
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
MAX30001_RPC.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include <stdio.h> 00035 #include "USBSerial.h" 00036 #include "StringHelper.h" 00037 #include "MAX30001.h" 00038 #include "Streaming.h" 00039 #include "StringInOut.h" 00040 #include "MAX30001_helper.h" 00041 #include "RpcFifo.h" 00042 #include "RpcServer.h" 00043 #include "Peripherals.h" 00044 #include "DataLoggingService.h" 00045 00046 extern int highDataRate; 00047 extern USBSerial *usbSerialPtr; 00048 00049 uint32_t max30001_RegRead(MAX30001::MAX30001_REG_map_t addr) { 00050 uint32_t data; 00051 Peripherals::max30001()->reg_read(addr, &data); 00052 return data; 00053 } 00054 00055 //****************************************************************************** 00056 void max30001_RegWrite(MAX30001::MAX30001_REG_map_t addr, uint32_t data) { 00057 Peripherals::max30001()->reg_write(addr, data); 00058 } 00059 00060 int MAX30001_WriteReg(char argStrs[32][32], char replyStrs[32][32]) { 00061 uint32_t args[2]; 00062 uint32_t reply[1]; 00063 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00064 00065 max30001_RegWrite((MAX30001::MAX30001_REG_map_t )args[0], args[1]); 00066 reply[0] = 0x80; 00067 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00068 return 0; 00069 } 00070 00071 //****************************************************************************** 00072 int MAX30001_ReadReg(char argStrs[32][32], char replyStrs[32][32]) { 00073 uint32_t args[1]; 00074 uint32_t reply[1]; 00075 uint32_t value; 00076 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00077 value = max30001_RegRead((MAX30001::MAX30001_REG_map_t )args[0]); 00078 reply[0] = value; 00079 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00080 return 0; 00081 } 00082 00083 //****************************************************************************** 00084 int MAX30001_Rbias_FMSTR_Init(char argStrs[32][32], char replyStrs[32][32]) { 00085 uint32_t args[5]; 00086 uint32_t reply[1]; 00087 uint32_t value; 00088 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00089 00090 value = Peripherals::max30001()->Rbias_FMSTR_Init(args[0], ///< En_rbias 00091 args[1], ///< Rbiasv 00092 args[2], ///< Rbiasp 00093 args[3], ///< Rbiasn 00094 args[4]); ///< Fmstr 00095 00096 reply[0] = value; 00097 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00098 return 0; 00099 } 00100 00101 //****************************************************************************** 00102 int MAX30001_CAL_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00103 uint32_t args[6]; 00104 uint32_t reply[1]; 00105 uint32_t value; 00106 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00107 00108 // Peripherals::serial()->printf("MAX30001_CAL_InitStart 0 "); 00109 value = Peripherals::max30001()->CAL_InitStart(args[0], ///< En_Vcal 00110 args[1], ///< Vmag 00111 args[2], ///< Fcal 00112 args[3], ///< Thigh 00113 args[4], ///< Fifty 00114 args[5]); ///< Vmode 00115 00116 reply[0] = value; 00117 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00118 return 0; 00119 } 00120 00121 //****************************************************************************** 00122 int MAX30001_ECG_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00123 uint32_t args[11]; 00124 uint32_t reply[1]; 00125 uint32_t value; 00126 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00127 00128 value = Peripherals::max30001()->ECG_InitStart(args[0], ///< En_ecg 00129 args[1], ///< Openp 00130 args[2], ///< Openn 00131 args[3], ///< Pol 00132 args[4], ///< Calp_sel 00133 args[5], ///< Caln_sel 00134 args[6], ///< E_fit 00135 args[7], ///< Rate 00136 args[8], ///< Gain 00137 args[9], ///< Dhpf 00138 args[10]); ///< Dlpf 00139 00140 MAX30001_Helper_SetStreamingFlag(eStreaming_ECG, 1); 00141 reply[0] = value; 00142 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00143 return 0; 00144 } 00145 00146 //****************************************************************************** 00147 int MAX30001_ECGFast_Init(char argStrs[32][32], char replyStrs[32][32]) { 00148 uint32_t args[3]; 00149 uint32_t reply[1]; 00150 uint32_t value; 00151 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00152 00153 value = Peripherals::max30001()->ECGFast_Init(args[0], ///< Clr_Fast 00154 args[1], ///< Fast 00155 args[2]); ///< Fast_Th 00156 00157 reply[0] = value; 00158 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00159 return 0; 00160 } 00161 00162 //****************************************************************************** 00163 int MAX30001_PACE_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00164 uint32_t args[9]; 00165 uint32_t reply[1]; 00166 uint32_t value; 00167 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00168 00169 value = Peripherals::max30001()->PACE_InitStart(args[0], ///< En_pace 00170 args[1], ///< Clr_pedge 00171 args[2], ///< Pol 00172 args[3], ///< Gn_diff_off 00173 args[4], ///< Gain 00174 args[5], ///< Aout_lbw 00175 args[6], ///< Aout 00176 args[7], ///< Dacp 00177 args[8]); ///< Dacn 00178 00179 00180 MAX30001_Helper_SetStreamingFlag(eStreaming_PACE, 1); 00181 reply[0] = value; 00182 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00183 return 0; 00184 } 00185 00186 //****************************************************************************** 00187 int MAX30001_BIOZ_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00188 uint32_t args[17]; 00189 uint32_t reply[1]; 00190 uint32_t value; 00191 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00192 00193 value = Peripherals::max30001()->BIOZ_InitStart(args[0], ///< En_bioz 00194 args[1], ///< Openp 00195 args[2], ///< Openn 00196 args[3], ///< Calp_sel 00197 args[4], ///< Caln_sel 00198 args[5], ///< CG_mode 00199 args[6], ///< B_fit 00200 args[7], ///< Rate 00201 args[8], ///< Ahpf 00202 args[9], ///< Ext_rbias 00203 args[10], ///< Gain 00204 args[11], ///< Dhpf 00205 args[12], ///< Dlpf 00206 args[13], ///< Fcgen 00207 args[14], ///< Cgmon 00208 args[15], ///< Cgmag 00209 args[16]); ///< Phoff 00210 00211 MAX30001_Helper_SetStreamingFlag(eStreaming_BIOZ, 1); 00212 reply[0] = value; 00213 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00214 return 0; 00215 } 00216 00217 //****************************************************************************** 00218 int MAX30001_RtoR_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00219 uint32_t args[9]; 00220 uint32_t reply[1]; 00221 uint32_t value; 00222 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00223 00224 value = Peripherals::max30001()->RtoR_InitStart(args[0], ///< En_rtor 00225 args[1], ///< Wndw 00226 args[2], ///< Gain 00227 args[3], ///< Pavg 00228 args[4], ///< Ptsf 00229 args[5], ///< Hoff 00230 args[6], ///< Ravg 00231 args[7], ///< Rhsf 00232 args[8]); ///< Clr_rrint 00233 00234 MAX30001_Helper_SetStreamingFlag(eStreaming_RtoR, 1); 00235 reply[0] = value; 00236 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00237 return 0; 00238 } 00239 00240 //****************************************************************************** 00241 int MAX30001_Stop_ECG(char argStrs[32][32], char replyStrs[32][32]) { 00242 uint32_t reply[1]; 00243 Peripherals::max30001()->Stop_ECG(); 00244 reply[0] = 0x80; 00245 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00246 return 0; 00247 } 00248 00249 //****************************************************************************** 00250 int MAX30001_Stop_PACE(char argStrs[32][32], char replyStrs[32][32]) { 00251 uint32_t reply[1]; 00252 Peripherals::max30001()->Stop_PACE(); 00253 reply[0] = 0x80; 00254 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00255 return 0; 00256 } 00257 00258 //****************************************************************************** 00259 int MAX30001_Stop_BIOZ(char argStrs[32][32], char replyStrs[32][32]) { 00260 uint32_t reply[1]; 00261 Peripherals::max30001()->Stop_BIOZ(); 00262 reply[0] = 0x80; 00263 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00264 return 0; 00265 } 00266 00267 //****************************************************************************** 00268 int MAX30001_Stop_RtoR(char argStrs[32][32], char replyStrs[32][32]) { 00269 uint32_t reply[1]; 00270 Peripherals::max30001()->Stop_RtoR(); 00271 reply[0] = 0x80; 00272 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00273 return 0; 00274 } 00275 00276 //****************************************************************************** 00277 int MAX30001_Stop_Cal(char argStrs[32][32], char replyStrs[32][32]) { 00278 00279 uint32_t reply[1]; 00280 00281 reply[0] = 0x80; 00282 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00283 return 0; 00284 } 00285 00286 //****************************************************************************** 00287 void max30001_ServiceStreaming() { 00288 //char ch; 00289 uint32_t val; 00290 00291 fifo_clear(GetStreamOutFifo()); 00292 00293 SetStreaming(TRUE); 00294 clearOutReadFifo(); 00295 while (IsStreaming() == TRUE) { 00296 00297 if (fifo_empty(GetStreamOutFifo()) == 0) { 00298 fifo_get32(GetStreamOutFifo(), &val); 00299 00300 usbSerialPtr->printf("%02X ", val); 00301 00302 } 00303 if (usbSerialPtr->available()) { 00304 usbSerialPtr->_getc(); 00305 00306 MAX30001_Helper_Stop(); 00307 SetStreaming(FALSE); 00308 fifo_clear(GetUSBIncomingFifo()); ///< clear USB serial incoming fifo 00309 fifo_clear(GetStreamOutFifo()); 00310 } 00311 00312 } 00313 } 00314 00315 //****************************************************************************** 00316 int MAX30001_Start(char argStrs[32][32], char replyStrs[32][32]) { 00317 uint32_t reply[1]; 00318 uint32_t all; 00319 fifo_clear(GetUSBIncomingFifo()); 00320 Peripherals::max30001()->synch(); 00321 ///< max30001_ServiceStreaming(); 00322 highDataRate = 0; 00323 Peripherals::max30001()->reg_read(MAX30001::STATUS, &all); 00324 LoggingService_StartLoggingUsb(); 00325 00326 reply[0] = 0x80; 00327 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00328 return 0; 00329 } 00330 00331 //****************************************************************************** 00332 int MAX30001_Stop(char argStrs[32][32], char replyStrs[32][32]) { 00333 /* uint32_t args[1]; 00334 uint32_t reply[1]; 00335 uint32_t value; 00336 //ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00337 max30001_StopTest(); 00338 reply[0] = 0x80; 00339 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs);*/ 00340 return 0; 00341 } 00342 00343 //****************************************************************************** 00344 int MAX30001_INT_assignment(char argStrs[32][32], char replyStrs[32][32]) { 00345 uint32_t args[17]; 00346 uint32_t reply[1]; 00347 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00348 00349 Peripherals::max30001()->INT_assignment( 00350 (MAX30001::max30001_intrpt_Location_t )args[0], 00351 (MAX30001::max30001_intrpt_Location_t )args[1], 00352 (MAX30001::max30001_intrpt_Location_t )args[2], 00353 (MAX30001::max30001_intrpt_Location_t )args[3], 00354 (MAX30001::max30001_intrpt_Location_t )args[4], 00355 (MAX30001::max30001_intrpt_Location_t )args[5], 00356 (MAX30001::max30001_intrpt_Location_t )args[6], 00357 (MAX30001::max30001_intrpt_Location_t )args[7], 00358 (MAX30001::max30001_intrpt_Location_t )args[8], 00359 (MAX30001::max30001_intrpt_Location_t )args[9], 00360 (MAX30001::max30001_intrpt_Location_t )args[10], 00361 (MAX30001::max30001_intrpt_Location_t )args[11], 00362 (MAX30001::max30001_intrpt_Location_t )args[12], 00363 (MAX30001::max30001_intrpt_Location_t )args[13], 00364 (MAX30001::max30001_intrpt_Location_t )args[14], 00365 (MAX30001::max30001_intrpt_type_t)args[15], 00366 (MAX30001::max30001_intrpt_type_t)args[16]); 00367 reply[0] = 0x80; 00368 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00369 return 0; 00370 } 00371 00372 //****************************************************************************** 00373 int MAX30001_StartTest(char argStrs[32][32], char replyStrs[32][32]) { 00374 uint32_t reply[1]; 00375 // ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00376 00377 /*** Set FMSTR over here ****/ 00378 00379 /*** Set and Start the VCAL input ***/ 00380 /* NOTE VCAL must be set first if VCAL is to be used */ 00381 Peripherals::max30001()->CAL_InitStart(0b1, 0b1, 0b1, 0b011, 0x7FF, 0b0); 00382 00383 /**** ECG Initialization ****/ 00384 Peripherals::max30001()->ECG_InitStart(0b1, 0b1, 0b1, 0b0, 0b10, 0b11, 31, 0b00, 0b00, 0b0, 0b01); 00385 00386 /***** PACE Initialization ***/ 00387 Peripherals::max30001()->PACE_InitStart(0b1, 0b0, 0b0, 0b1, 0b000, 0b0, 0b00, 0b0, 0b0); 00388 00389 /**** BIOZ Initialization ****/ 00390 Peripherals::max30001()->BIOZ_InitStart( 00391 0b1, 0b1, 0b1, 0b10, 0b11, 0b00, 7, 0b0, 0b111, 0b0, 0b10, 0b00, 0b00, 0b0001, 0b0, 0b111, 0b0000); 00392 00393 /*** Set RtoR registers ***/ 00394 Peripherals::max30001()->RtoR_InitStart( 00395 0b1, 0b0011, 0b1111, 0b00, 0b0011, 0b000001, 0b00, 0b000, 0b01); 00396 00397 /*** Set Rbias & FMSTR over here ****/ 00398 Peripherals::max30001()->Rbias_FMSTR_Init(0b01, 0b10, 0b1, 0b1, 0b00); 00399 00400 /**** Interrupt Setting ****/ 00401 00402 /*** Set ECG Lead ON/OFF ***/ 00403 // max30001_ECG_LeadOnOff(); 00404 00405 /*** Set BIOZ Lead ON/OFF ***/ 00406 // max30001_BIOZ_LeadOnOff(); Does not work yet... 00407 00408 /**** Do a Synch ****/ 00409 Peripherals::max30001()->synch(); 00410 00411 fifo_clear(GetUSBIncomingFifo()); 00412 max30001_ServiceStreaming(); 00413 00414 reply[0] = 0x80; 00415 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00416 return 0; 00417 } 00418 00419 //****************************************************************************** 00420 int MAX30001_Enable_ECG_LeadON(char argStrs[32][32], char replyStrs[32][32]) { 00421 uint32_t reply[1]; 00422 ///< switch to ECG DC Lead ON 00423 Peripherals::max30001()->Enable_LeadON(0b01); 00424 reply[0] = 0x80; 00425 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00426 return 0; 00427 } 00428 00429 //****************************************************************************** 00430 int MAX30001_Enable_BIOZ_LeadON(char argStrs[32][32], char replyStrs[32][32]) { 00431 uint32_t reply[1]; 00432 ///< switch to BIOZ DC Lead ON 00433 Peripherals::max30001()->Enable_LeadON(0b10); 00434 reply[0] = 0x80; 00435 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00436 return 0; 00437 } 00438 00439 //****************************************************************************** 00440 int MAX30001_Read_LeadON(char argStrs[32][32], char replyStrs[32][32]) { 00441 uint32_t reply[1]; 00442 ///< return the max30001_LeadOn var from the MAX30001 driver 00443 reply[0] = Peripherals::max30001()->max30001_LeadOn; 00444 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00445 return 0; 00446 }
Generated on Tue Jul 12 2022 17:59:19 by
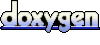