A library for talking to Multi-Tech's Cellular SocketModem Devices.
Dependents: M2X_dev axeda_wrapper_dev MTS_M2x_Example1 MTS_Cellular_Connect_Example ... more
TCPSocketConnection.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "TCPSocketConnection.h" 00020 #include <algorithm> 00021 00022 TCPSocketConnection::TCPSocketConnection() 00023 { 00024 } 00025 00026 int TCPSocketConnection::connect(const char* host, const int port) 00027 { 00028 if (!ip->open(host, port, mts::IPStack::TCP)) { 00029 return -1; 00030 } 00031 return 0; 00032 } 00033 00034 bool TCPSocketConnection::is_connected(void) 00035 { 00036 return ip->isOpen(); 00037 } 00038 00039 int TCPSocketConnection::send(char* data, int length) 00040 { 00041 Timer tmr; 00042 00043 if (!_blocking) { 00044 tmr.start(); 00045 while (tmr.read_ms() < _timeout) { 00046 if (ip->writeable()) 00047 break; 00048 } 00049 if (tmr.read_ms() >= _timeout) { 00050 return -1; 00051 } 00052 } 00053 return ip->write(data, length, 0); 00054 } 00055 00056 // -1 if unsuccessful, else number of bytes written 00057 int TCPSocketConnection::send_all(char* data, int length) 00058 { 00059 if (_blocking) { 00060 return ip->write(data, length, -1); 00061 } else { 00062 return ip->write(data, length, _timeout); 00063 } 00064 } 00065 00066 // -1 if unsuccessful, else number of bytes received 00067 int TCPSocketConnection::receive(char* data, int length) 00068 { 00069 Timer tmr; 00070 int time = -1; 00071 00072 if (!_blocking) { 00073 tmr.start(); 00074 while (time < _timeout + 20) { 00075 if (ip->readable()) { 00076 break; 00077 } 00078 time = tmr.read_ms(); 00079 } 00080 if (time >= _timeout + 20) { 00081 return -1; 00082 } 00083 } else { 00084 while(!ip->readable()); 00085 } 00086 00087 return ip->read(data, length, 0); 00088 } 00089 00090 00091 // -1 if unsuccessful, else number of bytes received 00092 int TCPSocketConnection::receive_all(char* data, int length) 00093 { 00094 if (_blocking) { 00095 return ip->read(data, length, -1); 00096 } else { 00097 return ip->read(data, length, _timeout); 00098 } 00099 }
Generated on Tue Jul 12 2022 21:46:23 by
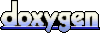