
Custom Channel Plan version of MTDOT Box firmware
Dependencies: DOGS102 GpsParser ISL29011 MMA845x MPL3115A2 MTS-Serial NCP5623B libmDot-Custom mDot_Channel_Plans
Fork of MTDOT-BOX-EVB-Factory-Firmware by
main.cpp
00001 /* Copyright (c) <2016> <MultiTech Systems>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 // mbed headers 00020 #include "mbed.h" 00021 #include "rtos.h" 00022 // MTS headers 00023 #include "mDot.h" 00024 #include "MTSLog.h" 00025 // display headers 00026 #include "DOGS102.h" 00027 #include "NCP5623B.h" 00028 #include "LayoutStartup.h" 00029 #include "LayoutScrollSelect.h" 00030 #include "LayoutConfig.h" 00031 #include "LayoutHelp.h" 00032 // button header 00033 #include "ButtonHandler.h" 00034 // LoRa header 00035 #include "LoRaHandler.h" 00036 // Sensor header 00037 #include "SensorHandler.h" 00038 // mode objects 00039 #include "ModeJoin.h" 00040 #include "ModeSingle.h" 00041 #include "ModeSweep.h" 00042 #include "ModeDemo.h" 00043 #include "ModeConfig.h" 00044 #include "ModeGps.h" 00045 #include "ModeData.h" 00046 // misc heders 00047 #include "FileName.h" 00048 #include <string> 00049 00050 #include "ChannelPlan.h" 00051 #include "ChannelPlans.h" 00052 00053 #define DISABLE_DUTY_CYCLE true 00054 00055 00056 // LCD and LED controllers 00057 SPI lcd_spi(SPI1_MOSI, SPI1_MISO, SPI1_SCK); 00058 I2C led_i2c(I2C_SDA, I2C_SCL); 00059 DigitalOut lcd_spi_cs(SPI1_CS, 1); 00060 DigitalOut lcd_cd(XBEE_ON_SLEEP, 1); 00061 DOGS102* lcd; 00062 NCP5623B* led_cont; 00063 00064 // Thread informaiton 00065 osThreadId main_id; 00066 00067 // Button controller 00068 ButtonHandler* buttons; 00069 00070 // LoRa controller 00071 LoRaHandler* lora_handler; 00072 00073 mDot* dot; 00074 00075 // GPS 00076 GPSPARSER* gps; 00077 MTSSerial gps_serial(XBEE_DOUT, XBEE_DIN, 256, 2048); 00078 00079 // Sensors 00080 SensorHandler* sensors; 00081 00082 // Modes 00083 ModeJoin* modeJoin; 00084 ModeSingle* modeSingle; 00085 ModeSweep* modeSweep; 00086 ModeDemo* modeDemo; 00087 ModeConfig* modeConfig; 00088 ModeGps* modeGps; 00089 ModeData* modeData; 00090 00091 // Serial debug port 00092 Serial debug(USBTX, USBRX); 00093 00094 // Survey Data File 00095 char file_name[] = "SurveyData.txt"; 00096 00097 // Prototypes 00098 void mainMenu(); 00099 00100 int main() { 00101 debug.baud(115200); 00102 00103 lcd = new DOGS102(lcd_spi, lcd_spi_cs, lcd_cd); 00104 // NCP5623B::LEDs 1 & 2 are the screen backlight - not used on default build 00105 // NCP5623B::LED3 is EVB LED2 00106 led_cont = new NCP5623B(led_i2c); 00107 00108 main_id = Thread::gettid(); 00109 buttons = new ButtonHandler(main_id); 00110 dot = mDot::getInstance(); 00111 lora_handler = new LoRaHandler(main_id); 00112 00113 lora::ChannelPlan *plan = new lora::CustomChannelPlan_AS923(*dot->getRadio(), *dot->getSettings());; 00114 dot->setChannelPlan(plan); 00115 00116 dot->setDisableDutyCycle(DISABLE_DUTY_CYCLE); 00117 dot->setLinkCheckThreshold(0); 00118 dot->setLinkCheckCount(0); 00119 00120 // Seed the RNG 00121 srand(dot->getRadioRandom()); 00122 00123 gps = new GPSPARSER(&gps_serial, led_cont); 00124 sensors = new SensorHandler(); 00125 00126 led_cont->setLEDCurrent(16); 00127 00128 MTSLog::setLogLevel(MTSLog::TRACE_LEVEL); 00129 00130 modeJoin = new ModeJoin(lcd, buttons, dot, lora_handler, gps, sensors); 00131 modeSingle = new ModeSingle(lcd, buttons, dot, lora_handler, gps, sensors); 00132 modeSweep = new ModeSweep(lcd, buttons, dot, lora_handler, gps, sensors); 00133 modeDemo = new ModeDemo(lcd, buttons, dot, lora_handler, gps, sensors); 00134 modeConfig = new ModeConfig(lcd, buttons, dot, lora_handler, gps, sensors); 00135 modeGps = new ModeGps(lcd, buttons, dot, lora_handler, gps, sensors, modeJoin); 00136 modeData = new ModeData(lcd, buttons, dot, lora_handler, gps, sensors); 00137 00138 00139 osDelay(1000); 00140 logInfo("%sGPS detected", gps->gpsDetected() ? "" : "no "); 00141 00142 // display startup screen for 3 seconds 00143 LayoutStartup ls(lcd, dot); 00144 ls.display(); 00145 ls.updateGPS(gps->gpsDetected()); 00146 osDelay(3000); 00147 00148 logInfo("displaying main menu"); 00149 mainMenu(); 00150 00151 return 0; 00152 } 00153 00154 void mainMenu() { 00155 bool mode_selected = false; 00156 std::string selected; 00157 std::string product; 00158 00159 typedef enum { 00160 demo = 1, 00161 config, 00162 single, 00163 sweep, 00164 gps, 00165 data 00166 00167 } menu_items; 00168 00169 std::string menu_strings[] = { 00170 "Select Mode", 00171 "LoRa Demo", 00172 "Configuration", 00173 "Survey Single", 00174 "Survey Sweep", 00175 "Survey GPS", 00176 "View Data" 00177 }; 00178 std::vector<std::string> items; 00179 items.push_back(menu_strings[demo]); 00180 items.push_back(menu_strings[config]); 00181 items.push_back(menu_strings[single]); 00182 items.push_back(menu_strings[sweep]); 00183 items.push_back(menu_strings[gps]); 00184 items.push_back(menu_strings[data]); 00185 00186 while (true) { 00187 product = "DOT-BOX/EVB "; 00188 product += dot->FrequencyBandStr(dot->getFrequencyBand()); 00189 00190 // reset session between modes 00191 dot->resetNetworkSession(); 00192 lora_handler->resetActivityLed(); 00193 00194 LayoutScrollSelect menu(lcd, items, product, menu_strings[0]); 00195 menu.display(); 00196 00197 while (! mode_selected) { 00198 osEvent e = Thread::signal_wait(buttonSignal); 00199 if (e.status == osEventSignal) { 00200 ButtonHandler::ButtonEvent ev = buttons->getButtonEvent(); 00201 switch (ev) { 00202 case ButtonHandler::sw1_press: 00203 selected = menu.select(); 00204 mode_selected = true; 00205 break; 00206 case ButtonHandler::sw2_press: 00207 menu.scroll(); 00208 break; 00209 case ButtonHandler::sw1_hold: 00210 break; 00211 default: 00212 break; 00213 } 00214 } 00215 } 00216 if (selected == menu_strings[demo]) { 00217 if (modeJoin->start()) 00218 modeDemo->start(); 00219 } else if (selected == menu_strings[config]) { 00220 modeConfig->start(); 00221 } else if (selected == menu_strings[single]) { 00222 if (modeJoin->start()) 00223 modeSingle->start(); 00224 } else if (selected == menu_strings[sweep]) { 00225 if (modeJoin->start()) 00226 modeSweep->start(); 00227 } else if (selected == menu_strings[gps]) { 00228 modeJoin->start(); 00229 modeGps->start(); 00230 } else if (selected == menu_strings[data]) { 00231 modeData->start(); 00232 } 00233 00234 mode_selected = false; 00235 } 00236 } 00237 00238 00239
Generated on Tue Jul 12 2022 13:07:49 by
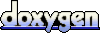