
Custom Channel Plan version of MTDOT Box firmware
Dependencies: DOGS102 GpsParser ISL29011 MMA845x MPL3115A2 MTS-Serial NCP5623B libmDot-Custom mDot_Channel_Plans
Fork of MTDOT-BOX-EVB-Factory-Firmware by
SensorHandler.cpp
00001 /* Copyright (c) <2016> <MultiTech Systems>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 /** 00020 * @file SensorHandler.cpp 00021 * @brief Reads on board sensors... acceleration, pressure, light and temperture. 00022 * @author Leon Lindenfelser 00023 * @version 1.0 00024 * 00025 */ 00026 00027 #include "SensorHandler.h" 00028 00029 SensorHandler::SensorHandler() 00030 : _getSensorThread(&SensorHandler::startSensorThread, this), 00031 _mDoti2c(PC_9,PA_8), 00032 _accelerometer(_mDoti2c,MMA845x::SA0_VSS), 00033 _barometricSensor(_mDoti2c), 00034 _lightSensor(_mDoti2c) 00035 { 00036 SensorHandler::initSensors(); 00037 _getSensorThread.signal_set(START_THREAD); 00038 return; 00039 } 00040 00041 SensorHandler::~SensorHandler(void) 00042 { 00043 _getSensorThread.terminate(); 00044 } 00045 00046 void SensorHandler::initSensors(){ 00047 // Setup the Accelerometer for 8g range, 14 bit resolution, Noise reduction off, sample rate 1.56 Hz 00048 // normal oversample mode, High pass filter off 00049 _accelerometer.setCommonParameters(MMA845x::RANGE_8g,MMA845x::RES_MAX,MMA845x::LN_OFF, 00050 MMA845x::DR_6_25,MMA845x::OS_NORMAL,MMA845x::HPF_OFF ); 00051 00052 // Setup the Barometric sensor for post processed Ambient pressure, 4 samples per data acquisition. 00053 //and a sample taken every second when in active mode 00054 _barometricSensor.setParameters(MPL3115A2::DATA_NORMAL, MPL3115A2::DM_BAROMETER, MPL3115A2::OR_16, 00055 MPL3115A2::AT_1); 00056 // Setup the Ambient Light Sensor for continuous Ambient Light Sensing, 16 bit resolution, 00057 // and 16000 lux range 00058 _lightSensor.setMode(ISL29011::ALS_CONT); 00059 _lightSensor.setResolution(ISL29011::ADC_16BIT); 00060 _lightSensor.setRange(ISL29011::RNG_16000); 00061 00062 // Set the accelerometer for active mode 00063 _accelerometer.activeMode(); 00064 00065 // Clear the min-max registers in the Barometric Sensor 00066 _barometricSensor.clearMinMaxRegs(); 00067 } 00068 00069 void SensorHandler::startSensorThread(void const *p) 00070 { 00071 SensorHandler *instance = (SensorHandler*)p; 00072 instance->readSensors(); 00073 } 00074 00075 void SensorHandler::readSensors() 00076 { 00077 uint8_t result; 00078 Timer timer; 00079 timer.start(); 00080 00081 _getSensorThread.signal_wait(START_THREAD); 00082 while(1){ 00083 // Test Accelerometer XYZ data ready bit to see if acquisition complete 00084 timer.reset(); 00085 do { 00086 osDelay(20); // allows other threads to process 00087 result = _accelerometer.getStatus(); 00088 if((result & MMA845x::XYZDR) != 0 ){ 00089 // Retrieve accelerometer data 00090 _mutex.lock(); 00091 _accelerometerData = _accelerometer.getXYZ(); 00092 _mutex.unlock(); 00093 } 00094 } while (((result & MMA845x::XYZDR) == 0 ) && (timer.read_ms() < 1000)); 00095 00096 00097 // Trigger a Pressure reading 00098 _barometricSensor.setParameters(MPL3115A2::DATA_NORMAL, MPL3115A2::DM_BAROMETER, MPL3115A2::OR_16, 00099 MPL3115A2::AT_1); 00100 _barometricSensor.triggerOneShot(); 00101 00102 // Test barometer device status to see if acquisition is complete 00103 timer.reset(); 00104 do { 00105 osDelay(20); // allows other threads to process 00106 result = _barometricSensor.getStatus(); 00107 if((result & MPL3115A2::PTDR) != 0){ 00108 // Retrieve barometric pressure 00109 _mutex.lock(); 00110 _pressure = _barometricSensor.getBaroData() >> 12; // convert 32 bit signed to 20 bit unsigned value 00111 _mutex.unlock(); 00112 } 00113 } while (((result & MPL3115A2::PTDR) == 0) && (timer.read_ms() < 100)); 00114 00115 00116 // Trigger an Altitude reading 00117 _barometricSensor.setParameters(MPL3115A2::DATA_NORMAL, MPL3115A2::DM_ALTIMETER, MPL3115A2::OR_16, 00118 MPL3115A2::AT_1); 00119 _barometricSensor.triggerOneShot(); 00120 00121 // Test barometer device status to see if acquisition is complete 00122 timer.reset(); 00123 do { 00124 osDelay(20); // allows other threads to process 00125 result = _barometricSensor.getStatus(); 00126 if((result & MPL3115A2::PTDR) != 0 ){ 00127 // Retrieve temperature and altitude. 00128 _mutex.lock(); 00129 _barometerData = _barometricSensor.getAllData(false); 00130 _mutex.unlock(); 00131 } 00132 } while (((result & MPL3115A2::PTDR) == 0 ) && (timer.read_ms() < 100)); 00133 00134 // Retrieve light level 00135 _mutex.lock(); 00136 _light = _lightSensor.getData(); 00137 _mutex.unlock(); 00138 osDelay(100); // allows other threads to process 00139 } 00140 } 00141 00142 MMA845x_DATA SensorHandler::getAcceleration(){ 00143 MMA845x_DATA data; 00144 _mutex.lock(); 00145 data = _accelerometerData; 00146 _mutex.unlock(); 00147 return data; 00148 } 00149 00150 float SensorHandler::getLight(){ 00151 float light; 00152 uint16_t whole; 00153 _mutex.lock(); 00154 whole = _light; 00155 _mutex.unlock(); 00156 light = whole * 24 % 100; 00157 light /= 100; 00158 light = light + (whole * 24 / 100); // 16000 lux full scale .24 lux per bit 00159 return light; 00160 } 00161 00162 uint16_t SensorHandler::getLightRaw(){ 00163 uint16_t light; 00164 _mutex.lock(); 00165 light = _light; 00166 _mutex.unlock(); 00167 return light; 00168 } 00169 00170 float SensorHandler::getPressure(){ 00171 float pressure; 00172 uint32_t whole; 00173 _mutex.lock(); 00174 whole = _pressure; 00175 _mutex.unlock(); 00176 pressure = (whole & 3) * .25; 00177 pressure = pressure + (whole >> 2); 00178 return pressure; 00179 } 00180 00181 uint32_t SensorHandler::getPressureRaw(){ 00182 uint32_t pressure; 00183 _mutex.lock(); 00184 pressure = _pressure; 00185 _mutex.unlock(); 00186 return pressure; 00187 } 00188 00189 float SensorHandler::getTemp(Scale scale){ 00190 float temperature; 00191 uint16_t whole; 00192 _mutex.lock(); 00193 whole = _barometerData._temp; 00194 _mutex.unlock(); 00195 temperature = whole & 0x0f; 00196 temperature *= .0625; 00197 temperature += (whole >> 4); 00198 if(scale == FAHRENHEIT){ 00199 temperature = temperature * 1.8 + 32; 00200 } 00201 return temperature; 00202 } 00203 00204 float SensorHandler::getAltitude(){ 00205 float altitude; 00206 uint32_t whole; 00207 _mutex.lock(); 00208 whole = _barometerData._baro; 00209 _mutex.unlock(); 00210 whole /= 4096; 00211 altitude = (whole & 0x0f) * .0625; 00212 whole /= 16; 00213 altitude += whole; 00214 return altitude; 00215 } 00216 00217 MPL3115A2_DATA SensorHandler::getBarometer(){ 00218 MPL3115A2_DATA data; 00219 _mutex.lock(); 00220 data = _barometerData; 00221 _mutex.unlock(); 00222 return data; 00223 } 00224
Generated on Tue Jul 12 2022 13:07:49 by
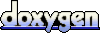