
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
Test_MAX30001.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "Test_MAX30001.h" 00034 #include "Test_Utilities.h" 00035 00036 uint32_t testing_max30001 = 0; 00037 uint32_t testing_ecg_flags[4]; 00038 #define TESTING_TIMEOUT_SECONDS 10 00039 00040 //****************************************************************************** 00041 void test_MAX30001(void (*outputString)(const char *)) { 00042 int totalPass = 1; 00043 uint32_t foundEcg = 0; 00044 uint32_t foundBioz = 0; 00045 uint32_t foundPace = 0; 00046 uint32_t foundRtoR = 0; 00047 uint32_t id; 00048 char str2[128]; 00049 int partVersion; // 0 = 30004 00050 // 1 = 30001 00051 // 2 = 30002 00052 // 3 = 30003 00053 Timer timer; 00054 MAX30001 *max30001; 00055 max30001 = Peripherals::max30001(); 00056 00057 // read the id 00058 max30001->reg_read(MAX30001::INFO, &id); 00059 // read id twice because it needs to be read twice 00060 max30001->reg_read(MAX30001::INFO, &id); 00061 partVersion = id >> 12; 00062 partVersion = partVersion & 0x3; 00063 00064 // display header 00065 if (partVersion == 0) 00066 outputString("Testing MAX30004|"); 00067 if (partVersion == 1) { 00068 outputString("Testing MAX30001|"); 00069 outputString("Testing ECG, RtoR, BioZ, PACE|"); 00070 } 00071 if (partVersion == 2) 00072 outputString("Testing MAX30002|"); 00073 if (partVersion == 3) { 00074 outputString("Testing MAX30003|"); 00075 outputString("Only Testing ECG and RtoR|"); 00076 } 00077 sprintf(str2, "Device ID = 0x%06X|", (unsigned int)id); 00078 outputString(str2); 00079 00080 // clear testing flags 00081 testing_ecg_flags[TESTING_ECG_FLAG] = 0; 00082 testing_ecg_flags[TESTING_BIOZ_FLAG] = 0; 00083 testing_ecg_flags[TESTING_PACE_FLAG] = 0; 00084 testing_ecg_flags[TESTING_RTOR_FLAG] = 0; 00085 00086 // start streams 00087 testing_max30001 = 1; 00088 if (partVersion == 1) 00089 outputString("Start Streaming ECG, RtoR, PACE, BIOZ, CAL enabled, " 00090 "verifying streams...|"); 00091 if (partVersion == 3) 00092 outputString( 00093 "Start Streaming ECG, RtoR, CAL enabled, verifying streams...|"); 00094 // CAL_InitStart(0b1, 0b1, 0b1, 0b011, 0x7FF, 0b0); 00095 max30001->CAL_InitStart(0b1, 0b1, 0b1, 0b011, 0x7FF, 0b0); 00096 max30001->ECG_InitStart(0b1, 0b1, 0b1, 0b0, 0b10, 0b11, 0x1F, 0b00, 00097 0b00, 0b0, 0b01); 00098 if (partVersion == 1) 00099 max30001->PACE_InitStart(0b1, 0b0, 0b0, 0b1, 0x0, 0b0, 0b00, 0b0, 00100 0b0); 00101 if (partVersion == 1) 00102 max30001->BIOZ_InitStart(0b1, 0b1, 0b1, 0b10, 0b11, 0b00, 7, 0b0, 00103 0b010, 0b0, 0b10, 0b00, 0b00, 2, 0b0, 00104 0b111, 0b0000); 00105 max30001->RtoR_InitStart(0b1, 0b0011, 0b1111, 0b00, 0b0011, 0b000001, 00106 0b00, 0b000, 0b01); 00107 max30001->Rbias_FMSTR_Init(0b01, 0b10, 0b1, 0b1, 0b00); 00108 max30001->synch(); 00109 00110 // look for each stream 00111 timer.start(); 00112 while (1) { 00113 if ((foundEcg == 0) && (testing_ecg_flags[TESTING_ECG_FLAG] == 1)) { 00114 foundEcg = 1; 00115 outputString("ECG Stream: PASS|"); 00116 } 00117 if ((foundBioz == 0) && (testing_ecg_flags[TESTING_BIOZ_FLAG] == 1)) { 00118 foundBioz = 1; 00119 outputString("Bioz Stream: PASS|"); 00120 } 00121 if ((foundPace == 0) && (testing_ecg_flags[TESTING_PACE_FLAG] == 1)) { 00122 foundPace = 1; 00123 outputString("PACE Stream: PASS|"); 00124 } 00125 if ((foundRtoR == 0) && (testing_ecg_flags[TESTING_RTOR_FLAG] == 1)) { 00126 foundRtoR = 1; 00127 outputString("RtoR Stream: PASS|"); 00128 } 00129 if ((foundEcg == 1) && (foundBioz == 1) && (foundPace == 1) && 00130 (foundRtoR == 1) && (partVersion == 1)) { 00131 break; 00132 } 00133 if ((foundEcg == 1) && (foundRtoR == 1) && (partVersion == 3)) { 00134 break; 00135 } 00136 if (timer.read() >= TESTING_TIMEOUT_SECONDS) { 00137 break; 00138 } 00139 } 00140 timer.stop(); 00141 if (foundEcg == 0) { 00142 outputString("ECG Stream: FAIL|"); 00143 totalPass = 0; 00144 } 00145 if ((foundBioz == 0) && (partVersion == 1)) { 00146 outputString("Bioz Stream: FAIL|"); 00147 totalPass = 0; 00148 } 00149 if ((foundPace == 0) && (partVersion == 1)) { 00150 outputString("PACE Stream: FAIL|"); 00151 totalPass = 0; 00152 } 00153 if (foundRtoR == 0) { 00154 outputString("RtoR Stream: FAIL|"); 00155 totalPass = 0; 00156 } 00157 00158 // stop all streams 00159 max30001->Stop_ECG(); 00160 if (partVersion == 1) 00161 max30001->Stop_PACE(); 00162 if (partVersion == 1) 00163 max30001->Stop_BIOZ(); 00164 max30001->Stop_RtoR(); 00165 testing_max30001 = 0; 00166 // final results 00167 outputString("Result: "); 00168 _printPassFail(totalPass, 0, outputString); 00169 }
Generated on Tue Jul 12 2022 17:59:20 by
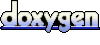