Class module for ISL29011 Ambient Light Sensor
Dependents: mDotEVBM2X MTDOT-EVBDemo-DRH MTDOT-BOX-EVB-Factory-Firmware-LIB-108 MTDOT-UDKDemo_Senet ... more
ISL29011.h
00001 /** 00002 * @file ISL29011.h 00003 * @brief Device driver - ISL29011 Ambient Light/IR Proximity Sensor 00004 * @author Tim Barr 00005 * @version 1.0 00006 * @see http://www.intersil.com/content/dam/Intersil/documents/isl2/isl29011.pdf 00007 * 00008 * Copyright (c) 2015 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 * 00022 */ 00023 00024 #ifndef ISL29011_H 00025 #define ISL29011_H 00026 00027 #include "mbed.h" 00028 00029 /** Using the Multitech MTDOT-EVB 00030 * 00031 * Example: 00032 * @code 00033 * #include "mbed.h" 00034 * #include "ISL29011.h" 00035 * 00036 00037 * 00038 * int main() 00039 * { 00040 00041 * } 00042 * @endcode 00043 */ 00044 00045 /** 00046 * @class ISL29011 00047 * @brief API abstraction for the ISL29011 Ambient Light/Proximity IC 00048 * initial version will be polling only. Interrupt service and rtos support will 00049 * be added at a later point 00050 */ 00051 class ISL29011 00052 { 00053 public: 00054 00055 /** 00056 * @static INT_FLG 00057 * @brief Interrupt flag bit 00058 */ 00059 uint8_t static const INT_FLG = 0x04; 00060 00061 /** 00062 * @static CT_16BIT 00063 * @brief Conversion time in usec for 16 bit setting 00064 */ 00065 uint32_t static const CT_16BIT = 90000; 00066 00067 /** 00068 * @static CT_12BIT 00069 * @brief conversion time in usec for 12 bit setting 00070 */ 00071 uint32_t static const CT_12BIT = 5630; 00072 00073 /** 00074 * @static CT_8BIT 00075 * @brief conversion time in usec for 8 bit setting 00076 */ 00077 uint32_t static const CT_8BIT = 351; 00078 00079 /** 00080 * @static CT_4BIT 00081 * @brief conversion time in usec for 4 bit setting 00082 */ 00083 uint32_t static const CT_4BIT = 22; 00084 00085 00086 /** 00087 * @enum OP_MODE 00088 * @brief operating mode of ILS29011 00089 */ 00090 enum OPERATION_MODE 00091 { 00092 PWR_DOWN = 0x00, 00093 ALS_ONCE = 0x20, 00094 IR_ONCE = 0x04, 00095 PROX_ONCE = 0xA0, 00096 ALS_CONT = 0xC0, 00097 IR_CONT = 0xE0, 00098 PROX_CONT 00099 }; 00100 00101 /** 00102 * @enum INT_PERSIST 00103 * @brief Number of cycles measurement needs to be out of threshold to generate an interrupt 00104 */ 00105 enum INT_PERSIST 00106 { 00107 NUMCYCLE_1 = 0x00, 00108 NUMCYCLE_2, NUMCYCLE_4, NUMCYCYLE_8, NUMCYCLE_16 00109 }; 00110 00111 /** 00112 * @enum PROX_SCHEME 00113 * @brief Dynamic Range scheme for IR proximity 00114 */ 00115 enum PROX_SCHEME 00116 { 00117 PROX_FULL = 0x00, /* full n (4,8,12,16) bit data */ 00118 PROX_NR = 0x80 /* n-1 (3,7,11,15) bit data */ 00119 }; 00120 00121 /** 00122 * @enum MOD_FREQ 00123 * @brief Modulation frequency of Proximity LED 00124 */ 00125 enum MOD_FREQ 00126 { 00127 FREQ_DC = 0x00, /* No modulation of LED */ 00128 FREQ_360k = 0x40 /* Proximity LED modulated at 360 kHz */ 00129 }; 00130 00131 /** 00132 * @enum LED_DRIVE 00133 * @brief Sets the drive current of the IR Proximity LED 00134 */ 00135 enum LED_DRIVE 00136 { 00137 LED_12_5 = 0x00, /* 12.5 mA current drive */ 00138 LED_25 = 0x10, /* 25 mA current drive */ 00139 LED_50 = 0x20, /* 50 mA current drive */ 00140 LED_100 = 0x30 /* 100 mA current driver */ 00141 }; 00142 00143 /** 00144 * @enum ADC_RESOLUTION 00145 * @brief Measurement resolution for ADC 00146 */ 00147 enum ADC_RESOLUTION 00148 { 00149 ADC_16BIT = 0x00, 00150 ADC_12BIT = 0x04, 00151 ADC_8BIT = 0x08, 00152 ADC_4BIT = 0x0B 00153 }; 00154 00155 /** 00156 * @enum LUX_RANGE 00157 * @brief Setting for LUX Range of ADC 00158 */ 00159 enum LUX_RANGE 00160 { 00161 RNG_1000 = 0x00, /* Full scale 1,000 LUX */ 00162 RNG_4000, /* Full scale 4,000 LUX */ 00163 RNG_16000, /* Full scale 16,000 LUX */ 00164 RNG_64000 /* Full scale 64,000 LUX */ 00165 }; 00166 00167 /** 00168 * @enum REGISTER 00169 * @brief The device register map 00170 */ 00171 enum REGISTER 00172 { 00173 COMMAND1 = 0x00, 00174 COMMAND2, DATA_LSB, DATA_MSB, INT_LT_LSB, INT_LT_MSB, INT_HT_LSB, INT_HT_MSB 00175 }; 00176 00177 /** Create the ISL29011 object 00178 * @param i2c - A defined I2C object 00179 * @param InterruptIn* - pointer to a defined InterruptIn object. Default to NULL if polled 00180 */ 00181 ISL29011(I2C &i2c, InterruptIn* isl_int = NULL); 00182 00183 /** Get the data 00184 * @return The last valid LUX reading from the ambient light sensor 00185 */ 00186 uint16_t getData(void); 00187 00188 /** Setup the ISL29011 measurement mode 00189 * @op_mode - Operating moe of sensor using the OPERATION_MODE enum 00190 * @return status of command 00191 */ 00192 uint8_t setMode(OPERATION_MODE op_mode) const; 00193 00194 /** Set Interrupt Threshold persistence 00195 * @int_persist - Sets the Interrupt Persistence Threshold using the INT_PERSIST enum 00196 * @return status of command 00197 * TODO - Still need to add interrupt support code 00198 */ 00199 uint8_t setPersistence(INT_PERSIST int_persist) const; 00200 00201 /** Set Proximity measurement parameters 00202 * @prox_scheme - Sets the Proximity measurement scheme using the PROX_SCHEME enum 00203 * @mod_freq - Sets the Moduletion Frequency using the MOD_FREQ enum 00204 * @led_drive - Sets the LED Drive current for Proximity mode using the LED_DRIVE enum 00205 * @return status of command 00206 * NOTE: function added for completeness. MTDOT-EVB does not have IR LED installed at this time 00207 */ 00208 uint8_t setProximity(PROX_SCHEME prox_scheme, MOD_FREQ mod_freq, LED_DRIVE led_drive) const; 00209 00210 /** Set ADC Resolution 00211 * @adc_resolution - Sets the ADC resolution using the ADC_RESOLUTION enum 00212 * @return status of command 00213 */ 00214 uint8_t setResolution(ADC_RESOLUTION adc_resolution) const; 00215 00216 /** Set the LUX Full Scale measurement range 00217 * @lux_range - Sets the maximum measured Lux value usngthe LUX_RANGE enum 00218 * @return status of command 00219 */ 00220 uint8_t setRange(LUX_RANGE lux_range ) const; 00221 00222 private: 00223 00224 I2C *_i2c; 00225 InterruptIn *_isl_int; 00226 bool _polling_mode; 00227 uint8_t static const _i2c_addr = (0x44 << 1); 00228 uint16_t _lux_data; 00229 00230 /* Initialize the ISL29011 device 00231 */ 00232 uint8_t init(void); 00233 00234 /* 00235 * Write to a register (exposed for debugging reasons) 00236 * Note: most writes are only valid in stop mode 00237 * @param reg - The register to be written 00238 * @param data - The data to be written 00239 */ 00240 uint8_t writeRegister(uint8_t const reg, uint8_t const data) const; 00241 00242 /* 00243 * Read from a register (exposed for debugging reasons) 00244 * @param reg - The register to read from 00245 * @return The register contents 00246 */ 00247 uint8_t readRegister(uint8_t const reg, char* data, uint8_t count = 1) const; 00248 00249 }; 00250 00251 #endif
Generated on Tue Jul 12 2022 18:25:00 by
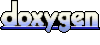