
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
MAX30001_helper.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include "MAX30001_helper.h" 00035 #include "MAX30001.h" 00036 #include "StringInOut.h" 00037 #include "Peripherals.h" 00038 00039 static uint8_t flags[4]; 00040 00041 int MAX30001_Helper_IsStreaming(eFlags flag) { 00042 return flags[(uint32_t)flag]; 00043 } 00044 00045 void MAX30001_Helper_SetStreamingFlag(eFlags flag, uint8_t state) { 00046 flags[(uint32_t)flag] = state; 00047 } 00048 00049 void MAX30001_Helper_Stop(void) { 00050 if (flags[(uint32_t)eStreaming_ECG] == 1) { 00051 Peripherals::max30001()->Stop_ECG(); 00052 } 00053 if (flags[(uint32_t)eStreaming_PACE] == 1) { 00054 Peripherals::max30001()->Stop_PACE(); 00055 } 00056 if (flags[(uint32_t)eStreaming_BIOZ] == 1) { 00057 Peripherals::max30001()->Stop_BIOZ(); 00058 } 00059 if (flags[(uint32_t)eStreaming_RtoR] == 1) { 00060 Peripherals::max30001()->Stop_RtoR(); 00061 } 00062 MAX30001_Helper_ClearStreamingFlags(); 00063 } 00064 00065 int MAX30001_AnyStreamingSet(void) { 00066 uint32_t i; 00067 for (i = 0; i < 4; i++) { 00068 if (flags[i] == 1) return 1; 00069 } 00070 return 0; 00071 } 00072 00073 void MAX30001_Helper_StartSync(void) { 00074 if (MAX30001_AnyStreamingSet() == 1) { 00075 Peripherals::max30001()->synch(); 00076 } 00077 } 00078 00079 void MAX30001_Helper_ClearStreamingFlags(void) { 00080 uint32_t i; 00081 for (i = 0; i < 4; i++) { 00082 flags[i] = 0; 00083 } 00084 } 00085 00086 void MAX30001_Helper_Debug_ShowStreamFlags(void) { 00087 putStr("\r\n"); 00088 if (flags[(uint32_t)eStreaming_ECG] == 1) { 00089 putStr("eStreaming_ECG, "); 00090 } 00091 if (flags[(uint32_t)eStreaming_PACE] == 1) { 00092 putStr("eStreaming_PACE, "); 00093 } 00094 if (flags[(uint32_t)eStreaming_BIOZ] == 1) { 00095 putStr("eStreaming_BIOZ, "); 00096 } 00097 if (flags[(uint32_t)eStreaming_RtoR] == 1) { 00098 putStr("eStreaming_RtoR, "); 00099 } 00100 putStr("\r\n"); 00101 } 00102 00103 void MAX30001_Helper_SetupInterrupts() { 00104 Peripherals::max30001()->INT_assignment(MAX30001::MAX30001_INT_B, MAX30001::MAX30001_NO_INT, MAX30001::MAX30001_NO_INT, ///< en_enint_loc, en_eovf_loc, en_fstint_loc, 00105 MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_NO_INT, ///< en_dcloffint_loc, en_bint_loc, en_bovf_loc, 00106 MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_NO_INT, ///< en_bover_loc, en_bundr_loc, en_bcgmon_loc, 00107 MAX30001::MAX30001_INT_B, MAX30001::MAX30001_NO_INT, MAX30001::MAX30001_NO_INT, ///< en_pint_loc, en_povf_loc, en_pedge_loc, 00108 MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_INT_B, MAX30001::MAX30001_NO_INT, ///< en_lonint_loc, en_rrint_loc, en_samp_loc, 00109 MAX30001::MAX30001_INT_ODNR, MAX30001::MAX30001_INT_ODNR); ///< intb_Type, int2b_Type) 00110 } 00111 00112 00113 00114 static uint8_t serialNumber[6]; 00115 uint8_t *MAX30001_Helper_getVersion(void) { 00116 // read the id 00117 Peripherals::max30001()->reg_read(MAX30001::INFO, (uint32_t *)serialNumber); 00118 // read id twice because it needs to be read twice 00119 Peripherals::max30001()->reg_read(MAX30001::INFO, (uint32_t *)serialNumber); 00120 return serialNumber; 00121 }
Generated on Tue Jul 12 2022 17:59:19 by
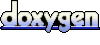