
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
StringHelper.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "mbed.h" 00034 #include "StringHelper.h" 00035 00036 /** 00037 * @brief Process an array of hex alpha numeric strings representing arguments of 00038 * type uint8 00039 * @param args Array of strings to process 00040 * @param argsUintPtr Pointer of uint8 to save converted arguments 00041 * @param numberOf Number of strings to convert 00042 */ 00043 void ProcessArgs(char args[32][32], uint8_t *argsUintPtr, int numberOf) { 00044 int i; 00045 int val; 00046 for (i = 0; i < numberOf; i++) { 00047 sscanf(args[i], "%x", &val); 00048 argsUintPtr[i] = (uint8_t)val; 00049 } 00050 } 00051 00052 /** 00053 * @brief Process an array of hex alpha numeric strings representing arguments of 00054 * type uint32 00055 * @param args Array of strings to process 00056 * @param argsUintPtr Pointer of uint32 to save converted arguments 00057 * @param numberOf Number of strings to convert 00058 */ 00059 void ProcessArgs32(char args[32][32], uint32_t *argsUintPtr, int numberOf) { 00060 int i; 00061 int val; 00062 for (i = 0; i < numberOf; i++) { 00063 sscanf(args[i], "%x", &val); 00064 argsUintPtr[i] = val; 00065 } 00066 } 00067 00068 /** 00069 * @brief Process an array of decimal numeric strings representing arguments of 00070 * type uint32 00071 * @param args Array of strings to process 00072 * @param argsUintPtr Pointer of uint32 to save converted arguments 00073 * @param numberOf Number of strings to convert 00074 */ 00075 void ProcessArgs32Dec(char args[32][32], uint32_t *argsUintPtr, int numberOf) { 00076 int i; 00077 int val; 00078 for (i = 0; i < numberOf; i++) { 00079 sscanf(args[i], "%d", &val); 00080 argsUintPtr[i] = val; 00081 } 00082 } 00083 00084 /** 00085 * @brief Parse a single string in decimal format to a uint32 number 00086 * @param str String to process 00087 * @returns Returns the converted number of type uint32 00088 */ 00089 uint32_t ParseAsciiDecU32(char *str) { 00090 unsigned int val; 00091 sscanf(str, "%u", &val); 00092 return (uint32_t)val; 00093 } 00094 00095 /** 00096 * @brief Parse a single string in hex format to a uint32 number 00097 * @param str String to process 00098 * @returns Returns the converted number of type uint32 00099 */ 00100 uint32_t ParseAsciiHexU32(char *str) { 00101 unsigned int val; 00102 sscanf(str, "%x", &val); 00103 return (uint32_t)val; 00104 } 00105 00106 /** 00107 * @brief Process a string that is "embedded" within another string using the /" 00108 * delimiters 00109 * @brief Extract the string 00110 * @param str String to process 00111 * @returns Returns the string 00112 */ 00113 void ProcessString(char args[32][32], uint8_t *str, int numberOf) { 00114 int i; 00115 int start; 00116 uint8_t *ptr; 00117 uint8_t *strPtr; 00118 ptr = (uint8_t *)args; 00119 strPtr = str; 00120 start = 0; 00121 for (i = 0; i < numberOf; i++) { 00122 if ((start == 0) && (*ptr == '\"')) { 00123 start = 1; 00124 ptr++; 00125 continue; 00126 } 00127 if ((start == 1) && (*ptr == '\"')) { 00128 break; 00129 } 00130 if (start == 1) { 00131 *strPtr = *ptr; 00132 strPtr++; 00133 } 00134 ptr++; 00135 } 00136 // terminate the string 00137 *strPtr = 0; 00138 } 00139 00140 /** 00141 * @brief Parse an incoming string until a CRLF is encountered, dst will contain 00142 * the parsed string 00143 * @param src source string to process 00144 * @param dst destination string to contain the parsed incoming string 00145 * @param length length of incoming src string 00146 * @returns updated pointer in src string 00147 */ 00148 char *ParseUntilCRLF(char *src, char *dst, int length) { 00149 int i; 00150 char *srcPtr; 00151 00152 srcPtr = src; 00153 i = 0; 00154 *dst = 0; 00155 while ((*srcPtr != 0) && (*srcPtr != 13)) { 00156 dst[i] = *srcPtr; 00157 i++; 00158 if (i >= length) 00159 break; 00160 srcPtr++; 00161 } 00162 if (*srcPtr == 13) 00163 srcPtr++; 00164 if (*srcPtr == 10) 00165 srcPtr++; 00166 dst[i] = 0; // terminate the string 00167 return srcPtr; 00168 } 00169 00170 /** 00171 * @brief Parse an incoming string hex value into an 8-bit value 00172 * @param str string to process 00173 * @returns 8-bit byte that is parsed from the string 00174 */ 00175 uint8_t StringToByte(char str[32]) { 00176 int val; 00177 uint8_t byt; 00178 sscanf(str, "%x", &val); 00179 byt = (uint8_t)val; 00180 return byt; 00181 } 00182 00183 /** 00184 * @brief Parse an incoming string hex value into 32-bit value 00185 * @param str string to process 00186 * @returns 32-bit value that is parsed from the string 00187 */ 00188 uint32_t StringToInt(char str[32]) { 00189 int val; 00190 uint32_t byt; 00191 sscanf(str, "%x", &val); 00192 byt = (uint32_t)val; 00193 return byt; 00194 } 00195 00196 /** 00197 * @brief Format a binary 8-bit array into a string 00198 * @param uint8Ptr byte buffer to process 00199 * @param numberOf number of bytes in the buffer 00200 * @param reply an array of strings to place the converted array values into 00201 */ 00202 void FormatReply(uint8_t *uint8Ptr, int numberOf, char reply[32][32]) { 00203 int i; 00204 for (i = 0; i < numberOf; i++) { 00205 sprintf(reply[i], "%02X", uint8Ptr[i]); 00206 } 00207 strcpy(reply[i], "\0"); 00208 } 00209 00210 /** 00211 * @brief Format a binary 32-bit array into a string 00212 * @param uint32Ptr 32-bit value buffer to process 00213 * @param numberOf number of values in the buffer 00214 * @param reply an array of strings to place the converted array values into 00215 */ 00216 void FormatReply32(uint32_t *uint32Ptr, int numberOf, char reply[32][32]) { 00217 int i; 00218 for (i = 0; i < numberOf; i++) { 00219 sprintf(reply[i], "%02X", (unsigned int)uint32Ptr[i]); 00220 } 00221 strcpy(reply[i], "\0"); 00222 } 00223 00224 /** 00225 * @brief Format a binary 8-bit array into a string 00226 * @param data 8-bit value buffer to process 00227 * @param length number of values in the buffer 00228 * @param str output string buffer 00229 * @return output string 00230 */ 00231 char *BytesToHexStr(uint8_t *data, uint32_t length, char *str) { 00232 uint32_t i; 00233 char tmpStr[8]; 00234 str[0] = 0; 00235 for (i = 0; i < length; i++) { 00236 sprintf(tmpStr, "%02X ", data[i]); 00237 strcat(str, tmpStr); 00238 } 00239 return str; 00240 }
Generated on Tue Jul 12 2022 17:59:19 by
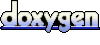