MAX7032 Transceiver Mbed Driver
Embed:
(wiki syntax)
Show/hide line numbers
Max7032_regs.h
00001 /******************************************************************************* 00002 * Copyright(C) Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files(the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc.shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc.Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc.retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #ifndef MAX7032_REGS_H_ 00035 #define MAX7032_REGS_H_ 00036 00037 /** 00038 * @brief POWER Register 00039 * 00040 * Address : 0x00 00041 */ 00042 typedef union { 00043 unsigned char raw; 00044 struct { 00045 unsigned char x : 1; /**< None Not used */ 00046 unsigned char rssio : 1; /**< RSSI amplifier enable 1 = Enable buffer 0 = Disable buffer */ 00047 unsigned char pa : 1; /**< Transmitter PA enable 1 = Enable PA 0 = Disable PA */ 00048 unsigned char pkdet : 1; /**< Peak-detector enable 1 = Enable peak detector 0 = Disable peak detector */ 00049 unsigned char baseb : 1; /**< Baseband enable 1 = Enable baseband 0 = Disable baseband */ 00050 unsigned char mixer : 1; /**< Mixer enable 1 = Enable mixer 0 = Disable mixer */ 00051 unsigned char agc : 1; /**< AGC enable 1 = Enable AGC 0 = Disable AGC */ 00052 unsigned char lna : 1; /**< LNA enable 1 = Enable LNA 0 = Disable LNA */ 00053 } bits; 00054 } max7032_power_t; 00055 00056 /** 00057 * @brief CONTRL Register 00058 * 00059 * Address : 0x01 00060 */ 00061 typedef union { 00062 unsigned char raw; 00063 struct { 00064 unsigned char sleep : 1; /**< Sleep mode 1 = Deep-sleep 00065 0 = Normal operation */ 00066 unsigned char ckout : 1; /**< Crystal clock output enable 1 = Enable crystal clock output 00067 0 = Disable crystal clock output */ 00068 unsigned char fcal : 1; /**< FSK calibration 1 = Perform FSK calibration Automatically */ 00069 unsigned char pcal : 1; /**< Polling timer calibration 1 = Perform polling timer calibration Automatically*/ 00070 unsigned char x : 1; /**< None Not used*/ 00071 unsigned char trk_en : 1; /**< Manual peak-detector tracking 1 = Force manual peak-detector tracking 00072 0 = Release peak-detector tracking*/ 00073 unsigned char gain : 1; /**< Gain state 1 = Force manual high-gain state if MGAIN = 1 00074 0 = Force manual low-gain state if MGAIN = 1*/ 00075 unsigned char agclk : 1; /**< AGC locking feature 1 = Enable AGC lock 00076 0 = Disable AGC lock*/ 00077 } bits; 00078 } max7032_contrl_t; 00079 00080 /** 00081 * @brief CONF0 Register 00082 * 00083 * Address : 0x02 00084 */ 00085 typedef union { 00086 unsigned char raw; 00087 struct { 00088 unsigned char onps : 2; /**< On-timer prescaler Sets the time base for the on timer */ 00089 unsigned char ofps : 2; /**< Off-timer prescaler Sets the time base for the off timer */ 00090 unsigned char drx : 1; /**< Discontinuous receive mode 1 = Enable DRX 00091 0 = Disable DRX */ 00092 unsigned char mgain : 1; /**< Manual gain mode 1 = Enable manual-gain mode 00093 0 = Disable manual-gain mode */ 00094 unsigned char t_r : 1; /**< Transmit or receive 1 = Enable transmit mode of the transceiver 00095 0 = Enable receive mode of the transceiver */ 00096 unsigned char mode : 1; /**< FSK or ASK modulation 1 = Enable FSK for both receive and transmit 00097 0 = Enable ASK for both receive and transmit */ 00098 } bits; 00099 } max7032_conf0_t; 00100 00101 /** 00102 * @brief CONF1 Register 00103 * 00104 * Address : 0x03 00105 */ 00106 typedef union { 00107 unsigned char raw; 00108 struct { 00109 unsigned char dt : 3; /**< AGC dwell timer AGC dwell timer */ 00110 unsigned char cdiv : 2; /**< Crystal divider CLKOUT crystal-divider */ 00111 unsigned char clkof : 1; /**< Continuous clock output 1 = Enable continuous clock output when CKOUT = 1 00112 0 = Continuous clock output */ 00113 unsigned char acal : 1; /**< Automatic FSK calibration 1 = Enable automatic FSK calibration 00114 0 = Disable automatic FSK calibration*/ 00115 unsigned char x : 1; /**< None Not used*/ 00116 } bits; 00117 } max7032_conf1_t; 00118 00119 /** 00120 * @brief OSC Register 00121 * 00122 * Address : 0x05 00123 */ 00124 typedef union { 00125 unsigned char raw; 00126 struct { 00127 unsigned char osc; 00128 } bits; 00129 } max7032_osc_t; 00130 00131 /** 00132 * @brief tOFF Register (Upper Byte) 00133 * 00134 * Address : 0x06 00135 */ 00136 typedef union { 00137 unsigned char raw; 00138 struct { 00139 unsigned char toff_upper; 00140 } bits; 00141 } max7032_toff_upper_t; 00142 00143 /** 00144 * @brief tOFF Register (Lower Byte) 00145 * 00146 * Address : 0x07 00147 */ 00148 typedef union { 00149 unsigned char raw; 00150 struct { 00151 unsigned char toff_lower; 00152 } bits; 00153 } max7032_toff_lower_t; 00154 00155 /** 00156 * @brief tCPU Register 00157 * 00158 * Address : 0x08 00159 */ 00160 typedef union { 00161 unsigned char raw; 00162 struct { 00163 unsigned char tcpu; 00164 } bits; 00165 } max7032_tcpu_t; 00166 00167 /** 00168 * @brief tRF Register (Upper Byte) 00169 * 00170 * Address : 0x09 00171 */ 00172 typedef union { 00173 unsigned char raw; 00174 struct { 00175 unsigned char trf_upper; 00176 } bits; 00177 } max7032_trf_upper_t; 00178 00179 /** 00180 * @brief tRF Register (Lower Byte) 00181 * 00182 * Address : 0x0A 00183 */ 00184 typedef union { 00185 unsigned char raw; 00186 struct { 00187 unsigned char trf_lower; 00188 } bits; 00189 } max7032_trf_lower_t; 00190 00191 /** 00192 * @brief tON Register (Upper Byte) 00193 * 00194 * Address : 0x0B 00195 */ 00196 typedef union { 00197 unsigned char raw; 00198 struct { 00199 unsigned char ton_upper; 00200 } bits; 00201 } max7032_ton_upper_t; 00202 00203 /** 00204 * @brief tON Register (Lower Byte) 00205 * 00206 * Address : 0x0C 00207 */ 00208 typedef union { 00209 unsigned char raw; 00210 struct { 00211 unsigned char ton_lower; 00212 } bits; 00213 } max7032_ton_lower_t; 00214 00215 /** 00216 * @brief TxLOW Register (Upper Byte) 00217 * 00218 * Address : 0x0D 00219 */ 00220 typedef union { 00221 unsigned char raw; 00222 struct { 00223 unsigned char txlow_upper; 00224 } bits; 00225 } max7032_txlow_upper_t; 00226 00227 /** 00228 * @brief TxLOW Register (Lower Byte) 00229 * 00230 * Address : 0x0E 00231 */ 00232 typedef union { 00233 unsigned char raw; 00234 struct { 00235 unsigned char txlow_lower; 00236 } bits; 00237 } max7032_txlow_lower_t; 00238 00239 /** 00240 * @brief TxHIGH Register (Upper Byte) 00241 * 00242 * Address : 0x0F 00243 */ 00244 typedef union { 00245 unsigned char raw; 00246 struct { 00247 unsigned char txhigh_upper; 00248 } bits; 00249 } max7032_txhigh_upper_t; 00250 00251 /** 00252 * @brief TxHIGH Register (Lower Byte) 00253 * 00254 * Address : 0x10 00255 */ 00256 typedef union { 00257 unsigned char raw; 00258 struct { 00259 unsigned char txhigh_lower; 00260 } bits; 00261 } max7032_txhigh_lower_t; 00262 00263 /** 00264 * @brief STATUS Register 00265 * 00266 * Address : 0x1A 00267 */ 00268 typedef union { 00269 unsigned char raw; 00270 struct { 00271 unsigned char fcald : 1; /**< FSK calibration done 1 = FSK calibration is completed 00272 0 = FSK calibration is in progress or not completed*/ 00273 unsigned char pcald : 1; /**< Polling timer calibration done 1 = Polling timer calibration is completed 00274 0 = Polling timer calibration is in progress or not completed*/ 00275 unsigned char x0 : 1; /**< None Zero*/ 00276 unsigned char x1 : 1; /**< None Zero*/ 00277 unsigned char x2 : 1; /**< None Zero*/ 00278 unsigned char clkon : 1; /**< Clock/crystal alive 1 = Valid clock at crystal inputs 00279 0 = No valid clock signal seen at the crystal inputs*/ 00280 unsigned char gains : 1; /**< AGC gain state 1 = LNA in high-gain state 00281 0 = LNA in low-gain state*/ 00282 unsigned char lckd : 1; /**< Lock detect 1 = Internal PLL is locked 00283 0 = Internal PLL is not locked */ 00284 } bits; 00285 } max7032_status_t; 00286 00287 /** 00288 * @brief DEMOD Register 00289 * 00290 * Address : 0x00 00291 */ 00292 typedef union { 00293 unsigned char raw; 00294 struct { 00295 unsigned char dummy_byte; 00296 00297 } bits; 00298 } max7032_dummy_t; 00299 00300 /** 00301 * @brief Register Set 00302 * 00303 * 00304 */ 00305 typedef struct { 00306 max7032_power_t power; 00307 max7032_contrl_t contrl; 00308 max7032_conf0_t conf0; 00309 max7032_conf1_t conf1; 00310 max7032_osc_t osc; 00311 max7032_toff_upper_t toff_upper; 00312 max7032_toff_lower_t toff_lower; 00313 max7032_tcpu_t tcpu; 00314 max7032_trf_upper_t trf_upper; 00315 max7032_trf_lower_t trf_lower; 00316 max7032_ton_upper_t ton_upper; 00317 max7032_ton_lower_t ton_lower; 00318 max7032_txlow_upper_t txlow_upper; 00319 max7032_txlow_lower_t txlow_lower; 00320 max7032_txhigh_upper_t txhigh_upper; 00321 max7032_txhigh_lower_t txhigh_lower; 00322 max7032_status_t status; 00323 } max7032_reg_map_t; 00324 00325 #endif /* MAX7032_REGS_H_ */
Generated on Thu Jul 14 2022 04:50:06 by
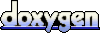