Class module for ISL29011 Ambient Light Sensor
Dependents: mDotEVBM2X MTDOT-EVBDemo-DRH MTDOT-BOX-EVB-Factory-Firmware-LIB-108 MTDOT-UDKDemo_Senet ... more
ISL29011.cpp
00001 /** 00002 * @file ISL29011.cpp 00003 * @brief Device driver - ISL29011 Ambient Light/IR Proximity Sensor IC 00004 * @author Tim Barr 00005 * @version 1.01 00006 * @see http://www.intersil.com/content/dam/Intersil/documents/isl2/isl29011.pdf 00007 * 00008 * Copyright (c) 2015 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 * 00022 * 1.01 TAB 7/6/15 Removed "= NULL" reference from object call. Only needs to be in .h file 00023 */ 00024 00025 #include "ISL29011.h" 00026 #include "mbed_debug.h" 00027 00028 ISL29011::ISL29011(I2C &i2c, InterruptIn* isl_int) 00029 { 00030 _i2c = &i2c; 00031 _isl_int = isl_int; 00032 00033 ISL29011::init(); 00034 00035 return; 00036 } 00037 00038 uint8_t ISL29011::init(void) 00039 { 00040 uint8_t result = 0; 00041 00042 // Reset all registers to POR values 00043 result = ISL29011::writeRegister(COMMAND1, 0x00); 00044 00045 if (result == 0) { 00046 if (_isl_int == NULL) 00047 _polling_mode = true; 00048 else _polling_mode = false; 00049 00050 result = ISL29011::writeRegister(COMMAND2, 0x00); 00051 result = ISL29011::writeRegister(INT_LT_LSB, 0x00); 00052 result = ISL29011::writeRegister(INT_LT_MSB, 0x00); 00053 result = ISL29011::writeRegister(INT_HT_LSB, 0xFF); 00054 result = ISL29011::writeRegister(INT_HT_MSB, 0xFF); 00055 } 00056 00057 if (result != 0) { 00058 debug("ILS29011:init failed\n\r"); 00059 } 00060 00061 return result; 00062 } 00063 00064 /** Get the data 00065 * @return The last valid LUX reading from the ambient light sensor 00066 */ 00067 uint16_t ISL29011::getData(void) 00068 { 00069 if (_polling_mode) { 00070 char datain[2]; 00071 00072 ISL29011::readRegister(DATA_LSB, datain, 2); 00073 _lux_data = (datain[1] << 8) | datain[0]; 00074 } 00075 return _lux_data; 00076 } 00077 00078 /** Setup the ISL29011 measurement mode 00079 * @return status of command 00080 */ 00081 uint8_t ISL29011::setMode(OPERATION_MODE op_mode) const 00082 { 00083 uint8_t result = 0; 00084 char datain[1]; 00085 char dataout; 00086 00087 00088 result |= ISL29011::readRegister(COMMAND1,datain); 00089 dataout = (datain[0] & 0x1F) | op_mode; 00090 result |= ISL29011::writeRegister(COMMAND1, dataout); 00091 return result; 00092 00093 } 00094 00095 /** Set Interrupt Persistence Threshold 00096 * @return status of command 00097 */ 00098 uint8_t ISL29011::setPersistence(INT_PERSIST int_persist) const 00099 { 00100 uint8_t result = 0; 00101 char datain[1]; 00102 char dataout; 00103 00104 result |= ISL29011::readRegister(COMMAND1,datain); 00105 dataout = (datain[0] & 0xFC) | int_persist; 00106 result |= ISL29011::writeRegister(COMMAND1, dataout); 00107 00108 return result; 00109 } 00110 00111 /** Set Proximity measurement parameters 00112 * @return status of command 00113 */ 00114 uint8_t ISL29011::setProximity(PROX_SCHEME prox_scheme, MOD_FREQ mod_freq, LED_DRIVE led_drive) const 00115 { 00116 uint8_t result = 0; 00117 char datain[1]; 00118 char dataout; 00119 00120 result |= ISL29011::readRegister(COMMAND2,datain); 00121 dataout = (datain[0] & 0x0F) | prox_scheme | mod_freq | led_drive; 00122 result |= ISL29011::writeRegister(COMMAND2, dataout); 00123 00124 return result; 00125 } 00126 00127 /** Set ADC Resolution 00128 * @return status of command 00129 */ 00130 uint8_t ISL29011::setResolution(ADC_RESOLUTION adc_resolution) const 00131 { 00132 uint8_t result = 0; 00133 char datain[1]; 00134 char dataout; 00135 00136 result |= ISL29011::readRegister(COMMAND2,datain); 00137 dataout = (datain[0] & 0xF3) | adc_resolution; 00138 result |= ISL29011::writeRegister(COMMAND2, dataout); 00139 00140 return result; 00141 } 00142 00143 /** Set the LUX Full Scale measurement range 00144 * @return status of command 00145 */ 00146 uint8_t ISL29011::setRange(LUX_RANGE lux_range ) const 00147 { 00148 uint8_t result = 0; 00149 char datain[1]; 00150 char dataout; 00151 00152 result |= ISL29011::readRegister(COMMAND2,datain); 00153 dataout = (datain[0] & 0xFC) | lux_range; 00154 result |= ISL29011::writeRegister(COMMAND2, dataout); 00155 00156 return result; 00157 } 00158 00159 00160 uint8_t ISL29011::writeRegister(uint8_t const reg, uint8_t const data) const 00161 { 00162 char buf[2] = {reg, data}; 00163 uint8_t result = 0; 00164 00165 buf[0] = reg; 00166 buf[1] = data; 00167 00168 result |= _i2c->write(_i2c_addr, buf, 2); 00169 00170 if (result != 0) { 00171 debug("ISL29011::writeRegister failed\n\r"); 00172 } 00173 00174 return result; 00175 } 00176 00177 uint8_t ISL29011::readRegister(uint8_t const reg, char* data, uint8_t count) const 00178 { 00179 uint8_t result = 0; 00180 char reg_out[1]; 00181 00182 reg_out[0] = reg; 00183 _i2c->lock(); 00184 result |= _i2c->write(_i2c_addr,reg_out,1,true); 00185 00186 if (result != 0) { 00187 debug("ISL29011::readRegister failed write\n\r"); 00188 goto exit; 00189 } 00190 00191 result |= _i2c->read(_i2c_addr,data,count,false); 00192 00193 if (result != 0) { 00194 debug("ISL29011::readRegister failed read\n\r"); 00195 } 00196 00197 exit: 00198 _i2c->unlock(); 00199 return result; 00200 }
Generated on Tue Jul 12 2022 18:25:00 by
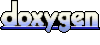