A library for talking to Multi-Tech's Cellular SocketModem Devices.
Dependents: M2X_dev axeda_wrapper_dev MTS_M2x_Example1 MTS_Cellular_Connect_Example ... more
Transport.h
00001 /* Universal Socket Modem Interface Library 00002 * Copyright (c) 2013 Multi-Tech Systems 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef TRANSPORT_H 00018 #define TRANSPORT_H 00019 00020 #include "mbed.h" 00021 #include "IPStack.h" 00022 00023 using namespace mts; 00024 00025 /** This class has been added to the standard mbed Socket library enabling people 00026 * to use the Socket library interfaces for different transports that have 00027 * their own internal IP-Stack. Use this class prior to instantiating any of the 00028 * other classes in this folder to determine the underlying transport that will 00029 * be used by them. It is important to know that the transport classes themsleves 00030 * like Cellular or WiFi, must be properly initialized and connected before any 00031 * of the Socket package classes can be used or even instantiated. 00032 */ 00033 class Transport 00034 { 00035 public: 00036 ///An enumeration that holds the supported Transport Types. 00037 enum TransportType { 00038 CELLULAR, WIFI, NONE, CUSTOM 00039 }; 00040 00041 /** This method allows you to set the transport to be used when creating other 00042 * objects from the Socket folder like TCPSocketConnection and UDPSocket. 00043 * 00044 * @param type the type of underlying transport to be used. The default is NONE. 00045 */ 00046 static void setTransport(TransportType type); 00047 00048 /** This method allows you to set the transport to be used when creatin other 00049 * objects from the Socket folder like TCPSocketConnection and UDPSocket. It 00050 * differs from the other setTransport method in that it allows for any transport 00051 * that derives from IPStack to be used with the native mbed Socket interfaces. 00052 * 00053 * @param type the type of underlying transport to be used as an IPStack object. 00054 */ 00055 static void setTransport(IPStack* type); 00056 00057 /** This method is used within the Socket class to get the appropraite transport 00058 * as an IPStack object. In general you do not need to call this directly, but 00059 * simply use the other classes in this folder. 00060 * 00061 * @returns a pointer to an object that implements IPStack. 00062 */ 00063 static IPStack* getInstance(); 00064 00065 private: 00066 static Transport::TransportType _type; // Member variable that holds the desired transport 00067 static IPStack* customType; //Member variable that holds an custom transport type. 00068 }; 00069 00070 #endif /* TRANSPORT_H */
Generated on Tue Jul 12 2022 21:46:23 by
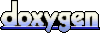