
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
Test_S25FS512.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "Test_S25FS512.h" 00034 #include "Test_Utilities.h" 00035 00036 //****************************************************************************** 00037 void test_S25FS512(void (*outputString)(const char *)) { 00038 char tempStr[32]; 00039 uint8_t page[264]; 00040 int totalPass = 1; 00041 int pass; 00042 int i; 00043 uint8_t data[128]; 00044 S25FS512 *s25FS512; 00045 00046 s25FS512 = Peripherals::s25FS512(); 00047 00048 // display header 00049 outputString("Testing S25FS512|"); 00050 00051 // read id test 00052 s25FS512->readIdentification(data, sizeof(data)); 00053 s25FS512->readIdentification(data, sizeof(data)); 00054 if ((data[1] == 0x01) && (data[2] == 0x02) && (data[3] == 0x19) && 00055 (data[4] == 0x4D)) { 00056 sprintf(tempStr, "Read ID: Pass "); 00057 outputString(tempStr); 00058 } else { 00059 sprintf(tempStr, "Read ID: Fail "); 00060 outputString(tempStr); 00061 totalPass = 0; 00062 } 00063 sprintf(tempStr, "(%02X%02X%02X%02X)|", data[1], data[2], data[3], data[4]); 00064 outputString(tempStr); 00065 00066 00067 if (totalPass == 1) { 00068 // format sector 0 00069 outputString("Formatting Sector 0, "); 00070 s25FS512->sectorErase_Helper(0); 00071 00072 // verify format sector 0 00073 outputString("Verifying Format of Sector 0: "); 00074 s25FS512->readPages_Helper(0, 0, page, 0); 00075 pass = s25FS512->isPageEmpty(page); 00076 _printPassFail(pass, 1, outputString); 00077 totalPass &= pass; 00078 00079 // fill page with random pattern 00080 for (i = 0; i < 256; i++) { 00081 page[i] = i; 00082 } 00083 // write to page 0 00084 outputString("Writing Page 0 to pattern, "); 00085 s25FS512->writePage_Helper(0, page, 0); 00086 // clear pattern in memory 00087 for (i = 0; i < 256; i++) { 00088 page[i] = 0x00; 00089 } 00090 // read back page and verify 00091 outputString("Read Page Verify: "); 00092 s25FS512->readPages_Helper(0, 0, page, 0); 00093 // compare memory for pattern 00094 pass = 1; 00095 for (i = 0; i < 256; i++) { 00096 if (page[i] != i) 00097 pass = 0; 00098 } 00099 _printPassFail(pass, 1, outputString); 00100 totalPass &= pass; 00101 00102 // format sector 0 to clean up after tests 00103 s25FS512->sectorErase_Helper(0); 00104 } else { 00105 outputString("Read Id Failed, Skipping rest of test|"); 00106 } 00107 00108 // final results 00109 outputString("Result: "); 00110 _printPassFail(totalPass, 0, outputString); 00111 }
Generated on Tue Jul 12 2022 17:59:20 by
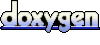