Timer based PWM output for MAX326XXFTHR platforms
Dependents: MAX32620FTHR_PwmBlinky
Fork of MAX326XXFTHR_PwmOut by
MAX326XXFTHR_PwmOut Class Reference
Timer based PWM output for the MAX326XXFTHR platform. More...
#include <MAX326XXFTHR_PwmOut.h>
Public Member Functions | |
MAX326XXFTHR_PwmOut (PinName pin) | |
Create a PwmOut connected to the specified pin. | |
void | write (float value) |
Set the ouput duty-cycle, specified as a percentage (float) | |
float | read () |
Return the current output duty-cycle setting, measured as a percentage (float) | |
void | period (float seconds) |
Set the PWM period, specified in seconds (float), keeping the duty cycle the same. | |
void | period_ms (int ms) |
Set the PWM period, specified in milli-seconds (int), keeping the duty cycle the same. | |
void | period_us (int us) |
Set the PWM period, specified in micro-seconds (int), keeping the duty cycle the same. | |
void | pulsewidth (float seconds) |
Set the PWM pulsewidth, specified in seconds (float), keeping the period the same. | |
void | pulsewidth_ms (int ms) |
Set the PWM pulsewidth, specified in milli-seconds (int), keeping the period the same. | |
void | pulsewidth_us (int us) |
Set the PWM pulsewidth, specified in micro-seconds (int), keeping the period the same. | |
MAX326XXFTHR_PwmOut & | operator= (float value) |
A operator shorthand for write() | |
MAX326XXFTHR_PwmOut & | operator= (PwmOut &rhs) |
A operator shorthand for write() | |
operator float () | |
An operator shorthand for read() | |
Protected Member Functions | |
void | lock_deep_sleep () |
Lock deep sleep only if it is not yet locked. | |
void | unlock_deep_sleep () |
Unlock deep sleep in case it is locked. |
Detailed Description
Timer based PWM output for the MAX326XXFTHR platform.
This library provides PWM output using the MAX326XX 32-bit timers. The mbed PwmOut API implementation uses the MAX326XX Pulse Train peripherals. The table below contains the available GPIO pins that can be connected to the six 32-bit timers (TMR0-5). Timer 0 is used for the microsecond ticker API and is not available for PWM output. On the MAX32630FTHR platform, timer 5 is used by the BLE API and will not be available for PWM output if the BLE API is used.
MAX32620FTHR 32-Bit Timer PWM Mapping
Timer | GPIO Port and Pin
TMR1 | P0_1, P0_7, P1_5, P3_1, P4_5, P5_3
TMR2 | P0_2, P1_0, P1_6, P2_4, P3_2, P4_0, P4_6, P5_4
TMR3 | P0_3 P1_1 P1_7, P2_5, P3_3, P4_7, P5_5
TMR4 | P0_4 P1_2 P2_6, P3_4, P5_0, P5_6
TMR5 | P0_5 P1_3 P2_7, P3_5, P5_1, P5_7
MAX32630FTHR 32-Bit Timer PWM Mapping
Timer | GPIO Port and Pin
TMR1 | P3_1, P5_3
TMR2 | P2_4, P3_2, P4_0, P5_4
TMR3 | P2_5, P3_3, P5_5
TMR4 | P2_6, P3_4, P5_0, P5_6
TMR5 | P3_5, P5_1
Note that for MAX32620FTHR and MAX32630FTHR GPIOs P2_4, P2_5 and P2_6 are connected to onboard LEDs 1, 2 and 3.
#include "mbed.h" #include "MAX326XXFTHR_PwmOut.h" MAX326XXFTHR_PwmOut led[] = { MAX326XXFTHR_PwmOut(LED1), MAX326XXFTHR_PwmOut(LED2), MAX326XXFTHR_PwmOut(LED3) }; int main() { float dc; unsigned int idx = 0; while (1) { for (dc = 0.0f; dc <= 1.0f; dc += 0.01f) { led[idx % 3].write(dc); led[(idx + 1) % 3].write(1.0f - dc); wait_ms(20); } idx++; } }
Definition at line 89 of file MAX326XXFTHR_PwmOut.h.
Constructor & Destructor Documentation
MAX326XXFTHR_PwmOut | ( | PinName | pin ) |
Create a PwmOut connected to the specified pin.
- Parameters:
-
pin PwmOut pin to connect to
Definition at line 97 of file MAX326XXFTHR_PwmOut.h.
Member Function Documentation
void lock_deep_sleep | ( | ) | [protected] |
Lock deep sleep only if it is not yet locked.
Definition at line 226 of file MAX326XXFTHR_PwmOut.h.
operator float | ( | ) |
An operator shorthand for read()
- See also:
- PwmOut::read()
Definition at line 219 of file MAX326XXFTHR_PwmOut.h.
MAX326XXFTHR_PwmOut& operator= | ( | float | value ) |
A operator shorthand for write()
- See also:
- PwmOut::write()
Definition at line 201 of file MAX326XXFTHR_PwmOut.h.
MAX326XXFTHR_PwmOut& operator= | ( | PwmOut & | rhs ) |
A operator shorthand for write()
- See also:
- PwmOut::write()
Definition at line 210 of file MAX326XXFTHR_PwmOut.h.
void period | ( | float | seconds ) |
Set the PWM period, specified in seconds (float), keeping the duty cycle the same.
- Parameters:
-
seconds Change the period of a PWM signal in seconds (float) without modifying the duty cycle
- Note:
- The resolution is currently in microseconds; periods smaller than this will be set to zero.
Definition at line 147 of file MAX326XXFTHR_PwmOut.h.
void period_ms | ( | int | ms ) |
Set the PWM period, specified in milli-seconds (int), keeping the duty cycle the same.
- Parameters:
-
ms Change the period of a PWM signal in milli-seconds without modifying the duty cycle
Definition at line 156 of file MAX326XXFTHR_PwmOut.h.
void period_us | ( | int | us ) |
Set the PWM period, specified in micro-seconds (int), keeping the duty cycle the same.
- Parameters:
-
us Change the period of a PWM signal in micro-seconds without modifying the duty cycle
Definition at line 165 of file MAX326XXFTHR_PwmOut.h.
void pulsewidth | ( | float | seconds ) |
Set the PWM pulsewidth, specified in seconds (float), keeping the period the same.
- Parameters:
-
seconds Change the pulse width of a PWM signal specified in seconds (float)
Definition at line 174 of file MAX326XXFTHR_PwmOut.h.
void pulsewidth_ms | ( | int | ms ) |
Set the PWM pulsewidth, specified in milli-seconds (int), keeping the period the same.
- Parameters:
-
ms Change the pulse width of a PWM signal specified in milli-seconds
Definition at line 183 of file MAX326XXFTHR_PwmOut.h.
void pulsewidth_us | ( | int | us ) |
Set the PWM pulsewidth, specified in micro-seconds (int), keeping the period the same.
- Parameters:
-
us Change the pulse width of a PWM signal specified in micro-seconds
Definition at line 192 of file MAX326XXFTHR_PwmOut.h.
float read | ( | ) |
Return the current output duty-cycle setting, measured as a percentage (float)
- Returns:
- A floating-point value representing the current duty-cycle being output on the pin, measured as a percentage. The returned value will lie between 0.0f (representing on 0%) and 1.0f (representing on 100%).
- Note:
- This value may not match exactly the value set by a previous write().
Definition at line 133 of file MAX326XXFTHR_PwmOut.h.
void unlock_deep_sleep | ( | ) | [protected] |
Unlock deep sleep in case it is locked.
Definition at line 234 of file MAX326XXFTHR_PwmOut.h.
void write | ( | float | value ) |
Set the ouput duty-cycle, specified as a percentage (float)
- Parameters:
-
value A floating-point value representing the output duty-cycle, specified as a percentage. The value should lie between 0.0f (representing on 0%) and 1.0f (representing on 100%). Values outside this range will be saturated to 0.0f or 1.0f.
Definition at line 116 of file MAX326XXFTHR_PwmOut.h.
Generated on Thu Jul 14 2022 04:34:27 by
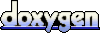