
Custom Channel Plan version of MTDOT Box firmware
Dependencies: DOGS102 GpsParser ISL29011 MMA845x MPL3115A2 MTS-Serial NCP5623B libmDot-Custom mDot_Channel_Plans
Fork of MTDOT-BOX-EVB-Factory-Firmware by
LayoutSemtech.cpp
00001 /* Copyright (c) <2016> <MultiTech Systems>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "LayoutSemtech.h" 00020 00021 00022 LayoutSemtech::LayoutSemtech(DOGS102* lcd, uint8_t band) 00023 : Layout(lcd), 00024 _band(band), 00025 _lDr(8,0,"DR"), 00026 _lFSB(0,0,"FSB"), 00027 _lSend(3,3,"Sending..."), 00028 _lTemp(8,6,"Temp "), 00029 _lNoGps(0,4,"No Gps Lock"), 00030 _lPower(13,0,"P"), 00031 _lBlank(0,1," "), 00032 _lNoLink(0,1,"Send Failed"), 00033 _lPadding(0,6,"Pad"), 00034 _lNoChannel(0,3,"No Free Channel"), 00035 _fDr(10,0,2), 00036 _fSw1(13,7,4), 00037 _fSw2(0,7,9), 00038 _fFSB(3,0,1), 00039 _fTemp(13,6,4), 00040 _fPower(14,0,2), 00041 _fNextCh(0,5,17), 00042 _fGpsLat(0,3,17), 00043 _fGpsLon(0,4,17), 00044 _fResult(3,3,16), 00045 _fGpsTime(0,5,16), 00046 _fDownSnr(12,2,5), 00047 _fPadding(4,6,3), 00048 _fDownRssi(0,2,11) 00049 {} 00050 00051 LayoutSemtech::~LayoutSemtech() {} 00052 00053 void LayoutSemtech::display() {} 00054 00055 void LayoutSemtech::initial() 00056 { 00057 writeLabel(_lBlank); 00058 } 00059 00060 void LayoutSemtech::display(bool success, mDot::snr_stats snr, mDot::rssi_stats rssi, int power, int fsb, int padding, int dr) 00061 { 00062 char buf[17]; 00063 size_t size; 00064 00065 clear(); 00066 startUpdate(); 00067 00068 writeLabel(_lDr); 00069 writeLabel(_lTemp); 00070 writeLabel(_lPower); 00071 writeLabel(_lPadding); 00072 writeLabel(_lFSB); 00073 00074 if(success) { 00075 size = snprintf(buf, sizeof(buf), "DWN %3d dbm", rssi.last); 00076 writeField(_fDownRssi, buf, size); 00077 00078 memset(buf, 0, sizeof(buf)); 00079 size = snprintf(buf, sizeof(buf), " %2.1f", (float)snr.last / 10.0); 00080 writeField(_fDownSnr, buf, size); 00081 } else { 00082 writeLabel(_lNoLink); 00083 } 00084 00085 memset(buf, 0, sizeof(buf)); 00086 size = snprintf(buf, sizeof(buf), "%d",dr); 00087 writeField(_fDr, buf, size, true); 00088 00089 memset(buf, 0, sizeof(buf)); 00090 size = snprintf(buf, sizeof(buf), "%d",power); 00091 writeField(_fPower, buf, size, true); 00092 00093 memset(buf, 0, sizeof(buf)); 00094 size = snprintf(buf, sizeof(buf), "%d",fsb); 00095 writeField(_fFSB, buf, size, true); 00096 00097 memset(buf, 0, sizeof(buf)); 00098 size = snprintf(buf, sizeof(buf), "%d",padding); 00099 writeField(_fPadding, buf, size, true); 00100 00101 endUpdate(); 00102 } 00103 00104 void LayoutSemtech::updateSw1(string Sw1, string Sw2, int dr, int power, int padding) 00105 { 00106 size_t size; 00107 char buf[17]; 00108 string temp; 00109 for(int i = Sw1.size(); i<4; i++) temp+=" "; 00110 temp+=Sw1; 00111 writeField(_fSw1, temp, true); 00112 startUpdate(); 00113 if(Sw2=="Data Rate") { 00114 memset(buf, 0, sizeof(buf)); 00115 size = snprintf(buf, sizeof(buf), "%d",dr); 00116 writeField(_fDr, buf, size, true); 00117 } else if(Sw2=="Power") { 00118 memset(buf, 0, sizeof(buf)); 00119 size = snprintf(buf, sizeof(buf), "%d",power); 00120 writeField(_fPower, buf, size, true); 00121 } else if(Sw2=="Padding") { 00122 memset(buf, 0, sizeof(buf)); 00123 size = snprintf(buf, sizeof(buf), "%d",padding); 00124 writeField(_fPadding, buf, size, true); 00125 } 00126 endUpdate(); 00127 } 00128 00129 void LayoutSemtech::updateSw2(string Sw2) 00130 { 00131 writeField(_fSw2, Sw2, true); 00132 } 00133 00134 void LayoutSemtech::sending() 00135 { 00136 clear(); 00137 writeLabel(_lSend); 00138 } 00139 00140 void LayoutSemtech::sendResult(string str) 00141 { 00142 clear(); 00143 writeField(_fResult,str,true); 00144 } 00145 00146 00147 void LayoutSemtech::updateNextCh(int count_down) 00148 { 00149 clear(); 00150 size_t size; 00151 char buf[17]; 00152 size = snprintf(buf, sizeof(buf), "Countdown:%d",count_down); 00153 writeField(_fNextCh, buf, size, true); 00154 writeLabel(_lNoChannel); 00155 } 00156 00157 void LayoutSemtech::updateStats(bool GPS, GPSPARSER::longitude lon, GPSPARSER::latitude lat, struct tm time, float temp) 00158 { 00159 char buf[17]; 00160 size_t size; 00161 00162 startUpdate(); 00163 00164 if(GPS) { 00165 size = snprintf(buf, sizeof(buf), "%d %d %d.%03d %c", 00166 abs(lon.degrees), 00167 lon.minutes, 00168 (lon.seconds * 6) / 1000, 00169 (lon.seconds * 6) % 1000, 00170 (lon.degrees > 0) ? 'E' : 'W'); 00171 writeField(_fGpsLon, buf, size, true); 00172 00173 memset(buf, 0, sizeof(buf)); 00174 size = snprintf(buf, sizeof(buf), "%d %d %d.%03d %c", 00175 abs(lat.degrees), 00176 lat.minutes, 00177 (lat.seconds * 6) / 1000, 00178 (lat.seconds * 6) % 1000, 00179 (lat.degrees > 0) ? 'N' : 'S'); 00180 writeField(_fGpsLat, buf, size, true); 00181 00182 memset(buf, 0, sizeof(buf)); 00183 size = snprintf(buf, sizeof(buf), "%02d:%02d %02d/%02d/%04d", 00184 time.tm_hour, 00185 time.tm_min, 00186 time.tm_mon + 1, 00187 time.tm_mday, 00188 time.tm_year + 1900); 00189 writeField(_fGpsTime, buf, size, true); 00190 00191 } else writeLabel(_lNoGps); 00192 00193 memset(buf, 0, sizeof(buf)); 00194 size = snprintf(buf, sizeof(buf), "%.1f",temp); 00195 writeField(_fTemp, buf, size, true); 00196 00197 endUpdate(); 00198 } 00199
Generated on Tue Jul 12 2022 13:07:49 by
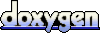