
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
StringInOut.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "mbed.h" 00034 #include "USBSerial.h" 00035 #include "RpcFifo.h" 00036 #include "RpcServer.h" 00037 #include "StringInOut.h" 00038 #include "Peripherals.h" 00039 00040 /// a running index that keeps track of where an incoming string has been 00041 /// buffered to 00042 static int lineBuffer_index = 0; 00043 /// a flag that keeps track of the state of accumulating a string 00044 static int getLine_State = GETLINE_WAITING; 00045 00046 extern USBSerial *usbSerialPtr; 00047 00048 /** 00049 * @brief Place incoming USB characters into a fifo 00050 * @param data_IN buffer of characters 00051 * @param len length of data 00052 */ 00053 int fifoIncomingChars(uint8_t data_IN[], unsigned int len) { 00054 unsigned int i; 00055 for (i = 0; i < len; i++) { 00056 fifo_put8(GetUSBIncomingFifo(), data_IN[i]); 00057 } 00058 return 0; 00059 } 00060 00061 /** 00062 * @brief Check the USB incoming fifo to see if there is data to be read 00063 * @return 1 if there is data to be read, 0 if data is not available 00064 */ 00065 int isReadReady(void) { 00066 if (fifo_empty(GetUSBIncomingFifo()) == 0) 00067 return 1; 00068 return 0; 00069 } 00070 00071 /** 00072 * @brief Clear the incoming USB read fifo 00073 */ 00074 void clearOutReadFifo(void) { fifo_clear(GetUSBIncomingFifo()); } 00075 00076 /** 00077 * @brief Block until a character can be read from the USB 00078 * @return the character read 00079 */ 00080 char getch(void) { 00081 uint8_t ch; 00082 // block until char is ready 00083 while (isReadReady() == 0) { 00084 } 00085 // read a char from buffer 00086 fifo_get8(GetUSBIncomingFifo(), &ch); 00087 return ch; 00088 } 00089 00090 /** 00091 * @brief Place incoming USB characters into a fifo 00092 * @param lineBuffer buffer to place the incoming characters 00093 * @param bufferLength length of buffer 00094 * @return GETLINE_WAITING if still waiting for a CRLF, GETLINE_DONE 00095 */ 00096 int getLine(char *lineBuffer, int bufferLength) { 00097 uint8_t ch; 00098 00099 //USBSerial *serial = Peripherals::usbSerial(); 00100 if (getLine_State == GETLINE_DONE) { 00101 getLine_State = GETLINE_WAITING; 00102 } 00103 if (usbSerialPtr->available() != 0) { 00104 ch = usbSerialPtr->_getc(); 00105 if (ch != 0x0A && ch != 0x0D) { 00106 lineBuffer[lineBuffer_index++] = ch; 00107 } 00108 if (ch == 0x0D) { 00109 lineBuffer[lineBuffer_index++] = 0; 00110 lineBuffer_index = 0; 00111 getLine_State = GETLINE_DONE; 00112 } 00113 if (lineBuffer_index > bufferLength) { 00114 lineBuffer[bufferLength - 1] = 0; 00115 getLine_State = GETLINE_DONE; 00116 } 00117 } 00118 return getLine_State; 00119 } 00120 00121 /** 00122 * @brief Block until a fixed number of characters has been accumulated from the 00123 * incoming USB 00124 * @param lineBuffer buffer to place the incoming characters 00125 * @param maxLength length of buffer 00126 */ 00127 void getStringFixedLength(uint8_t *lineBuffer, int maxLength) { 00128 uint8_t ch; 00129 int index = 0; 00130 // block until maxLength is captured 00131 while (1) { 00132 ch = getch(); 00133 lineBuffer[index++] = ch; 00134 if (index == maxLength) 00135 return; 00136 } 00137 } 00138 00139 /** 00140 * @brief Outut an array of bytes out the USB serial port 00141 * @param data buffer to output 00142 * @param length length of buffer 00143 */ 00144 int putBytes(uint8_t *data, uint32_t length) { 00145 int sent; 00146 int send; 00147 bool status; 00148 uint8_t *ptr; 00149 ptr = data; 00150 // 00151 // break up the string into chunks 00152 // 00153 sent = 0; 00154 do { 00155 send = MAX_PACKET_SIZE_EPBULK; 00156 if ((sent + MAX_PACKET_SIZE_EPBULK) > (int)length) { 00157 send = length - sent; 00158 } 00159 status = usbSerialPtr->writeBlock(ptr,send); 00160 if (status != true) { 00161 while (1) ; 00162 } 00163 sent += send; 00164 ptr += send; 00165 } while (sent < (int)length); 00166 return 0; 00167 } 00168 00169 /** 00170 * @brief Output a string out the USB serial port 00171 * @param str to output 00172 */ 00173 int putStr(const char *str) { 00174 int length; 00175 uint8_t *ptr; 00176 ptr = (uint8_t *)str; 00177 length = strlen(str); 00178 putBytes(ptr, length); 00179 return 0; 00180 }
Generated on Tue Jul 12 2022 17:59:19 by
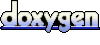