bugfix for duplicate symbol
Fork of nRF51822 by
Embed:
(wiki syntax)
Show/hide line numbers
app_error.c
Go to the documentation of this file.
00001 /* Copyright (c) 2014 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 */ 00012 00013 /** @file 00014 * 00015 * @defgroup app_error Common application error handler 00016 * @{ 00017 * @ingroup app_common 00018 * 00019 * @brief Common application error handler. 00020 */ 00021 00022 #include "nrf.h" 00023 #include "app_error.h " 00024 #include "compiler_abstraction.h" 00025 #include "nordic_common.h" 00026 #ifdef DEBUG 00027 #include "bsp.h" 00028 00029 /* global error variables - in order to prevent removal by optimizers */ 00030 uint32_t m_error_code; 00031 uint32_t m_line_num; 00032 const uint8_t * m_p_file_name; 00033 #endif 00034 00035 /**@brief Function for error handling, which is called when an error has occurred. 00036 * 00037 * @warning This handler is an example only and does not fit a final product. You need to analyze 00038 * how your product is supposed to react in case of error. 00039 * 00040 * @param[in] error_code Error code supplied to the handler. 00041 * @param[in] line_num Line number where the handler is called. 00042 * @param[in] p_file_name Pointer to the file name. 00043 * 00044 * Function is implemented as weak so that it can be overwritten by custom application error handler 00045 * when needed. 00046 */ 00047 00048 /*lint -save -e14 */ 00049 __WEAK void app_error_handler(uint32_t error_code, uint32_t line_num, const uint8_t * p_file_name) 00050 { 00051 // On assert, the system can only recover with a reset. 00052 #ifndef DEBUG 00053 NVIC_SystemReset(); 00054 #else 00055 00056 #ifdef BSP_DEFINES_ONLY 00057 LEDS_ON(LEDS_MASK); 00058 #else 00059 UNUSED_VARIABLE(bsp_indication_set(BSP_INDICATE_FATAL_ERROR)); 00060 // This call can be used for debug purposes during application development. 00061 // @note CAUTION: Activating this code will write the stack to flash on an error. 00062 // This function should NOT be used in a final product. 00063 // It is intended STRICTLY for development/debugging purposes. 00064 // The flash write will happen EVEN if the radio is active, thus interrupting 00065 // any communication. 00066 // Use with care. Uncomment the line below to use. 00067 //ble_debug_assert_handler(error_code, line_num, p_file_name); 00068 #endif // BSP_DEFINES_ONLY 00069 00070 m_error_code = error_code; 00071 m_line_num = line_num; 00072 m_p_file_name = p_file_name; 00073 00074 UNUSED_VARIABLE(m_error_code); 00075 UNUSED_VARIABLE(m_line_num); 00076 UNUSED_VARIABLE(m_p_file_name); 00077 __disable_irq(); 00078 while(1) ; 00079 #endif // DEBUG 00080 } 00081 /*lint -restore */
Generated on Tue Jul 12 2022 16:20:23 by
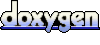