
Custom Channel Plan version of MTDOT Box firmware
Dependencies: DOGS102 GpsParser ISL29011 MMA845x MPL3115A2 MTS-Serial NCP5623B libmDot-Custom mDot_Channel_Plans
Fork of MTDOT-BOX-EVB-Factory-Firmware by
LoRaHandler.cpp
00001 /* Copyright (c) <2016> <MultiTech Systems>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "LoRaHandler.h" 00020 00021 #define signal (int32_t)0xA0 00022 00023 typedef enum { 00024 l_none = 0, 00025 l_link_check, 00026 l_send, 00027 l_join 00028 } InternalLoRa; 00029 00030 uint8_t cmd = l_none; 00031 std::vector<uint8_t> send_data; 00032 00033 void l_worker(void const* argument) { 00034 LoRaHandler* l = (LoRaHandler*)argument; 00035 osEvent e; 00036 00037 l->_dot = mDot::getInstance(); 00038 int32_t ret; 00039 mDot::link_check lc; 00040 mDot::rssi_stats rs; 00041 mDot::snr_stats ss; 00042 00043 while (true) { 00044 e = Thread::signal_wait(signal); 00045 if (e.status == osEventSignal) { 00046 l->_status = LoRaHandler::busy; 00047 l->_tick.attach(l, &LoRaHandler::blinker, 0.05); 00048 switch (cmd) { 00049 case l_link_check: 00050 l->_mutex.lock(); 00051 lc = l->_dot->networkLinkCheck(); 00052 l->_mutex.unlock(); 00053 if (lc.status) { 00054 l->_link.up = lc; 00055 l->_mutex.lock(); 00056 rs = l->_dot->getRssiStats(); 00057 ss = l->_dot->getSnrStats(); 00058 l->_mutex.unlock(); 00059 l->_link.down.rssi = rs.last; 00060 l->_link.down.snr = ss.last; 00061 l->_status = LoRaHandler::link_check_success; 00062 } else { 00063 l->_status = LoRaHandler::link_check_failure; 00064 } 00065 osSignalSet(l->_main, loraSignal); 00066 l->_tick.detach(); 00067 l->_activity_led = LoRaHandler::green; 00068 break; 00069 00070 case l_send: 00071 l->_mutex.lock(); 00072 ret = l->_dot->send(send_data); 00073 l->_mutex.unlock(); 00074 if (ret == mDot::MDOT_OK) 00075 l->_status = LoRaHandler::send_success; 00076 else 00077 l->_status = LoRaHandler::send_failure; 00078 osSignalSet(l->_main, loraSignal); 00079 l->_tick.detach(); 00080 l->_activity_led = LoRaHandler::green; 00081 break; 00082 00083 case l_join: 00084 l->_mutex.lock(); 00085 ret = l->_dot->joinNetworkOnce(); 00086 l->_join_attempts++; 00087 l->_mutex.unlock(); 00088 if (ret == mDot::MDOT_OK) { 00089 l->_status = LoRaHandler::join_success; 00090 osSignalSet(l->_main, loraSignal); 00091 l->_tick.detach(); 00092 l->_activity_led = LoRaHandler::green; 00093 } else { 00094 l->_status = LoRaHandler::join_failure; 00095 osSignalSet(l->_main, loraSignal); 00096 l->_tick.detach(); 00097 l->_activity_led = LoRaHandler::red; 00098 } 00099 00100 break; 00101 00102 default: 00103 l->_status = LoRaHandler::none; 00104 break; 00105 } 00106 } 00107 } 00108 } 00109 00110 LoRaHandler::LoRaHandler(osThreadId main) 00111 : _main(main), 00112 _thread(l_worker, (void*)this), 00113 _status(none), 00114 _join_attempts(1), 00115 _activity_led(XBEE_DIO1, PIN_OUTPUT, PullNone, red) 00116 { 00117 _link.status = false; 00118 _activity_led = red; 00119 } 00120 00121 bool LoRaHandler::linkCheck() { 00122 return action(l_link_check); 00123 } 00124 00125 bool LoRaHandler::send(std::vector<uint8_t> data) { 00126 send_data = data; 00127 return action(l_send); 00128 } 00129 00130 bool LoRaHandler::join() { 00131 return action(l_join); 00132 } 00133 00134 bool LoRaHandler::action(uint8_t c) { 00135 if (_status != busy) { 00136 cmd = c; 00137 _thread.signal_set(signal); 00138 _thread.signal_clr(signal); 00139 return true; 00140 } 00141 00142 return false; 00143 } 00144 00145 LoRaHandler::LoRaStatus LoRaHandler::getStatus() { 00146 LoRaStatus status; 00147 _mutex.lock(); 00148 status = _status; 00149 _mutex.unlock(); 00150 00151 return status; 00152 } 00153 00154 LoRaHandler::LoRaLink LoRaHandler::getLinkCheckResults() { 00155 LoRaLink link; 00156 _mutex.lock(); 00157 link = _link; 00158 _mutex.unlock(); 00159 00160 return link; 00161 } 00162 00163 uint32_t LoRaHandler::getJoinAttempts() { 00164 uint32_t val; 00165 00166 _mutex.lock(); 00167 val = _join_attempts; 00168 _mutex.unlock(); 00169 00170 return val; 00171 } 00172 00173 void LoRaHandler::resetJoinAttempts() { 00174 _mutex.lock(); 00175 _join_attempts = 1; 00176 _mutex.unlock(); 00177 } 00178 00179 void LoRaHandler::blinker() { 00180 _activity_led = !_activity_led; 00181 } 00182 00183 void LoRaHandler::resetActivityLed() { 00184 _activity_led = red; 00185 } 00186 00187
Generated on Tue Jul 12 2022 13:07:49 by
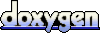