #define __LORAMACEVENT_H__ 00017 00018 /*! 00019 * LoRaMAC event flags 00020 */ 00021 typedef union { 00022 uint8_t Value; 00023 struct { 00024 uint8_t :1; 00025 uint8_t Tx :1; 00026 uint8_t Rx :1; 00027 uint8_t RxData :1; 00028 uint8_t RxSlot :2; 00029 uint8_t LinkCheck :1; 00030 uint8_t JoinAccept :1; 00031 } Bits; 00032 } LoRaMacEventFlags ; 00033 00034 typedef enum { 00035 LORAMAC_EVENT_INFO_STATUS_OK = 0, 00036 LORAMAC_EVENT_INFO_STATUS_ERROR, 00037 LORAMAC_EVENT_INFO_STATUS_TX_TIMEOUT, 00038 LORAMAC_EVENT_INFO_STATUS_RX_TIMEOUT, 00039 LORAMAC_EVENT_INFO_STATUS_RX_ERROR, 00040 LORAMAC_EVENT_INFO_STATUS_JOIN_FAIL, 00041 LORAMAC_EVENT_INFO_STATUS_DOWNLINK_FAIL, 00042 LORAMAC_EVENT_INFO_STATUS_ADDRESS_FAIL, 00043 LORAMAC_EVENT_INFO_STATUS_MIC_FAIL, 00044 } LoRaMacEventInfoStatus; 00045 00046 /*! 00047 * LoRaMAC event information 00048 */ 00049 typedef struct { 00050 LoRaMacEventInfoStatus Status; 00051 bool TxAckReceived; 00052 uint8_t TxNbRetries; 00053 uint8_t TxDatarate; 00054 uint8_t RxPort; 00055 uint8_t *RxBuffer; 00056 uint8_t RxBufferSize; 00057 int16_t RxRssi; 00058 uint8_t RxSnr; 00059 uint16_t Energy; 00060 uint8_t DemodMargin; 00061 uint8_t NbGateways; 00062 } LoRaMacEventInfo ; 00063 00064 /*! 00065 * LoRaMAC events structure 00066 * Used to notify upper layers of MAC events 00067 */ 00068 class LoRaMacEvent { 00069 public: 00070 00071 virtual ~LoRaMacEvent () {} 00072 00073 /*! 00074 * MAC layer event callback prototype. 00075 * 00076 * \param [IN] flags Bit field indicating the MAC events occurred 00077 * \param [IN] info Details about MAC events occurred 00078 */ 00079 virtual void MacEvent (LoRaMacEventFlags *flags, LoRaMacEventInfo *info) { 00080 00081 if (flags->Bits.Rx) { 00082 delete[] info->RxBuffer; 00083 } 00084 } 00085 00086 virtual uint8_t MeasureBattery(void) { 00087 return 255; 00088 } 00089 }; 00090 00091 #endif // __LORAMACEVENT_H__
Generated on Wed Jul 13 2022 11:18:29 by
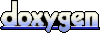