USB device stack
Dependents: blinky_max32630fthr FTHR_USB_serial FTHR_OLED HSP_RPC_GUI_3_0_1 ... more
Fork of USBDevice by
USBMouseKeyboard Class Reference
USBMouseKeyboard example. More...
#include <USBMouseKeyboard.h>
Inherits USBHID.
Public Member Functions | |
USBMouseKeyboard (MOUSE_TYPE mouse_type=REL_MOUSE, uint16_t vendor_id=0x0021, uint16_t product_id=0x0011, uint16_t product_release=0x0001) | |
Constructor. | |
bool | update (int16_t x, int16_t y, uint8_t buttons, int8_t z) |
Write a state of the mouse. | |
bool | move (int16_t x, int16_t y) |
Move the cursor to (x, y) | |
bool | press (uint8_t button) |
Press one or several buttons. | |
bool | release (uint8_t button) |
Release one or several buttons. | |
bool | doubleClick () |
Double click (MOUSE_LEFT) | |
bool | click (uint8_t button) |
Click. | |
bool | scroll (int8_t z) |
Scrolling. | |
bool | keyCode (uint8_t key, uint8_t modifier=0) |
To send a character defined by a modifier(CTRL, SHIFT, ALT) and the key. | |
virtual int | _putc (int c) |
Send a character. | |
bool | mediaControl (MEDIA_KEY key) |
Control media keys. | |
uint8_t | lockStatus () |
Read status of lock keys. | |
bool | send (HID_REPORT *report) |
Send a Report. | |
bool | sendNB (HID_REPORT *report) |
Send a Report. | |
bool | read (HID_REPORT *report) |
Read a report: blocking. | |
bool | readNB (HID_REPORT *report) |
Read a report: non blocking. |
Detailed Description
USBMouseKeyboard example.
#include "mbed.h" #include "USBMouseKeyboard.h" USBMouseKeyboard key_mouse; int main(void) { while(1) { key_mouse.move(20, 0); key_mouse.printf("Hello From MBED\r\n"); wait(1); } }
#include "mbed.h" #include "USBMouseKeyboard.h" USBMouseKeyboard key_mouse(ABS_MOUSE); int main(void) { while(1) { key_mouse.move(X_MAX_ABS/2, Y_MAX_ABS/2); key_mouse.printf("Hello from MBED\r\n"); wait(1); } }
Definition at line 70 of file USBMouseKeyboard.h.
Constructor & Destructor Documentation
USBMouseKeyboard | ( | MOUSE_TYPE | mouse_type = REL_MOUSE , |
uint16_t | vendor_id = 0x0021 , |
||
uint16_t | product_id = 0x0011 , |
||
uint16_t | product_release = 0x0001 |
||
) |
Constructor.
- Parameters:
-
mouse_type Mouse type: ABS_MOUSE (absolute mouse) or REL_MOUSE (relative mouse) (default: REL_MOUSE) leds Leds bus: first: NUM_LOCK, second: CAPS_LOCK, third: SCROLL_LOCK vendor_id Your vendor_id (default: 0x1234) product_id Your product_id (default: 0x0001) product_release Your preoduct_release (default: 0x0001)
Definition at line 84 of file USBMouseKeyboard.h.
Member Function Documentation
int _putc | ( | int | c ) | [virtual] |
Send a character.
- Parameters:
-
c character to be sent
- Returns:
- true if there is no error, false otherwise
Definition at line 653 of file USBMouseKeyboard.cpp.
bool click | ( | uint8_t | button ) |
Click.
- Parameters:
-
button state of the buttons ( ex: clic(MOUSE_LEFT))
- Returns:
- true if there is no error, false otherwise
Definition at line 636 of file USBMouseKeyboard.cpp.
bool doubleClick | ( | ) |
Double click (MOUSE_LEFT)
- Returns:
- true if there is no error, false otherwise
Definition at line 629 of file USBMouseKeyboard.cpp.
bool keyCode | ( | uint8_t | key, |
uint8_t | modifier = 0 |
||
) |
To send a character defined by a modifier(CTRL, SHIFT, ALT) and the key.
//To send CTRL + s (save) keyboard.keyCode('s', KEY_CTRL);
- Parameters:
-
modifier bit 0: KEY_CTRL, bit 1: KEY_SHIFT, bit 2: KEY_ALT (default: 0) key character to send
- Returns:
- true if there is no error, false otherwise
Definition at line 657 of file USBMouseKeyboard.cpp.
uint8_t lockStatus | ( | ) |
Read status of lock keys.
Useful to switch-on/off leds according to key pressed. Only the first three bits of the result is important:
- First bit: NUM_LOCK
- Second bit: CAPS_LOCK
- Third bit: SCROLL_LOCK
- Returns:
- status of lock keys
Definition at line 565 of file USBMouseKeyboard.cpp.
bool mediaControl | ( | MEDIA_KEY | key ) |
Control media keys.
- Parameters:
-
key media key pressed (KEY_NEXT_TRACK, KEY_PREVIOUS_TRACK, KEY_STOP, KEY_PLAY_PAUSE, KEY_MUTE, KEY_VOLUME_UP, KEY_VOLUME_DOWN)
- Returns:
- true if there is no error, false otherwise
Definition at line 690 of file USBMouseKeyboard.cpp.
bool move | ( | int16_t | x, |
int16_t | y | ||
) |
Move the cursor to (x, y)
- Parameters:
-
x x-axis position y y-axis position
- Returns:
- true if there is no error, false otherwise
Definition at line 621 of file USBMouseKeyboard.cpp.
bool press | ( | uint8_t | button ) |
Press one or several buttons.
- Parameters:
-
button button state (ex: press(MOUSE_LEFT))
- Returns:
- true if there is no error, false otherwise
Definition at line 643 of file USBMouseKeyboard.cpp.
bool read | ( | HID_REPORT * | report ) | [inherited] |
Read a report: blocking.
- Parameters:
-
report pointer to the report to fill
- Returns:
- true if successful
Definition at line 45 of file USBHID.cpp.
bool readNB | ( | HID_REPORT * | report ) | [inherited] |
Read a report: non blocking.
- Parameters:
-
report pointer to the report to fill
- Returns:
- true if successful
Definition at line 57 of file USBHID.cpp.
bool release | ( | uint8_t | button ) |
Release one or several buttons.
- Parameters:
-
button button state (ex: release(MOUSE_LEFT))
- Returns:
- true if there is no error, false otherwise
Definition at line 648 of file USBMouseKeyboard.cpp.
bool scroll | ( | int8_t | z ) |
Scrolling.
- Parameters:
-
z value of the wheel (>0 to go down, <0 to go up)
- Returns:
- true if there is no error, false otherwise
Definition at line 625 of file USBMouseKeyboard.cpp.
bool send | ( | HID_REPORT * | report ) | [inherited] |
Send a Report.
warning: blocking
- Parameters:
-
report Report which will be sent (a report is defined by all data and the length)
- Returns:
- true if successful
Definition at line 34 of file USBHID.cpp.
bool sendNB | ( | HID_REPORT * | report ) | [inherited] |
Send a Report.
warning: non blocking
- Parameters:
-
report Report which will be sent (a report is defined by all data and the length)
- Returns:
- true if successful
Definition at line 39 of file USBHID.cpp.
bool update | ( | int16_t | x, |
int16_t | y, | ||
uint8_t | buttons, | ||
int8_t | z | ||
) |
Write a state of the mouse.
- Parameters:
-
x x-axis position y y-axis position buttons buttons state (first bit represents MOUSE_LEFT, second bit MOUSE_RIGHT and third bit MOUSE_MIDDLE) z wheel state (>0 to scroll down, <0 to scroll up)
- Returns:
- true if there is no error, false otherwise
Definition at line 569 of file USBMouseKeyboard.cpp.
Generated on Wed Jul 13 2022 12:44:03 by
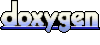