
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
RpcDeclarations.h
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #ifndef _RPCDECLARATIONS_H_ 00034 #define _RPCDECLARATIONS_H_ 00035 00036 /// define the parts of a RPC. ObjectName, MethodName and function 00037 struct RPC_registeredProcedure { 00038 const char *objectName; 00039 const char *methodName; 00040 //enum eArgType argTypes[4]; 00041 int (*func)(char args[32][32], char results[32][32]); 00042 struct RPC_registeredProcedure *next; 00043 }; 00044 00045 /// used to keep track of the head of the list and the end of a list 00046 struct RPC_Object { 00047 struct RPC_registeredProcedure *head; 00048 struct RPC_registeredProcedure *last; 00049 }; 00050 00051 00052 #define I2C_NAME "I2c" 00053 /** 00054 * @brief /I2c/WriteRead instance slaveAddress writeNumber dataToWrite readNumber 00055 * @details Command allows you to write and read generic I2c commands using a given I2c instance master 00056 * @param instance The I2c master on the Nimitz to use 00057 * @param slaveAddress Slave address to use when communicating 00058 * @param writeNumber The number of bytes to write 00059 * @param dataToWrite A series of space separated hex values that are to be written 00060 * @param readNumber The number of bytes to read may be 0 if reading is not needed 00061 * @details Example: /I2c/WriteRead 1 A0 3 11 22 33 2 00062 * @details This performs an I2c write and read using instance 1, slave address A0, and writes 3 bytes, 00063 * @details the 3 bytes that are written are 11 22 33, 2 bytes are meant to be read after the write 00064 */ 00065 struct RPC_registeredProcedure Define_I2c_WriteRead = { I2C_NAME, "WriteRead", I2C_WriteRead }; 00066 00067 //example /I2c/WriteRead 1 A0 3 11 22 33 2 00068 #define System_NAME "System" 00069 00070 /** 00071 * @brief /System/ReadVer 00072 * @details Returns the version string of the FW that is currently running 00073 * @details Example: /System/ReadVer 00074 * @details The command returns a version string similar to this: "HSP FW Version 2.0.1f 8/23/16" 00075 */ 00076 struct RPC_registeredProcedure Define_System_ReadVer = { System_NAME, "ReadVer", System_ReadVer }; 00077 /** 00078 * @brief /System/ReadBuildTime 00079 * @details Returns the build string of the FW that is currently running, this is the time and date that the firmware was built 00080 * @details Example: /System/ReadBuildTime 00081 * @details The command returns a build string similar to this: "Build Time: Fri Jul 1 15:48:31 2016" 00082 */ 00083 struct RPC_registeredProcedure Define_System_ReadBuildTime = { System_NAME, "ReadBuildTime", System_ReadBuildTime }; 00084 00085 #define MAX30101_NAME "MAX30101" //"MAX30101" 00086 /** 00087 * @brief /MAX30101/WriteReg address data 00088 * @details Returns the version string of the FW that is currently running 00089 * @param address Register address to write to within the MAX30101 00090 * @param data The data to write to the MAX30101 00091 * @details Example: /MAX30101/WriteReg 01 123456 00092 */ 00093 struct RPC_registeredProcedure Define_MAX30101_WriteReg = { MAX30101_NAME, "WriteReg", MAX30101_WriteReg }; 00094 /** 00095 * @brief /MAX30101/ReadReg address data 00096 * @details Returns the version string of the FW that is currently running 00097 * @param address Register address to write to within the MAX30101 00098 * @param data The data to write to the MAX30101 00099 * @details Example: /MAX30101/WriteReg 01 123456 00100 */ 00101 struct RPC_registeredProcedure Define_MAX30101_ReadReg = { MAX30101_NAME, "ReadReg", MAX30101_ReadReg }; 00102 /** 00103 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00104 * @details This function sets up for the SpO2 mode. 00105 * @param fifo_waterlevel_mark 00106 * @param sample_avg 00107 * @param sample_rate 00108 * @param pulse_width 00109 * @param red_led_current 00110 * @param ir_led_current 00111 */ 00112 struct RPC_registeredProcedure Define_MAX30101_SpO2mode_Init = { MAX30101_NAME, "SpO2mode_init", MAX30101_SpO2mode_init }; 00113 /** 00114 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00115 * @details This function sets up for the SpO2 mode. 00116 * @param fifo_waterlevel_mark 00117 * @param sample_avg 00118 * @param sample_rate 00119 * @param pulse_width 00120 * @param red_led_current 00121 * @param ir_led_current 00122 */ 00123 struct RPC_registeredProcedure Define_MAX30101_SpO2mode_InitStart = { MAX30101_NAME, "SpO2mode_InitStart", MAX30101_SpO2mode_InitStart }; 00124 /** 00125 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00126 * @details This function sets up for the SpO2 mode. 00127 * @param fifo_waterlevel_mark 00128 * @param sample_avg 00129 * @param sample_rate 00130 * @param pulse_width 00131 * @param red_led_current 00132 * @param ir_led_current 00133 */ 00134 struct RPC_registeredProcedure Define_MAX30101_HRmode_Init = { MAX30101_NAME, "HRmode_init", MAX30101_HRmode_init }; 00135 /** 00136 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00137 * @details This function sets up for the SpO2 mode. 00138 * @param fifo_waterlevel_mark 00139 * @param sample_avg 00140 * @param sample_rate 00141 * @param pulse_width 00142 * @param red_led_current 00143 * @param ir_led_current 00144 */ 00145 struct RPC_registeredProcedure Define_MAX30101_HRmode_InitStart = { MAX30101_NAME, "HRmode_InitStart", MAX30101_HRmode_InitStart }; 00146 /** 00147 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00148 * @details This function sets up for the SpO2 mode. 00149 * @param fifo_waterlevel_mark 00150 * @param sample_avg 00151 * @param sample_rate 00152 * @param pulse_width 00153 * @param red_led_current 00154 * @param ir_led_current 00155 */ 00156 struct RPC_registeredProcedure Define_MAX30101_Multimode_init = { MAX30101_NAME, "Multimode_init", MAX30101_Multimode_init }; 00157 /** 00158 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00159 * @details This function sets up for the SpO2 mode. 00160 * @param fifo_waterlevel_mark 00161 * @param sample_avg 00162 * @param sample_rate 00163 * @param pulse_width 00164 * @param red_led_current 00165 * @param ir_led_current 00166 */ 00167 struct RPC_registeredProcedure Define_MAX30101_Multimode_InitStart = { MAX30101_NAME, "Multimode_InitStart", MAX30101_Multimode_InitStart }; 00168 /** 00169 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00170 * @details This function sets up for the SpO2 mode. 00171 * @param fifo_waterlevel_mark 00172 * @param sample_avg 00173 * @param sample_rate 00174 * @param pulse_width 00175 * @param red_led_current 00176 * @param ir_led_current 00177 */ 00178 struct RPC_registeredProcedure Define_MAX30101_SpO2mode_stop = { MAX30101_NAME, "SpO2mode_stop", MAX30101_SpO2mode_stop }; 00179 /** 00180 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00181 * @details This function sets up for the SpO2 mode. 00182 * @param fifo_waterlevel_mark 00183 * @param sample_avg 00184 * @param sample_rate 00185 * @param pulse_width 00186 * @param red_led_current 00187 * @param ir_led_current 00188 */ 00189 struct RPC_registeredProcedure Define_MAX30101_HRmode_stop = { MAX30101_NAME, "HRmode_stop", MAX30101_HRmode_stop }; 00190 /** 00191 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00192 * @details This function sets up for the SpO2 mode. 00193 * @param fifo_waterlevel_mark 00194 * @param sample_avg 00195 * @param sample_rate 00196 * @param pulse_width 00197 * @param red_led_current 00198 * @param ir_led_current 00199 */ 00200 struct RPC_registeredProcedure Define_MAX30101_Multimode_stop = { MAX30101_NAME, "Multimode_stop", MAX30101_Multimode_stop }; 00201 00202 #define MAX30001_NAME "MAX30001" 00203 #define MAX30003_NAME "MAX30003" 00204 00205 #define MAX31725_NAME "MAX31725" 00206 #define MAX30205_NAME "MAX30205" 00207 00208 /** 00209 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00210 * @details This function sets up for the SpO2 mode. 00211 * @param fifo_waterlevel_mark 00212 * @param sample_avg 00213 * @param sample_rate 00214 * @param pulse_width 00215 * @param red_led_current 00216 * @param ir_led_current 00217 */ 00218 struct RPC_registeredProcedure Define_MAX30001_WriteReg = { MAX30001_NAME, "WriteReg", MAX30001_WriteReg }; 00219 /** 00220 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00221 * @details This function sets up for the SpO2 mode. 00222 * @param fifo_waterlevel_mark 00223 * @param sample_avg 00224 * @param sample_rate 00225 * @param pulse_width 00226 * @param red_led_current 00227 * @param ir_led_current 00228 */ 00229 struct RPC_registeredProcedure Define_MAX30001_ReadReg = { MAX30001_NAME, "ReadReg", MAX30001_ReadReg }; 00230 /** 00231 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00232 * @details This function sets up for the SpO2 mode. 00233 * @param fifo_waterlevel_mark 00234 * @param sample_avg 00235 * @param sample_rate 00236 * @param pulse_width 00237 * @param red_led_current 00238 * @param ir_led_current 00239 */ 00240 struct RPC_registeredProcedure Define_MAX30001_Start = { MAX30001_NAME, "Start", MAX30001_Start }; 00241 /** 00242 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00243 * @details This function sets up for the SpO2 mode. 00244 * @param fifo_waterlevel_mark 00245 * @param sample_avg 00246 * @param sample_rate 00247 * @param pulse_width 00248 * @param red_led_current 00249 * @param ir_led_current 00250 */ 00251 struct RPC_registeredProcedure Define_MAX30001_Stop = { MAX30001_NAME, "Stop", MAX30001_Stop }; 00252 /** 00253 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00254 * @details This function sets up for the SpO2 mode. 00255 * @param fifo_waterlevel_mark 00256 * @param sample_avg 00257 * @param sample_rate 00258 * @param pulse_width 00259 * @param red_led_current 00260 * @param ir_led_current 00261 */ 00262 struct RPC_registeredProcedure Define_MAX30001_Rbias_FMSTR_Init = { MAX30001_NAME, "Rbias_FMSTR_Init", MAX30001_Rbias_FMSTR_Init }; 00263 /** 00264 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00265 * @details This function sets up for the SpO2 mode. 00266 * @param fifo_waterlevel_mark 00267 * @param sample_avg 00268 * @param sample_rate 00269 * @param pulse_width 00270 * @param red_led_current 00271 * @param ir_led_current 00272 */ 00273 struct RPC_registeredProcedure Define_MAX30001_CAL_InitStart = { MAX30001_NAME, "CAL_InitStart", MAX30001_CAL_InitStart }; 00274 /** 00275 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00276 * @details This function sets up for the SpO2 mode. 00277 * @param fifo_waterlevel_mark 00278 * @param sample_avg 00279 * @param sample_rate 00280 * @param pulse_width 00281 * @param red_led_current 00282 * @param ir_led_current 00283 */ 00284 struct RPC_registeredProcedure Define_MAX30001_ECG_InitStart = { MAX30001_NAME, "ECG_InitStart", MAX30001_ECG_InitStart }; 00285 /** 00286 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00287 * @details This function sets up for the SpO2 mode. 00288 * @param fifo_waterlevel_mark 00289 * @param sample_avg 00290 * @param sample_rate 00291 * @param pulse_width 00292 * @param red_led_current 00293 * @param ir_led_current 00294 */ 00295 struct RPC_registeredProcedure Define_MAX30001_ECGFast_Init = { MAX30001_NAME, "ECGFast_Init", MAX30001_ECGFast_Init }; 00296 /** 00297 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00298 * @details This function sets up for the SpO2 mode. 00299 * @param fifo_waterlevel_mark 00300 * @param sample_avg 00301 * @param sample_rate 00302 * @param pulse_width 00303 * @param red_led_current 00304 * @param ir_led_current 00305 */ 00306 struct RPC_registeredProcedure Define_MAX30001_PACE_InitStart = { MAX30001_NAME, "PACE_InitStart", MAX30001_PACE_InitStart }; 00307 /** 00308 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00309 * @details This function sets up for the SpO2 mode. 00310 * @param fifo_waterlevel_mark 00311 * @param sample_avg 00312 * @param sample_rate 00313 * @param pulse_width 00314 * @param red_led_current 00315 * @param ir_led_current 00316 */ 00317 struct RPC_registeredProcedure Define_MAX30001_BIOZ_InitStart = { MAX30001_NAME, "BIOZ_InitStart", MAX30001_BIOZ_InitStart }; 00318 /** 00319 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00320 * @details This function sets up for the SpO2 mode. 00321 * @param fifo_waterlevel_mark 00322 * @param sample_avg 00323 * @param sample_rate 00324 * @param pulse_width 00325 * @param red_led_current 00326 * @param ir_led_current 00327 */ 00328 struct RPC_registeredProcedure Define_MAX30001_RtoR_InitStart = { MAX30001_NAME, "RtoR_InitStart", MAX30001_RtoR_InitStart }; 00329 /** 00330 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00331 * @details This function sets up for the SpO2 mode. 00332 * @param fifo_waterlevel_mark 00333 * @param sample_avg 00334 * @param sample_rate 00335 * @param pulse_width 00336 * @param red_led_current 00337 * @param ir_led_current 00338 */ 00339 struct RPC_registeredProcedure Define_MAX30001_Stop_ECG = { MAX30001_NAME, "Stop_ECG", MAX30001_Stop_ECG }; 00340 /** 00341 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00342 * @details This function sets up for the SpO2 mode. 00343 * @param fifo_waterlevel_mark 00344 * @param sample_avg 00345 * @param sample_rate 00346 * @param pulse_width 00347 * @param red_led_current 00348 * @param ir_led_current 00349 */ 00350 struct RPC_registeredProcedure Define_MAX30001_Stop_PACE = { MAX30001_NAME, "Stop_PACE", MAX30001_Stop_PACE }; 00351 /** 00352 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00353 * @details This function sets up for the SpO2 mode. 00354 * @param fifo_waterlevel_mark 00355 * @param sample_avg 00356 * @param sample_rate 00357 * @param pulse_width 00358 * @param red_led_current 00359 * @param ir_led_current 00360 */ 00361 struct RPC_registeredProcedure Define_MAX30001_Stop_BIOZ = { MAX30001_NAME, "Stop_BIOZ", MAX30001_Stop_BIOZ }; 00362 /** 00363 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00364 * @details This function sets up for the SpO2 mode. 00365 * @param fifo_waterlevel_mark 00366 * @param sample_avg 00367 * @param sample_rate 00368 * @param pulse_width 00369 * @param red_led_current 00370 * @param ir_led_current 00371 */ 00372 struct RPC_registeredProcedure Define_MAX30001_Stop_RtoR = { MAX30001_NAME, "Stop_RtoR", MAX30001_Stop_RtoR }; 00373 /** 00374 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00375 * @details This function sets up for the SpO2 mode. 00376 * @param fifo_waterlevel_mark 00377 * @param sample_avg 00378 * @param sample_rate 00379 * @param pulse_width 00380 * @param red_led_current 00381 * @param ir_led_current 00382 */ 00383 struct RPC_registeredProcedure Define_MAX30001_Stop_Cal = { MAX30001_NAME, "Stop_Cal", MAX30001_Stop_Cal }; 00384 /** 00385 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00386 * @details This function sets up for the SpO2 mode. 00387 * @param fifo_waterlevel_mark 00388 * @param sample_avg 00389 * @param sample_rate 00390 * @param pulse_width 00391 * @param red_led_current 00392 * @param ir_led_current 00393 */ 00394 struct RPC_registeredProcedure Define_MAX30001_Enable_ECG_LeadON = { MAX30001_NAME, "Enable_ECG_LeadON", MAX30001_Enable_ECG_LeadON }; 00395 /** 00396 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00397 * @details This function sets up for the SpO2 mode. 00398 * @param fifo_waterlevel_mark 00399 * @param sample_avg 00400 * @param sample_rate 00401 * @param pulse_width 00402 * @param red_led_current 00403 * @param ir_led_current 00404 */ 00405 struct RPC_registeredProcedure Define_MAX30001_Enable_BIOZ_LeadON = { MAX30001_NAME, "Enable_BIOZ_LeadON", MAX30001_Enable_BIOZ_LeadON }; 00406 /** 00407 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00408 * @details This function sets up for the SpO2 mode. 00409 * @param fifo_waterlevel_mark 00410 * @param sample_avg 00411 * @param sample_rate 00412 * @param pulse_width 00413 * @param red_led_current 00414 * @param ir_led_current 00415 */ 00416 struct RPC_registeredProcedure Define_MAX30001_Read_LeadON = { MAX30001_NAME, "Read_LeadON", MAX30001_Read_LeadON }; 00417 /** 00418 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00419 * @details This function sets up for the SpO2 mode. 00420 * @param fifo_waterlevel_mark 00421 * @param sample_avg 00422 * @param sample_rate 00423 * @param pulse_width 00424 * @param red_led_current 00425 * @param ir_led_current 00426 */ 00427 struct RPC_registeredProcedure Define_MAX30001_StartTest = { MAX30001_NAME, "StartTest", MAX30001_StartTest }; 00428 /** 00429 * @brief /MAX30101/SpO2mode_init fifo_waterlevel_mark sample_avg sample_rate pulse_width red_led_current ir_led_current 00430 * @details This function sets up for the SpO2 mode. 00431 * @param fifo_waterlevel_mark 00432 * @param sample_avg 00433 * @param sample_rate 00434 * @param pulse_width 00435 * @param red_led_current 00436 * @param ir_led_current 00437 */ 00438 struct RPC_registeredProcedure Define_MAX30001_INT_assignment = { MAX30001_NAME, "INT_assignment", MAX30001_INT_assignment }; 00439 00440 #define LOGGING_NAME "Logging" 00441 /** 00442 * @brief /Logging/StartMissionDefine 00443 * @details A command to send when you are starting to define a mission 00444 */ 00445 struct RPC_registeredProcedure Define_Logging_StartMissionDefine = { LOGGING_NAME, "StartMissionDefine", Logging_RPC_StartMissionDefine }; 00446 /** 00447 * @brief /Logging/AppendMissionCmd missionString 00448 * @details Specify a RPC command that is part of a mission 00449 */ 00450 struct RPC_registeredProcedure Define_Logging_AppendMissionCmd = { LOGGING_NAME, "AppendMissionCmd", Logging_RPC_AppendMissionCmd }; 00451 /** 00452 * @brief /Logging/EndMissionDefine 00453 * @details RPC command that indicated the end of defining a mission 00454 */ 00455 struct RPC_registeredProcedure Define_Logging_EndMissionDefine = { LOGGING_NAME, "EndMissionDefine", Logging_RPC_EndMissionDefine }; 00456 /** 00457 * @brief /Logging/WriteMission 00458 * @details Write the described mission to flash 00459 */ 00460 struct RPC_registeredProcedure Define_Logging_WriteMission = { LOGGING_NAME, "WriteMission", Logging_RPC_WriteMission }; 00461 /** 00462 * @brief /Logging/ReadMission 00463 * @details Read the mission from flash 00464 */ 00465 struct RPC_registeredProcedure Define_Logging_ReadMission = { LOGGING_NAME, "ReadMission", Logging_RPC_ReadMission }; 00466 /** 00467 * @brief /Logging/EraseMission 00468 * @details Erase the mission in flash 00469 */ 00470 struct RPC_registeredProcedure Define_Logging_EraseMission = { LOGGING_NAME, "EraseMission", Logging_RPC_EraseMission }; 00471 /** 00472 * @brief /Logging/EraseWrittenSectors 00473 * @details Erase the datalog in flash, this erases all of the datalog that has been written to the flash 00474 */ 00475 struct RPC_registeredProcedure Define_Logging_EraseWrittenSectors = { LOGGING_NAME, "EraseWrittenSectors", Logging_EraseWrittenSectors }; 00476 /** 00477 * @brief /Logging/StartLoggingUsb 00478 * @details Start streaming data through USB 00479 */ 00480 struct RPC_registeredProcedure Define_Logging_StartLoggingUsb = { LOGGING_NAME, "StartLoggingUsb", Logging_StartLoggingUsb }; 00481 /** 00482 * @brief /Logging/StartLoggingFlash 00483 * @details Start logging data to flash 00484 */ 00485 struct RPC_registeredProcedure Define_Logging_StartLoggingFlash = { LOGGING_NAME, "StartLoggingFlash", Logging_StartLoggingFlash }; 00486 /** 00487 * @brief /Logging/GetLastWrittenPage 00488 * @details Returns the last page that has been written to flash, this call searchs until it finds an empty flash page 00489 */ 00490 struct RPC_registeredProcedure Define_Logging_GetLastWrittenPage = { LOGGING_NAME, "GetLastWrittenPage", Logging_GetLastWrittenPage }; 00491 /** 00492 * @brief /Logging/Start 00493 * @details Starts a datalogging session into flash, allows the ability to start datalogging via RPC call 00494 */ 00495 struct RPC_registeredProcedure Define_Logging_Start = { LOGGING_NAME, "Start", Logging_Start }; 00496 00497 #define LIS2DH_NAME "LIS2DH" 00498 /** 00499 * @brief /LIS2DH/InitStart 00500 * @details Starts streaming interrupts from the LIS2DH device 00501 */ 00502 struct RPC_registeredProcedure Define_LIS2DH_InitStart = { LIS2DH_NAME, "InitStart", LIS2DH_InitStart }; 00503 /** 00504 * @brief /LIS2DH/ReadReg address 00505 * @details Reads a register 00506 * @param address Register address to read from 00507 */ 00508 struct RPC_registeredProcedure Define_LIS2DH_ReadReg = { LIS2DH_NAME, "ReadReg", LIS2DH_ReadReg }; 00509 /** 00510 * @brief /LIS2DH/WriteReg address data 00511 * @details Write a register 00512 * @param address Register address to read from 00513 * @param data Data to write 00514 */ 00515 struct RPC_registeredProcedure Define_LIS2DH_WriteReg = { LIS2DH_NAME, "WriteReg", LIS2DH_WriteReg }; 00516 /** 00517 * @brief /LIS2DH/Stop 00518 * @details Stop the interrupts within the LIS2DH 00519 */ 00520 struct RPC_registeredProcedure Define_LIS2DH_Stop = { LIS2DH_NAME, "Stop", LIS2DH_Stop }; 00521 00522 #define BMP280_NAME "BMP280" 00523 /** 00524 * @brief /BMP280/InitStart 00525 * @details Start the polling process for the BMP280 00526 */ 00527 struct RPC_registeredProcedure Define_BMP280_InitStart = { BMP280_NAME, "InitStart", BMP280_InitStart }; 00528 00529 #define MAX30205_1_NAME "MAX30205_1" 00530 #define MAX31725_1_NAME "MAX31725_1" 00531 /** 00532 * @brief /MAX30205_1/InitStart 00533 * @details Start the polling process for the MAX30205 instance 1 00534 */ 00535 struct RPC_registeredProcedure Define_MAX30205_1_InitStart = { MAX30205_1_NAME, "InitStart", MAX30205_1_InitStart }; 00536 struct RPC_registeredProcedure Define_MAX31725_1_InitStart = { MAX31725_1_NAME, "InitStart", MAX30205_1_InitStart }; 00537 00538 #define MAX30205_2_NAME "MAX30205_2" 00539 #define MAX31725_2_NAME "MAX31725_2" 00540 /** 00541 * @brief /MAX30205_2/InitStart 00542 * @details Start the polling process for the MAX30205 instance 2 00543 */ 00544 struct RPC_registeredProcedure Define_MAX30205_2_InitStart = { MAX30205_2_NAME, "InitStart", MAX30205_2_InitStart }; 00545 struct RPC_registeredProcedure Define_MAX31725_2_InitStart = { MAX31725_2_NAME, "InitStart", MAX30205_2_InitStart }; 00546 00547 #define LED_NAME "Led" 00548 /** 00549 * @brief /Led/On 00550 * @details Turn on the HSP onboard LED 00551 */ 00552 struct RPC_registeredProcedure Define_Led_On = { LED_NAME, "On", Led_On }; 00553 /** 00554 * @brief /Led/Off 00555 * @details Turn off the HSP onboard LED 00556 */ 00557 struct RPC_registeredProcedure Define_Led_Off = { LED_NAME, "Off", Led_Off }; 00558 /** 00559 * @brief /Led/Blink mS 00560 * @details Start blinking the HSP onboard LED 00561 * @param mS Blink using a mS period 00562 */ 00563 struct RPC_registeredProcedure Define_Led_BlinkHz = { LED_NAME, "Blink", Led_BlinkHz }; 00564 /** 00565 * @brief /Led/Pattern pattern 00566 * @details Rotate a 32-bit pattern through the LED so that specific blink patterns can be obtained 00567 * @param pattern A 32-bit pattern to rotate through 00568 */ 00569 struct RPC_registeredProcedure Define_Led_BlinkPattern = { LED_NAME, "Pattern", Led_BlinkPattern }; 00570 00571 #define S25FS512_NAME "S25FS512" 00572 /** 00573 * @brief /S25FS512/ReadId 00574 * @details Rotate a 32-bit pattern through the LED so that specific blink patterns can be obtained 00575 * @param pattern A 32-bit pattern to rotate through 00576 */ 00577 struct RPC_registeredProcedure Define_S25FS512_ReadId = { S25FS512_NAME, "ReadId", S25FS512_ReadId }; 00578 /** 00579 * @brief /S25FS512/ReadPagesBinary startPage endPage 00580 * @details Read a page from flash, return the data in binary (non-ascii) 00581 * @param startPage The Starting page to read from 00582 * @param endPage The last page to read from 00583 */ 00584 struct RPC_registeredProcedure Define_S25FS512_ReadPagesBinary = { S25FS512_NAME, "ReadPagesBinary", S25FS512_ReadPagesBinary }; 00585 /** 00586 * @brief /S25FS512/Reset 00587 * @details Issue a soft reset to the flash device 00588 */ 00589 struct RPC_registeredProcedure Define_S25FS512_Reset = { S25FS512_NAME, "Reset", S25FS512_Reset }; 00590 /** 00591 * @brief /S25FS512/EnableHWReset 00592 * @details Enable HW resets to the device 00593 */ 00594 struct RPC_registeredProcedure Define_S25FS512_EnableHWReset = { S25FS512_NAME, "EnableHWReset", S25FS512_EnableHWReset }; 00595 /** 00596 * @brief /S25FS512/SpiWriteRead 00597 * @details Write and read SPI to the flash device using Quad SPI 00598 */ 00599 struct RPC_registeredProcedure Define_S25FS512_SpiWriteRead = { S25FS512_NAME, "SpiWriteRead", S25FS512_SpiWriteRead }; 00600 /** 00601 * @brief /S25FS512/SpiWriteRead4Wire 00602 * @details Write and read SPI to the flash device using 4 wire 00603 */ 00604 struct RPC_registeredProcedure Define_S25FS512_SpiWriteRead4Wire = { S25FS512_NAME, "SpiWriteRead4Wire", S25FS512_SpiWriteRead4Wire }; 00605 00606 #define TESTING_NAME "Testing" 00607 /** 00608 * @brief /Testing/Test_S25FS512 00609 * @details Start a testing sequence for this device, returns PASS and FAIL strings and detailed results of the test 00610 */ 00611 struct RPC_registeredProcedure Define_Testing_Test_S25FS512 = { TESTING_NAME, "Test_S25FS512", Test_S25FS512}; 00612 /** 00613 * @brief /Testing/Test_BMP280 00614 * @details Start a testing sequence for this device, returns PASS and FAIL strings and detailed results of the test 00615 */ 00616 struct RPC_registeredProcedure Define_Testing_Test_BMP280 = { TESTING_NAME, "Test_BMP280", Test_BMP280}; 00617 /** 00618 * @brief /Testing/Test_LIS2DH 00619 * @details Start a testing sequence for this device, returns PASS and FAIL strings and detailed results of the test 00620 */ 00621 struct RPC_registeredProcedure Define_Testing_Test_LIS2DH = { TESTING_NAME, "Test_LIS2DH", Test_LIS2DH }; 00622 /** 00623 * @brief /Testing/Test_LSM6DS3 00624 * @details Start a testing sequence for this device, returns PASS and FAIL strings and detailed results of the test 00625 */ 00626 struct RPC_registeredProcedure Define_Testing_Test_LSM6DS3 = { TESTING_NAME, "Test_LSM6DS3", Test_LSM6DS3 }; 00627 /** 00628 * @brief /Testing/Test_MAX30205_1 00629 * @details Start a testing sequence for this device, returns PASS and FAIL strings and detailed results of the test 00630 */ 00631 struct RPC_registeredProcedure Define_Testing_Test_MAX30205_1 = { TESTING_NAME, "Test_MAX30205_1", Test_MAX30205_1 }; 00632 /** 00633 * @brief /Testing/Test_MAX30205_2 00634 * @details Start a testing sequence for this device, returns PASS and FAIL strings and detailed results of the test 00635 */ 00636 struct RPC_registeredProcedure Define_Testing_Test_MAX30205_2 = { TESTING_NAME, "Test_MAX30205_2", Test_MAX30205_2 }; 00637 /** 00638 * @brief /Testing/Test_MAX30101 00639 * @details Start a testing sequence for this device, returns PASS and FAIL strings and detailed results of the test 00640 */ 00641 struct RPC_registeredProcedure Define_Testing_Test_MAX30101 = { TESTING_NAME, "Test_MAX30101", Test_MAX30101 }; 00642 /** 00643 * @brief /Testing/Test_MAX30001 00644 * @details Start a testing sequence for this device, returns PASS and FAIL strings and detailed results of the test 00645 */ 00646 struct RPC_registeredProcedure Define_Testing_Test_MAX30001 = { TESTING_NAME, "Test_MAX30001", Test_MAX30001 }; 00647 /** 00648 * @brief /Testing/Test_EM9301 00649 * @details Start a testing sequence for this device, returns PASS and FAIL strings and detailed results of the test 00650 */ 00651 struct RPC_registeredProcedure Define_Testing_Test_EM9301 = { TESTING_NAME, "Test_EM9301", Test_EM9301 }; 00652 /** 00653 * @brief /Testing/Test_EM9301 00654 * @details Start a testing sequence for this device, returns PASS and FAIL strings and detailed results of the test 00655 */ 00656 //struct RPC_registeredProcedure Define_Testing_Test_SensorReadings = { TESTING_NAME, "Test_SensorReadings", Test_EM9301 }; 00657 00658 #define MAX14720_NAME "MAX14720" 00659 /** 00660 * @brief /MAX14720/ReadBoostVSet 00661 * @details Read the BoostVSet value 00662 * @return Returns the value from the BoostVSet register of the device 00663 */ 00664 struct RPC_registeredProcedure Define_MAX14720_ReadBoostVSet = { MAX14720_NAME, "ReadBoostVSet", MAX14720_ReadBoostVSet }; 00665 /** 00666 * @brief /MAX14720/WriteBoostVSet 00667 * @details Write the BoostVSet value 00668 * @param value The binary value to set the BoostVSet register to 00669 * @return Returns 0x80 on success of the command 00670 */ 00671 struct RPC_registeredProcedure Define_MAX14720_WriteBoostVSet = { MAX14720_NAME, "WriteBoostVSet", MAX14720_WriteBoostVSet }; 00672 /** 00673 * @brief /MAX14720/ReadReg 00674 * @details Read one of the MAX14720 registers 00675 * @param address Address of the register to read 00676 * @return Returns the value from the addressed register from the device 00677 */ 00678 struct RPC_registeredProcedure Define_MAX14720_ReadReg = { MAX14720_NAME, "ReadReg", MAX14720_ReadReg }; 00679 /** 00680 * @brief /MAX14720/WriteReg 00681 * @details Write one of the MAX14720 registers 00682 * @param address Address of the register to write 00683 * @param data Value of the data to write 00684 * @return Returns 0x80 on success of the command 00685 */ 00686 struct RPC_registeredProcedure Define_MAX14720_WriteReg = { MAX14720_NAME, "WriteReg", MAX14720_WriteReg }; 00687 00688 #endif /* _RPCDECLARATIONS_H_ */
Generated on Tue Jul 12 2022 17:59:19 by
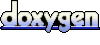