
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
S25FS512.h
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #ifndef S25FS512_H_ 00034 #define S25FS512_H_ 00035 00036 #include "mbed.h" 00037 #include "QuadSpiInterface.h" 00038 00039 00040 #define ADDRESS_INC_4K 0x1000 00041 #define ADDRESS_INC_32K 0x8000 00042 #define ADDRESS_INC_64K 0x10000 00043 00044 #define ADDRESS_4K_START 0x0 00045 #define ADDRESS_4K_END 0x8000 00046 00047 #define ADDRESS_32K_START 0x8000 00048 #define ADDRESS_32k_END 0x10000 00049 00050 #define ADDRESS_64K_START 0x10000 00051 #define ADDRESS_64k_END 0x2000000 00052 00053 #define SIZE_OF_EXTERNAL_FLASH 0x2000000 // 33,554,432 Bytes 00054 #define SIZE_OF_PAGE 0x100 00055 00056 #define IOMUX_IO_ENABLE 1 00057 00058 #define S25FS512_SPI_PORT 1 00059 #define S25FS512_CS_PIN 0 00060 #define S25FS512_CS_POLARITY 0 00061 #define S25FS512_CS_ACTIVITY_DELAY 0 00062 #define S25FS512_CS_INACTIVITY_DELAY 0 00063 #define S25FS512_CLK_HI 4 00064 #define S25FS512_CLK_LOW 4 00065 #define S25FS512_ALT_CLK 0 00066 #define S25FS512_CLK_POLARITY 0 00067 #define S25FS512_CLK_PHASE 0 00068 #define S25FS512_WRITE 1 00069 #define S25FS512_READ 0 00070 00071 ///< Byte addresses for flash configuration 00072 #define Address_CR1NV 0x00000002 00073 #define Address_CR1V 0x00800002 00074 #define Address_CR2NV 0x00000003 00075 #define Address_CR2V 0x00800003 00076 00077 #define INT_PORT_B 3 00078 #define INT_PIN_B 6 00079 00080 00081 class S25FS512 { 00082 public: 00083 00084 ///< @detail S25FS512 Commands 00085 typedef enum{ 00086 Write_Reg = 0x01, 00087 Read_Status_Reg1 = 0x05, 00088 Write_Enable = 0x06, 00089 Erase_4k = 0x20, 00090 Bulk_Erase = 0x60, 00091 Read_Any_Reg = 0x65, 00092 Software_Reset_Enable = 0x66, 00093 Write_Any_Reg = 0x71, 00094 Software_Reset = 0x99, 00095 Read_Id = 0x9F, 00096 Erase_64k_256k = 0xD8, 00097 Quad_IO_Read = 0xEB, 00098 } S25FS512_Commands_t; 00099 00100 S25FS512(QuadSpiInterface *_quadSpiInterface); 00101 ~S25FS512(void); 00102 00103 QuadSpiInterface *quadSpiInterface; 00104 00105 /** @brief Initialize the driver 00106 */ 00107 void init(void); 00108 00109 /** @brief Detect the presence of the flash device 00110 */ 00111 uint8_t detect(void); 00112 00113 /** @brief Read the identification of the flash 00114 */ 00115 int8_t readIdentification(uint8_t *dataArray, uint8_t length); 00116 00117 /** @brief Bulk erase the flash device 00118 */ 00119 int8_t bulkErase_Helper(void); 00120 00121 /** @brief Erase Parameter Sectors 00122 */ 00123 int8_t parameterSectorErase_Helper(uint32_t address); 00124 00125 /** @brief Write a Page 00126 */ 00127 int8_t writePage_Helper(uint32_t pageNumber, uint8_t *buffer, uint32_t offset); 00128 00129 /** @brief Read a Page 00130 */ 00131 int8_t readPages_Helper(uint32_t startPageNumber,uint32_t endPageNumber, uint8_t *buffer, uint32_t offset); 00132 00133 /** @brief Erase a Sector 00134 @param address Address of sector to erase 00135 */ 00136 int8_t sectorErase_Helper(uint32_t address); 00137 00138 /** @brief Scans through byte pointer for a page worth of data to see if the page is all FFs 00139 @param ptr Byte pointer to buffer to scan 00140 @return Returns a 1 if the page is empty, 0 if it is not all FFs 00141 */ 00142 bool isPageEmpty(uint8_t *ptr); 00143 00144 /** @brief Issue a software reset to the flash device 00145 */ 00146 uint8_t reset(void); 00147 00148 /** @brief Enable a hardware reset 00149 */ 00150 uint8_t enableHWReset(void); 00151 00152 /** @brief Read the id byte of this device 00153 */ 00154 void readID(uint8_t *id); 00155 00156 void test_verifyPage0Empty(uint8_t *ptr, int currentPage, int pagesWrittenTo); 00157 00158 int8_t readPartialPage_Helper(uint32_t pageNumber, uint8_t *buffer, uint32_t count); 00159 00160 private: 00161 void disableInterrupt(uint8_t state); 00162 int8_t reg_write_read_multiple_quad_last(uint8_t *dataIn, uint8_t numberIn, uint8_t *dataOut, uint8_t numberOut, uint8_t last); 00163 int8_t reg_write_read_multiple_quad(uint8_t *dataIn, uint8_t numberIn, uint8_t *dataOut, uint8_t numberOut); 00164 int8_t reg_write_read_multiple_4Wire(uint8_t *bufferOut, uint8_t numberOut, uint8_t *bufferIn, uint8_t numberIn); 00165 uint8_t spiWriteRead (uint8_t writeNumber,uint8_t *writeData, uint8_t readNumber, uint8_t *readData); 00166 uint8_t spiWriteRead4Wire(uint8_t writeNumber,uint8_t *writeData, uint8_t readNumber, uint8_t *readData); 00167 int8_t writeAnyRegister(uint32_t address, uint8_t data); 00168 int8_t writeAnyRegister4Wire(uint32_t address, uint8_t data); 00169 int8_t writeRegisters(void); 00170 uint8_t wren(void); 00171 int setQuadMode(void); 00172 int wren4Wire(void); 00173 // int8_t setQuadMode(); 00174 int8_t readAnyRegister(uint32_t address, uint8_t *data, uint32_t length); 00175 int8_t bulkErase(void); 00176 int8_t pageProgram(uint32_t address, uint8_t *buffer); 00177 int8_t quadIoRead_Pages(uint32_t address, uint8_t *buffer, uint32_t numberOfPages); 00178 int8_t checkBusy(void); 00179 void waitTillNotBusy(void); 00180 int8_t sectorErase(uint32_t address); 00181 int8_t parameterSectorErase(uint32_t address); 00182 int8_t quadIoRead_PartialPage(uint32_t address, uint8_t *buffer, uint32_t numberOfBytesInPage); 00183 00184 uint8_t flashBuffer[257 + 10]; 00185 }; 00186 #endif /* S25FS512_H_ */
Generated on Tue Jul 12 2022 17:59:19 by
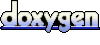