
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
MAX30001.h
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 /** 00033 * 00034 * Maxim Integrated MAX30001 ECG/BIOZ chip 00035 * 00036 * @code 00037 * #include "mbed.h" 00038 * #include "MAX30001.h" 00039 * 00040 * /// Initialization values for ECG_InitStart() 00041 * #define EN_ECG 0b1 00042 * #define OPENP 0b1 00043 * #define OPENN 0b1 00044 * #define POL 0b0 00045 * #define CALP_SEL 0b10 00046 * #define CALN_SEL 0b11 00047 * #define E_FIT 31 00048 * #define RATE 0b00 00049 * #define GAIN 0b00 00050 * #define DHPF 0b0 00051 * #define DLPF 0b01 00052 * 00053 * /// Initialization values for CAL_InitStart() 00054 * #define EN_VCAL 0b1 00055 * #define VMODE 0b1 00056 * #define VMAG 0b1 00057 * #define FCAL 0b011 00058 * #define THIGH 0x7FF 00059 * #define FIFTY 0b0 00060 * 00061 * /// Initializaton values for Rbias_FMSTR_Init() 00062 * #define EN_RBIAS 0b01 00063 * #define RBIASV 0b10 00064 * #define RBIASP 0b1 00065 * #define RBIASN 0b1 00066 * #define FMSTR 0b00 00067 * 00068 * #define BUFFER_LENGTH 50 00069 * 00070 * // @brief SPI Master 0 with SPI0_SS for use with MAX30001 00071 * SPI spi(SPI0_MOSI, SPI0_MISO, SPI0_SCK, SPI0_SS); // used by MAX30001 00072 * 00073 * //@brief ECG device 00074 * MAX30001 max30001(&spi); 00075 * InterruptIn max30001_InterruptB(P3_6); 00076 * InterruptIn max30001_Interrupt2B(P4_5); 00077 * //@brief PWM used as fclk for the MAX30001 00078 * PwmOut pwmout(P1_7); 00079 * 00080 * //@brief Creating a buffer to hold the data 00081 * uint32_t ecgBuffer[BUFFER_LENGTH]; 00082 * int ecgIndex = 0; 00083 * char data_trigger = 0; 00084 * 00085 * 00086 * // 00087 * // @brief Creates a packet that will be streamed via USB Serial 00088 * // the packet created will be inserted into a fifo to be streamed at a later time 00089 * // @param id Streaming ID 00090 * // @param buffer Pointer to a uint32 array that contains the data to include in the packet 00091 * // @param number Number of elements in the buffer 00092 * // 00093 * void StreamPacketUint32_ecg(uint32_t id, uint32_t *buffer, uint32_t number) { 00094 * int i; 00095 * if (id == MAX30001_DATA_ECG) { 00096 * for (i = 0; i < number; i++) { 00097 * ecgBuffer[ecgIndex] = buffer[i]; 00098 * ecgIndex++; 00099 * if (ecgIndex > BUFFER_LENGTH) 00100 * { 00101 * data_trigger = 1; 00102 * ecgIndex = 0; 00103 * } 00104 * } 00105 * } 00106 * if (id == MAX30001_DATA_BIOZ) { 00107 * /// Add code for reading BIOZ data 00108 * } 00109 * if (id == MAX30001_DATA_PACE) { 00110 * /// Add code for reading Pace data 00111 * } 00112 * if (id == MAX30001_DATA_RTOR) { 00113 * /// Add code for reading RtoR data 00114 * } 00115 * } 00116 * 00117 * 00118 * int main() { 00119 * 00120 * uint32_t all; 00121 * 00122 * /// set NVIC priorities for GPIO to prevent priority inversion 00123 * NVIC_SetPriority(GPIO_P0_IRQn, 5); 00124 * NVIC_SetPriority(GPIO_P1_IRQn, 5); 00125 * NVIC_SetPriority(GPIO_P2_IRQn, 5); 00126 * NVIC_SetPriority(GPIO_P3_IRQn, 5); 00127 * NVIC_SetPriority(GPIO_P4_IRQn, 5); 00128 * NVIC_SetPriority(GPIO_P5_IRQn, 5); 00129 * NVIC_SetPriority(GPIO_P6_IRQn, 5); 00130 * // used by the MAX30001 00131 * NVIC_SetPriority(SPI1_IRQn, 0); 00132 * 00133 * 00134 * /// Setup interrupts and callback functions 00135 * max30001_InterruptB.disable_irq(); 00136 * max30001_Interrupt2B.disable_irq(); 00137 * 00138 * max30001_InterruptB.mode(PullUp); 00139 * max30001_InterruptB.fall(&MAX30001::Mid_IntB_Handler); 00140 * 00141 * max30001_Interrupt2B.mode(PullUp); 00142 * max30001_Interrupt2B.fall(&MAX30001::Mid_Int2B_Handler); 00143 * 00144 * max30001_InterruptB.enable_irq(); 00145 * max30001_Interrupt2B.enable_irq(); 00146 * 00147 * max30001.AllowInterrupts(1); 00148 * 00149 * // Configuring the FCLK for the ECG, set to 32.768KHZ 00150 * pwmout.period_us(31); 00151 * pwmout.write(0.5); // 0-1 is 0-100%, 0.5 = 50% duty cycle. 00152 * max30001.sw_rst(); // Do a software reset of the MAX30001 00153 * 00154 * max30001.INT_assignment(MAX30001::MAX30001_INT_B, MAX30001::MAX30001_NO_INT, MAX30001::MAX30001_NO_INT, // en_enint_loc, en_eovf_loc, en_fstint_loc, 00155 * MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_NO_INT, // en_dcloffint_loc, en_bint_loc, en_bovf_loc, 00156 * MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_NO_INT, // en_bover_loc, en_bundr_loc, en_bcgmon_loc, 00157 * MAX30001::MAX30001_INT_B, MAX30001::MAX30001_NO_INT, MAX30001::MAX30001_NO_INT, // en_pint_loc, en_povf_loc, en_pedge_loc, 00158 * MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_INT_B, MAX30001::MAX30001_NO_INT, // en_lonint_loc, en_rrint_loc, en_samp_loc, 00159 * MAX30001::MAX30001_INT_ODNR, MAX30001::MAX30001_INT_ODNR); // intb_Type, int2b_Type) 00160 * 00161 * max30001.onDataAvailable(&StreamPacketUint32_ecg); 00162 * 00163 * /// Set and Start the VCAL input 00164 * /// @brief NOTE VCAL must be set first if VCAL is to be used 00165 * max30001.CAL_InitStart(EN_VCAL , VMODE, VMAG, FCAL, THIGH, FIFTY); 00166 * 00167 * /// ECG Initialization 00168 * max30001.ECG_InitStart(EN_ECG, OPENP, OPENN, POL, CALP_SEL, CALN_SEL, E_FIT, RATE, GAIN, DHPF, DLPF); 00169 * 00170 * /// @details The user can call any of the InitStart functions for Pace, BIOZ and RtoR 00171 * 00172 * 00173 * /// @brief Set Rbias & FMSTR over here 00174 * max30001.Rbias_FMSTR_Init(EN_RBIAS, RBIASV, RBIASP, RBIASN,FMSTR); 00175 * 00176 * max30001.synch(); 00177 * 00178 * /// clear the status register for a clean start 00179 * max30001.reg_read(MAX30001::STATUS, &all); 00180 * 00181 * printf("Please wait for data to start streaming\n"); 00182 * fflush(stdout); 00183 * 00184 * while (1) { 00185 * if(data_trigger == 1){ 00186 * printf("%ld ", ecgBuffer[ecgIndex]); // Print the ECG data on a serial port terminal software 00187 * fflush(stdout); 00188 * } 00189 * } 00190 * } 00191 * @endcode 00192 * 00193 */ 00194 00195 00196 #ifndef MAX30001_H_ 00197 #define MAX30001_H_ 00198 00199 #include "mbed.h" 00200 00201 #define mbed_COMPLIANT ///< Uncomment to Use timer for MAX30001 FCLK (for mbed) 00202 ///< Comment to use the RTC clock 00203 00204 #define ASYNC_SPI_BUFFER_SIZE (32 * 3) ///< Maximimum buffer size for async byte transfers 00205 00206 ///< Defines for data callbacks 00207 #define MAX30001_DATA_ECG 0x30 00208 #define MAX30001_DATA_PACE 0x31 00209 #define MAX30001_DATA_RTOR 0x32 00210 #define MAX30001_DATA_BIOZ 0x33 00211 #define MAX30001_DATA_LEADOFF_DC 0x34 00212 #define MAX30001_DATA_LEADOFF_AC 0x35 00213 #define MAX30001_DATA_BCGMON 0x36 00214 #define MAX30001_DATA_ACLEADON 0x37 00215 00216 #define MAX30001_SPI_MASTER_PORT 0 00217 #define MAX30001_SPI_SS_INDEX 0 00218 00219 #define MAX30001_INT_PORT_B 3 00220 #define MAX30001_INT_PIN_B 6 00221 00222 #define MAX30001_INT_PORT_2B 4 00223 #define MAX30001_INT_PIN_2B 5 00224 00225 #define MAX30001_INT_PORT_FCLK 1 00226 #define MAX30001_INT_PIN_FCLK 7 00227 00228 #define MAX30001_FUNC_SEL_TMR 2 ///< 0=FW Control, 1= Pulse Train, 2=Timer 00229 00230 #define MAX30001_INDEX 3 00231 #define MAX30001_POLARITY 0 00232 #define MAX30001_PERIOD 30518 00233 #define MAX30001_CYCLE 50 00234 00235 #define MAX30001_IOMUX_IO_ENABLE 1 00236 00237 #define MAX30001_SPI_PORT 0 00238 #define MAX30001_CS_PIN 0 00239 #define MAX30001_CS_POLARITY 0 00240 #define MAX30001_CS_ACTIVITY_DELAY 0 00241 #define MAX30001_CS_INACTIVITY_DELAY 0 00242 #define MAX30001_CLK_HI 1 00243 #define MAX30001_CLK_LOW 1 00244 #define MAX30001_ALT_CLK 0 00245 #define MAX30001_CLK_POLARITY 0 00246 #define MAX30001_CLK_PHASE 0 00247 #define MAX30001_WRITE 1 00248 #define MAX30001_READ 0 00249 00250 #define MAX30001_INT_PORT_B 3 00251 #define MAX30001INT_PIN_B 6 00252 00253 void MAX30001_AllowInterrupts(int state); 00254 00255 /** 00256 * @brief Maxim Integrated MAX30001 ECG/BIOZ chip 00257 */ 00258 class MAX30001 { 00259 00260 public: 00261 typedef enum { ///< MAX30001 Register addresses 00262 STATUS = 0x01, 00263 EN_INT = 0x02, 00264 EN_INT2 = 0x03, 00265 MNGR_INT = 0x04, 00266 MNGR_DYN = 0x05, 00267 SW_RST = 0x08, 00268 SYNCH = 0x09, 00269 FIFO_RST = 0x0A, 00270 INFO = 0x0F, 00271 CNFG_GEN = 0x10, 00272 CNFG_CAL = 0x12, 00273 CNFG_EMUX = 0x14, 00274 CNFG_ECG = 0x15, 00275 CNFG_BMUX = 0x17, 00276 CNFG_BIOZ = 0x18, 00277 CNFG_PACE = 0x1A, 00278 CNFG_RTOR1 = 0x1D, 00279 CNFG_RTOR2 = 0x1E, 00280 00281 // Data locations 00282 ECG_FIFO_BURST = 0x20, 00283 ECG_FIFO = 0x21, 00284 FIFO_BURST = 0x22, 00285 BIOZ_FIFO = 0x23, 00286 RTOR = 0x25, 00287 00288 PACE0_FIFO_BURST = 0x30, 00289 PACE0_A = 0x31, 00290 PACE0_B = 0x32, 00291 PACE0_C = 0x33, 00292 00293 PACE1_FIFO_BURST = 0x34, 00294 PACE1_A = 0x35, 00295 PACE1_B = 0x36, 00296 PACE1_C = 0x37, 00297 00298 PACE2_FIFO_BURST = 0x38, 00299 PACE2_A = 0x39, 00300 PACE2_B = 0x3A, 00301 PACE2_C = 0x3B, 00302 00303 PACE3_FIFO_BURST = 0x3C, 00304 PACE3_A = 0x3D, 00305 PACE3_B = 0x3E, 00306 PACE3_C = 0x3F, 00307 00308 PACE4_FIFO_BURST = 0x40, 00309 PACE4_A = 0x41, 00310 PACE4_B = 0x42, 00311 PACE4_C = 0x43, 00312 00313 PACE5_FIFO_BURST = 0x44, 00314 PACE5_A = 0x45, 00315 PACE5_B = 0x46, 00316 PACE5_C = 0x47, 00317 00318 } MAX30001_REG_map_t ; 00319 00320 /** 00321 * @brief STATUS (0x01) 00322 */ 00323 typedef union max30001_status_reg { 00324 uint32_t all; 00325 00326 struct { 00327 uint32_t loff_nl : 1; 00328 uint32_t loff_nh : 1; 00329 uint32_t loff_pl : 1; 00330 uint32_t loff_ph : 1; 00331 00332 uint32_t bcgmn : 1; 00333 uint32_t bcgmp : 1; 00334 uint32_t reserved1 : 1; 00335 uint32_t reserved2 : 1; 00336 00337 uint32_t pllint : 1; 00338 uint32_t samp : 1; 00339 uint32_t rrint : 1; 00340 uint32_t lonint : 1; 00341 00342 uint32_t pedge : 1; 00343 uint32_t povf : 1; 00344 uint32_t pint : 1; 00345 uint32_t bcgmon : 1; 00346 00347 uint32_t bundr : 1; 00348 uint32_t bover : 1; 00349 uint32_t bovf : 1; 00350 uint32_t bint : 1; 00351 00352 uint32_t dcloffint : 1; 00353 uint32_t fstint : 1; 00354 uint32_t eovf : 1; 00355 uint32_t eint : 1; 00356 00357 uint32_t reserved : 8; 00358 00359 } bit; 00360 00361 } max30001_status_t; 00362 00363 00364 /** 00365 * @brief EN_INT (0x02) 00366 */ 00367 00368 typedef union max30001_en_int_reg { 00369 uint32_t all; 00370 00371 struct { 00372 uint32_t intb_type : 2; 00373 uint32_t reserved1 : 1; 00374 uint32_t reserved2 : 1; 00375 00376 uint32_t reserved3 : 1; 00377 uint32_t reserved4 : 1; 00378 uint32_t reserved5 : 1; 00379 uint32_t reserved6 : 1; 00380 00381 uint32_t en_pllint : 1; 00382 uint32_t en_samp : 1; 00383 uint32_t en_rrint : 1; 00384 uint32_t en_lonint : 1; 00385 00386 uint32_t en_pedge : 1; 00387 uint32_t en_povf : 1; 00388 uint32_t en_pint : 1; 00389 uint32_t en_bcgmon : 1; 00390 00391 uint32_t en_bundr : 1; 00392 uint32_t en_bover : 1; 00393 uint32_t en_bovf : 1; 00394 uint32_t en_bint : 1; 00395 00396 uint32_t en_dcloffint : 1; 00397 uint32_t en_fstint : 1; 00398 uint32_t en_eovf : 1; 00399 uint32_t en_eint : 1; 00400 00401 uint32_t reserved : 8; 00402 00403 } bit; 00404 00405 } max30001_en_int_t; 00406 00407 00408 /** 00409 * @brief EN_INT2 (0x03) 00410 */ 00411 typedef union max30001_en_int2_reg { 00412 uint32_t all; 00413 00414 struct { 00415 uint32_t intb_type : 2; 00416 uint32_t reserved1 : 1; 00417 uint32_t reserved2 : 1; 00418 00419 uint32_t reserved3 : 1; 00420 uint32_t reserved4 : 1; 00421 uint32_t reserved5 : 1; 00422 uint32_t reserved6 : 1; 00423 00424 uint32_t en_pllint : 1; 00425 uint32_t en_samp : 1; 00426 uint32_t en_rrint : 1; 00427 uint32_t en_lonint : 1; 00428 00429 uint32_t en_pedge : 1; 00430 uint32_t en_povf : 1; 00431 uint32_t en_pint : 1; 00432 uint32_t en_bcgmon : 1; 00433 00434 uint32_t en_bundr : 1; 00435 uint32_t en_bover : 1; 00436 uint32_t en_bovf : 1; 00437 uint32_t en_bint : 1; 00438 00439 uint32_t en_dcloffint : 1; 00440 uint32_t en_fstint : 1; 00441 uint32_t en_eovf : 1; 00442 uint32_t en_eint : 1; 00443 00444 uint32_t reserved : 8; 00445 00446 } bit; 00447 00448 } max30001_en_int2_t; 00449 00450 /** 00451 * @brief MNGR_INT (0x04) 00452 */ 00453 typedef union max30001_mngr_int_reg { 00454 uint32_t all; 00455 00456 struct { 00457 uint32_t samp_it : 2; 00458 uint32_t clr_samp : 1; 00459 uint32_t clr_pedge : 1; 00460 uint32_t clr_rrint : 2; 00461 uint32_t clr_fast : 1; 00462 uint32_t reserved1 : 1; 00463 uint32_t reserved2 : 4; 00464 uint32_t reserved3 : 4; 00465 00466 uint32_t b_fit : 3; 00467 uint32_t e_fit : 5; 00468 00469 uint32_t reserved : 8; 00470 00471 } bit; 00472 00473 } max30001_mngr_int_t; 00474 00475 /** 00476 * @brief MNGR_DYN (0x05) 00477 */ 00478 typedef union max30001_mngr_dyn_reg { 00479 uint32_t all; 00480 00481 struct { 00482 uint32_t bloff_lo_it : 8; 00483 uint32_t bloff_hi_it : 8; 00484 uint32_t fast_th : 6; 00485 uint32_t fast : 2; 00486 uint32_t reserved : 8; 00487 } bit; 00488 00489 } max30001_mngr_dyn_t; 00490 00491 00492 /** 00493 * @brief INFO (0x0F) 00494 */ 00495 typedef union max30001_info_reg { 00496 uint32_t all; 00497 struct { 00498 uint32_t serial : 12; 00499 uint32_t part_id : 2; 00500 uint32_t sample : 1; 00501 uint32_t reserved1 : 1; 00502 uint32_t rev_id : 4; 00503 uint32_t pattern : 4; 00504 uint32_t reserved : 8; 00505 } bit; 00506 00507 } max30001_info_t; 00508 00509 /** 00510 * @brief CNFG_GEN (0x10) 00511 */ 00512 typedef union max30001_cnfg_gen_reg { 00513 uint32_t all; 00514 struct { 00515 uint32_t rbiasn : 1; 00516 uint32_t rbiasp : 1; 00517 uint32_t rbiasv : 2; 00518 uint32_t en_rbias : 2; 00519 uint32_t vth : 2; 00520 uint32_t imag : 3; 00521 uint32_t ipol : 1; 00522 uint32_t en_dcloff : 2; 00523 uint32_t en_bloff : 2; 00524 uint32_t reserved1 : 1; 00525 uint32_t en_pace : 1; 00526 uint32_t en_bioz : 1; 00527 uint32_t en_ecg : 1; 00528 uint32_t fmstr : 2; 00529 uint32_t en_ulp_lon : 2; 00530 uint32_t reserved : 8; 00531 } bit; 00532 00533 } max30001_cnfg_gen_t; 00534 00535 00536 /** 00537 * @brief CNFG_CAL (0x12) 00538 */ 00539 typedef union max30001_cnfg_cal_reg { 00540 uint32_t all; 00541 struct { 00542 uint32_t thigh : 11; 00543 uint32_t fifty : 1; 00544 uint32_t fcal : 3; 00545 uint32_t reserved1 : 5; 00546 uint32_t vmag : 1; 00547 uint32_t vmode : 1; 00548 uint32_t en_vcal : 1; 00549 uint32_t reserved2 : 1; 00550 uint32_t reserved : 8; 00551 } bit; 00552 00553 } max30001_cnfg_cal_t; 00554 00555 /** 00556 * @brief CNFG_EMUX (0x14) 00557 */ 00558 typedef union max30001_cnfg_emux_reg { 00559 uint32_t all; 00560 struct { 00561 uint32_t reserved1 : 16; 00562 uint32_t caln_sel : 2; 00563 uint32_t calp_sel : 2; 00564 uint32_t openn : 1; 00565 uint32_t openp : 1; 00566 uint32_t reserved2 : 1; 00567 uint32_t pol : 1; 00568 uint32_t reserved : 8; 00569 } bit; 00570 00571 } max30001_cnfg_emux_t; 00572 00573 00574 /** 00575 * @brief CNFG_ECG (0x15) 00576 */ 00577 typedef union max30001_cnfg_ecg_reg { 00578 uint32_t all; 00579 struct { 00580 uint32_t reserved1 : 12; 00581 uint32_t dlpf : 2; 00582 uint32_t dhpf : 1; 00583 uint32_t reserved2 : 1; 00584 uint32_t gain : 2; 00585 uint32_t reserved3 : 4; 00586 uint32_t rate : 2; 00587 00588 uint32_t reserved : 8; 00589 } bit; 00590 00591 } max30001_cnfg_ecg_t; 00592 00593 /** 00594 * @brief CNFG_BMUX (0x17) 00595 */ 00596 typedef union max30001_cnfg_bmux_reg { 00597 uint32_t all; 00598 struct { 00599 uint32_t fbist : 2; 00600 uint32_t reserved1 : 2; 00601 uint32_t rmod : 3; 00602 uint32_t reserved2 : 1; 00603 uint32_t rnom : 3; 00604 uint32_t en_bist : 1; 00605 uint32_t cg_mode : 2; 00606 uint32_t reserved3 : 2; 00607 uint32_t caln_sel : 2; 00608 uint32_t calp_sel : 2; 00609 uint32_t openn : 1; 00610 uint32_t openp : 1; 00611 uint32_t reserved4 : 2; 00612 uint32_t reserved : 8; 00613 } bit; 00614 00615 } max30001_cnfg_bmux_t; 00616 00617 /** 00618 * @brief CNFG_BIOZ (0x18) 00619 */ 00620 typedef union max30001_bioz_reg { 00621 uint32_t all; 00622 struct { 00623 uint32_t phoff : 4; 00624 uint32_t cgmag : 3; 00625 uint32_t cgmon : 1; 00626 uint32_t fcgen : 4; 00627 uint32_t dlpf : 2; 00628 uint32_t dhpf : 2; 00629 uint32_t gain : 2; 00630 uint32_t reserved1 : 1; 00631 uint32_t ext_rbias : 1; 00632 uint32_t ahpf : 3; 00633 uint32_t rate : 1; 00634 uint32_t reserved : 8; 00635 } bit; 00636 00637 } max30001_cnfg_bioz_t; 00638 00639 00640 /** 00641 * @brief CNFG_PACE (0x1A) 00642 */ 00643 typedef union max30001_cnfg_pace_reg { 00644 uint32_t all; 00645 00646 struct { 00647 uint32_t dacn : 4; 00648 uint32_t dacp : 4; 00649 uint32_t reserved1 : 4; 00650 uint32_t aout : 2; 00651 uint32_t aout_lbw : 1; 00652 uint32_t reserved2 : 1; 00653 uint32_t gain : 3; 00654 uint32_t gn_diff_off : 1; 00655 uint32_t reserved3 : 3; 00656 uint32_t pol : 1; 00657 uint32_t reserved : 8; 00658 } bit; 00659 00660 } max30001_cnfg_pace_t; 00661 00662 /** 00663 * @brief CNFG_RTOR1 (0x1D) 00664 */ 00665 typedef union max30001_cnfg_rtor1_reg { 00666 uint32_t all; 00667 struct { 00668 uint32_t reserved1 : 8; 00669 uint32_t ptsf : 4; 00670 uint32_t pavg : 2; 00671 uint32_t reserved2 : 1; 00672 uint32_t en_rtor : 1; 00673 uint32_t gain : 4; 00674 uint32_t wndw : 4; 00675 uint32_t reserved : 8; 00676 } bit; 00677 00678 } max30001_cnfg_rtor1_t; 00679 00680 /** 00681 * @brief CNFG_RTOR2 (0x1E) 00682 */ 00683 typedef union max30001_cnfg_rtor2_reg { 00684 uint32_t all; 00685 struct { 00686 uint32_t reserved1 : 8; 00687 uint32_t rhsf : 3; 00688 uint32_t reserved2 : 1; 00689 uint32_t ravg : 2; 00690 uint32_t reserved3 : 2; 00691 uint32_t hoff : 6; 00692 uint32_t reserved4 : 2; 00693 uint32_t reserved : 8; 00694 } bit; 00695 00696 } max30001_cnfg_rtor2_t; 00697 00698 /*********************************************************************************/ 00699 00700 typedef enum { 00701 MAX30001_NO_INT = 0, ///< No interrupt 00702 MAX30001_INT_B = 1, ///< INTB selected for interrupt 00703 MAX30001_INT_2B = 2 ///< INT2B selected for interrupt 00704 } max30001_intrpt_Location_t ; 00705 00706 typedef enum { 00707 MAX30001_INT_DISABLED = 0b00, 00708 MAX30001_INT_CMOS = 0b01, 00709 MAX30001_INT_ODN = 0b10, 00710 MAX30001_INT_ODNR = 0b11 00711 } max30001_intrpt_type_t; 00712 00713 typedef enum { ///< Input Polarity selection 00714 MAX30001_NON_INV = 0, ///< Non-Inverted 00715 MAX30001_INV = 1 ///< Inverted 00716 } max30001_emux_pol_t ; 00717 00718 typedef enum { ///< OPENP and OPENN setting 00719 MAX30001_ECG_CON_AFE = 0, ///< ECGx is connected to AFE channel 00720 MAX30001_ECG_ISO_AFE = 1 ///< ECGx is isolated from AFE channel 00721 } max30001_emux_openx_t ; 00722 00723 typedef enum { ///< EMUX_CALP_SEL & EMUX_CALN_SEL 00724 MAX30001_NO_CAL_SIG = 0b00, ///< No calibration signal is applied 00725 MAX30001_INPT_VMID = 0b01, ///< Input is connected to VMID 00726 MAX30001_INPT_VCALP = 0b10, ///< Input is connected to VCALP 00727 MAX30001_INPT_VCALN = 0b11 ///< Input is connected to VCALN 00728 } max30001_emux_calx_sel_t ; 00729 00730 typedef enum { ///< EN_ECG, EN_BIOZ, EN_PACE 00731 MAX30001_CHANNEL_DISABLED = 0b0, 00732 MAX30001_CHANNEL_ENABLED = 0b1 00733 } max30001_en_feature_t ; 00734 00735 /*********************************************************************************/ 00736 // Data 00737 uint32_t max30001_ECG_FIFO_buffer[32]; ///< (303 for internal test) 00738 uint32_t max30001_BIOZ_FIFO_buffer[8]; ///< (303 for internal test) 00739 00740 uint32_t max30001_PACE[18]; ///< Pace Data 0-5 00741 00742 uint32_t max30001_RtoR_data; ///< This holds the RtoR data 00743 00744 uint32_t max30001_DCLeadOff; ///< This holds the LeadOff data, Last 4 bits give 00745 ///< the status, BIT3=LOFF_PH, BIT2=LOFF_PL, 00746 ///< BIT1=LOFF_NH, BIT0=LOFF_NL 00747 ///< 8th and 9th bits tell Lead off is due to ECG or BIOZ. 00748 ///< 0b01 = ECG Lead Off and 0b10 = BIOZ Lead off 00749 00750 uint32_t max30001_ACLeadOff; ///< This gives the state of the BIOZ AC Lead Off 00751 ///< state. BIT 1 = BOVER, BIT 0 = BUNDR 00752 00753 uint32_t max30001_bcgmon; ///< This holds the BCGMON data, BIT 1 = BCGMP, BIT0 = 00754 ///< BCGMN 00755 00756 uint32_t max30001_LeadOn; ///< This holds the LeadOn data, BIT1 = BIOZ Lead ON, 00757 ///< BIT0 = ECG Lead ON, BIT8= Lead On Status Bit 00758 00759 uint32_t max30001_timeout; ///< If the PLL does not respond, timeout and get out. 00760 00761 typedef struct { ///< Creating a structure for BLE data 00762 int16_t R2R; 00763 int16_t fmstr; 00764 } max30001_bledata_t; 00765 00766 max30001_bledata_t hspValMax30001; // R2R, FMSTR 00767 00768 max30001_status_t global_status; 00769 00770 /** 00771 * @brief Constructor that accepts pin names for the SPI interface 00772 * @param spi pointer to the mbed SPI object 00773 */ 00774 MAX30001(SPI *spi); 00775 00776 /** 00777 * @brief Constructor that accepts pin names for the SPI interface 00778 * @param mosi master out slave in pin name 00779 * @param miso master in slave out pin name 00780 * @param sclk serial clock pin name 00781 * @param cs chip select pin name 00782 */ 00783 MAX30001(PinName mosi, PinName miso, PinName sclk, PinName cs); 00784 00785 /** 00786 * MAX30001 destructor 00787 */ 00788 ~MAX30001(void); 00789 00790 /** 00791 * @brief This function is MAXIM Proprietary. It channels the RTC crystal 00792 * @brief clock to P1.7. Thus providing 32768Hz on FCLK pin of the MAX30001-3 00793 */ 00794 void FCLK_MaximOnly(void); 00795 00796 /** 00797 * @brief This function sets up the Resistive Bias mode and also selects the master clock frequency. 00798 * @brief Uses Register: CNFG_GEN-0x10 00799 * @param En_rbias: Enable and Select Resitive Lead Bias Mode 00800 * @param Rbiasv: Resistive Bias Mode Value Selection 00801 * @param Rbiasp: Enables Resistive Bias on Positive Input 00802 * @param Rbiasn: Enables Resistive Bias on Negative Input 00803 * @param Fmstr: Selects Master Clock Frequency 00804 * @returns 0-if no error. A non-zero value indicates an error. 00805 * 00806 */ 00807 int Rbias_FMSTR_Init(uint8_t En_rbias, uint8_t Rbiasv, 00808 uint8_t Rbiasp, uint8_t Rbiasn, uint8_t Fmstr); 00809 00810 /** 00811 * @brief This function uses sets up the calibration signal internally. If it is desired to use the internal signal, then 00812 * @brief this function must be called and the registers set, prior to setting the CALP_SEL and CALN_SEL in the ECG_InitStart 00813 * @brief and BIOZ_InitStart functions. 00814 * @brief Uses Register: CNFG_CAL-0x12 00815 * @param En_Vcal: Calibration Source (VCALP and VCALN) Enable 00816 * @param Vmode: Calibration Source Mode Selection 00817 * @param Vmag: Calibration Source Magnitude Selection (VMAG) 00818 * @param Fcal: Calibration Source Frequency Selection (FCAL) 00819 * @param Thigh: Calibration Source Time High Selection 00820 * @param Fifty: Calibration Source Duty Cycle Mode Selection 00821 * @returns 0-if no error. A non-zero value indicates an error. 00822 * 00823 */ 00824 int CAL_InitStart(uint8_t En_Vcal, uint8_t Vmode, uint8_t Vmag, 00825 uint8_t Fcal, uint16_t Thigh, uint8_t Fifty); 00826 00827 /** 00828 * @brief This function disables the VCAL signal 00829 * @returns 0-if no error. A non-zero value indicates an error. 00830 */ 00831 int CAL_Stop(void); 00832 00833 /** 00834 * @brief This function handles the assignment of the two interrupt pins (INTB & INT2B) with various 00835 * @brief functions/behaviors of the MAX30001. Also, each pin can be configured for different drive capability. 00836 * @brief Uses Registers: EN_INT-0x02 and EN_INT2-0x03. 00837 * @param max30001_intrpt_Locatio_t <argument>: All the arguments with the aforementioned enumeration essentially 00838 * can be configured to generate an interrupt on either INTB or INT2B or NONE. 00839 * @param max30001_intrpt_type_t intb_Type: INTB Port Type (EN_INT Selections). 00840 * @param max30001_intrpt_type _t int2b_Type: INT2B Port Type (EN_INT2 Selections) 00841 * @returns 0-if no error. A non-zero value indicates an error. 00842 * 00843 */ 00844 int INT_assignment(max30001_intrpt_Location_t en_enint_loc, max30001_intrpt_Location_t en_eovf_loc, max30001_intrpt_Location_t en_fstint_loc, 00845 max30001_intrpt_Location_t en_dcloffint_loc, max30001_intrpt_Location_t en_bint_loc, max30001_intrpt_Location_t en_bovf_loc, 00846 max30001_intrpt_Location_t en_bover_loc, max30001_intrpt_Location_t en_bundr_loc, max30001_intrpt_Location_t en_bcgmon_loc, 00847 max30001_intrpt_Location_t en_pint_loc, max30001_intrpt_Location_t en_povf_loc, max30001_intrpt_Location_t en_pedge_loc, 00848 max30001_intrpt_Location_t en_lonint_loc, max30001_intrpt_Location_t en_rrint_loc, max30001_intrpt_Location_t en_samp_loc, 00849 max30001_intrpt_type_t intb_Type, max30001_intrpt_type_t int2b_Type); 00850 00851 00852 00853 /** 00854 * @brief For MAX30001/3 ONLY 00855 * @brief This function sets up the MAX30001 for the ECG measurements. 00856 * @brief Registers used: CNFG_EMUX, CNFG_GEN, MNGR_INT, CNFG_ECG. 00857 * @param En_ecg: ECG Channel Enable <CNFG_GEN register bits> 00858 * @param Openp: Open the ECGN Input Switch (most often used for testing and calibration studies) <CNFG_EMUX register bits> 00859 * @param Openn: Open the ECGN Input Switch (most often used for testing and calibration studies) <CNFG_EMUX register bits> 00860 * @param Calp_sel: ECGP Calibration Selection <CNFG_EMUX register bits> 00861 * @param Caln_sel: ECGN Calibration Selection <CNFG_EMUX register bits> 00862 * @param E_fit: ECG FIFO Interrupt Threshold (issues EINT based on number of unread FIFO records) <CNFG_GEN register bits> 00863 * @param Clr_rrint: RTOR R Detect Interrupt (RRINT) Clear Behavior <CNFG_GEN register bits> 00864 * @param Rate: ECG Data Rate 00865 * @param Gain: ECG Channel Gain Setting 00866 * @param Dhpf: ECG Channel Digital High Pass Filter Cutoff Frequency 00867 * @param Dlpf: ECG Channel Digital Low Pass Filter Cutoff Frequency 00868 * @returns 0-if no error. A non-zero value indicates an error. 00869 * 00870 */ 00871 int ECG_InitStart(uint8_t En_ecg, uint8_t Openp, uint8_t Openn, 00872 uint8_t Pol, uint8_t Calp_sel, uint8_t Caln_sel, 00873 uint8_t E_fit, uint8_t Rate, uint8_t Gain, 00874 uint8_t Dhpf, uint8_t Dlpf); 00875 00876 /** 00877 * @brief For MAX30001/3 ONLY 00878 * @brief This function enables the Fast mode feature of the ECG. 00879 * @brief Registers used: MNGR_INT-0x04, MNGR_DYN-0x05 00880 * @param Clr_Fast: FAST MODE Interrupt Clear Behavior <MNGR_INT Register> 00881 * @param Fast: ECG Channel Fast Recovery Mode Selection (ECG High Pass Filter Bypass) <MNGR_DYN Register> 00882 * @param Fast_Th: Automatic Fast Recovery Threshold 00883 * @returns 0-if no error. A non-zero value indicates an error. 00884 * 00885 */ 00886 int ECGFast_Init(uint8_t Clr_Fast, uint8_t Fast, uint8_t Fast_Th); 00887 00888 /** 00889 * @brief For MAX30001/3 ONLY 00890 * @brief This function disables the ECG. 00891 * @brief Uses Register CNFG_GEN-0x10. 00892 * @returns 0-if no error. A non-zero value indicates an error. 00893 * 00894 */ 00895 int Stop_ECG(void); 00896 00897 /** 00898 * @brief For MAX30001 ONLY 00899 * @brief This function sets up the MAX30001 for pace signal detection. 00900 * @brief If both PACE and BIOZ are turned ON, then make sure Fcgen is set for 80K or 40K in the 00901 * @brief max30001_BIOZ_InitStart() function. However, if Only PACE is on but BIOZ off, then Fcgen can be set 00902 * @brief for 80K only, in the max30001_BIOZ_InitStart() function 00903 * @brief Registers used: MNGR_INT-0x04, CNFG_GEN-0x37, CNFG_PACE-0x1A. 00904 * @param En_pace : PACE Channel Enable <CNFG_GEN Register> 00905 * @param Clr_pedge : PACE Edge Detect Interrupt (PEDGE) Clear Behavior <MNGR_INT Register> 00906 * @param Pol: PACE Input Polarity Selection <CNFG_PACE Register> 00907 * @param Gn_diff_off: PACE Differentiator Mode <CNFG_PACE Register> 00908 * @param Gain: PACE Channel Gain Selection <CNFG_PACE Register> 00909 * @param Aout_lbw: PACE Analog Output Buffer Bandwidth Mode <CNFG_PACE Register> 00910 * @param Aout: PACE Single Ended Analog Output Buffer Signal Monitoring Selection <CNFG_PACE Register> 00911 * @param Dacp (4bits): PACE Detector Positive Comparator Threshold <CNFG_PACE Register> 00912 * @param Dacn(4bits): PACE Detector Negative Comparator Threshold <CNFG_PACE Register> 00913 * @returns 0-if no error. A non-zero value indicates an error <CNFG_PACE Register> 00914 * 00915 */ 00916 int PACE_InitStart(uint8_t En_pace, uint8_t Clr_pedge, uint8_t Pol, 00917 uint8_t Gn_diff_off, uint8_t Gain, 00918 uint8_t Aout_lbw, uint8_t Aout, uint8_t Dacp, 00919 uint8_t Dacn); 00920 00921 /** 00922 *@brief For MAX30001 ONLY 00923 *@param This function disables the PACE. Uses Register CNFG_GEN-0x10. 00924 *@returns 0-if no error. A non-zero value indicates an error. 00925 * 00926 */ 00927 int Stop_PACE(void); 00928 00929 /** 00930 * @brief For MAX30001/2 ONLY 00931 * @brief This function sets up the MAX30001 for BIOZ measurement. 00932 * @brief Registers used: MNGR_INT-0x04, CNFG_GEN-0X10, CNFG_BMUX-0x17,CNFG_BIOZ-0x18. 00933 * @param En_bioz: BIOZ Channel Enable <CNFG_GEN Register> 00934 * @param Openp: Open the BIP Input Switch <CNFG_BMUX Register> 00935 * @param Openn: Open the BIN Input Switch <CNFG_BMUX Register> 00936 * @param Calp_sel: BIP Calibration Selection <CNFG_BMUX Register> 00937 * @param Caln_sel: BIN Calibration Selection <CNFG_BMUX Register> 00938 * @param CG_mode: BIOZ Current Generator Mode Selection <CNFG_BMUX Register> 00939 * @param B_fit: BIOZ FIFO Interrupt Threshold (issues BINT based on number of unread FIFO records) <MNGR_INT Register> 00940 * @param Rate: BIOZ Data Rate <CNFG_BIOZ Register> 00941 * @param Ahpf: BIOZ/PACE Channel Analog High Pass Filter Cutoff Frequency and Bypass <CNFG_BIOZ Register> 00942 * @param Ext_rbias: External Resistor Bias Enable <CNFG_BIOZ Register> 00943 * @param Gain: BIOZ Channel Gain Setting <CNFG_BIOZ Register> 00944 * @param Dhpf: BIOZ Channel Digital High Pass Filter Cutoff Frequency <CNFG_BIOZ Register> 00945 * @param Dlpf: BIOZ Channel Digital Low Pass Filter Cutoff Frequency <CNFG_BIOZ Register> 00946 * @param Fcgen: BIOZ Current Generator Modulation Frequency <CNFG_BIOZ Register> 00947 * @param Cgmon: BIOZ Current Generator Monitor <CNFG_BIOZ Register> 00948 * @param Cgmag: BIOZ Current Generator Magnitude <CNFG_BIOZ Register> 00949 * @param Phoff: BIOZ Current Generator Modulation Phase Offset <CNFG_BIOZ Register> 00950 * @returns 0-if no error. A non-zero value indicates an error. 00951 * 00952 */ 00953 int BIOZ_InitStart(uint8_t En_bioz, uint8_t Openp, uint8_t Openn, 00954 uint8_t Calp_sel, uint8_t Caln_sel, 00955 uint8_t CG_mode, 00956 /* uint8_t En_bioz,*/ uint8_t B_fit, uint8_t Rate, 00957 uint8_t Ahpf, uint8_t Ext_rbias, uint8_t Gain, 00958 uint8_t Dhpf, uint8_t Dlpf, uint8_t Fcgen, 00959 uint8_t Cgmon, uint8_t Cgmag, uint8_t Phoff); 00960 00961 /** 00962 * @brief For MAX30001/2 ONLY 00963 * @brief This function disables the BIOZ. Uses Register CNFG_GEN-0x10. 00964 * @returns 0-if no error. A non-zero value indicates an error. 00965 * @returns 0-if no error. A non-zero value indicates an error. 00966 * 00967 */ 00968 int Stop_BIOZ(void); 00969 00970 /** 00971 * @brief For MAX30001/2 ONLY 00972 * @brief BIOZ modulated Resistance Built-in-Self-Test, Registers used: CNFG_BMUX-0x17 00973 * @param En_bist: Enable Modulated Resistance Built-in-Self-test <CNFG_BMUX Register> 00974 * @param Rnom: BIOZ RMOD BIST Nominal Resistance Selection <CNFG_BMUX Register> 00975 * @param Rmod: BIOZ RMOD BIST Modulated Resistance Selection <CNFG_BMUX Register> 00976 * @param Fbist: BIOZ RMOD BIST Frequency Selection <CNFG_BMUX Register> 00977 * @returns 0-if no error. A non-zero value indicates an error. 00978 * 00979 */ 00980 int BIOZ_InitBist(uint8_t En_bist, uint8_t Rnom, uint8_t Rmod, 00981 uint8_t Fbist); 00982 00983 /** 00984 * @brief For MAX30001/3/4 ONLY 00985 * @brief Sets up the device for RtoR measurement 00986 * @param EN_rtor: ECG RTOR Detection Enable <RTOR1 Register> 00987 * @param Wndw: R to R Window Averaging (Window Width = RTOR_WNDW[3:0]*8mS) <RTOR1 Register> 00988 * @param Gain: R to R Gain (where Gain = 2^RTOR_GAIN[3:0], plus an auto-scale option) <RTOR1 Register> 00989 * @param Pavg: R to R Peak Averaging Weight Factor <RTOR1 Register> 00990 * @param Ptsf: R to R Peak Threshold Scaling Factor <RTOR1 Register> 00991 * @param Hoff: R to R minimum Hold Off <RTOR2 Register> 00992 * @param Ravg: R to R Interval Averaging Weight Factor <RTOR2 Register> 00993 * @param Rhsf: R to R Interval Hold Off Scaling Factor <RTOR2 Register> 00994 * @param Clr_rrint: RTOR Detect Interrupt Clear behaviour <MNGR_INT Register> 00995 * @returns 0-if no error. A non-zero value indicates an error. 00996 * 00997 */ 00998 int RtoR_InitStart(uint8_t En_rtor, uint8_t Wndw, uint8_t Gain, 00999 uint8_t Pavg, uint8_t Ptsf, uint8_t Hoff, 01000 uint8_t Ravg, uint8_t Rhsf, uint8_t Clr_rrint); 01001 01002 /** 01003 * @brief For MAX30001/3/4 ONLY 01004 * @brief This function disables the RtoR. Uses Register CNFG_RTOR1-0x1D 01005 * @returns 0-if no error. A non-zero value indicates an error. 01006 * 01007 */ 01008 int Stop_RtoR(void); 01009 01010 /** 01011 * @brief This is a function that waits for the PLL to lock; once a lock is achieved it exits out. (For convenience only) 01012 * @returns 0-if no error. A non-zero value indicates an error. 01013 * 01014 */ 01015 int PLL_lock(void); 01016 01017 /** 01018 * @brief This function causes the MAX30001 to reset. Uses Register SW_RST-0x08 01019 * @return 0-if no error. A non-zero value indicates an error. 01020 * 01021 */ 01022 int sw_rst(void); 01023 01024 /** 01025 * @brief This function provides a SYNCH operation. Uses Register SYCNH-0x09. Please refer to the data sheet for 01026 * @brief the details on how to use this. 01027 * @returns 0-if no error. A non-zero value indicates an error. 01028 * 01029 */ 01030 int synch(void); 01031 01032 /** 01033 * @brief This function performs a FIFO Reset. Uses Register FIFO_RST-0x0A. Please refer to the data sheet 01034 * @brief for the details on how to use this. 01035 * @returns 0-if no error. A non-zero value indicates an error. 01036 */ 01037 int fifo_rst(void); 01038 01039 /** 01040 * 01041 * @brief This is a callback function which collects all the data from the ECG, BIOZ, PACE and RtoR. It also handles 01042 * @brief Lead On/Off. This function is passed through the argument of max30001_COMMinit(). 01043 * @returns 0-if no error. A non-zero value indicates an error. 01044 * 01045 */ 01046 int int_handler(void); 01047 01048 /** 01049 * @brief This is function called from the max30001_int_handler() function and processes all the ECG, BIOZ, PACE 01050 * @brief and the RtoR data and sticks them in appropriate arrays and variables each unsigned 32 bits. 01051 * @param ECG data will be in the array (input): max30001_ECG_FIFO_buffer[] 01052 * @param Pace data will be in the array (input): max30001_PACE[] 01053 * @param RtoRdata will be in the variable (input): max30001_RtoR_data 01054 * @param BIOZ data will be in the array (input): max30001_BIOZ_FIFO_buffer[] 01055 * @param global max30001_ECG_FIFO_buffer[] 01056 * @param global max30001_PACE[] 01057 * @param global max30001_BIOZ_FIFO_buffer[] 01058 * @param global max30001_RtoR_data 01059 * @param global max30001_DCLeadOff 01060 * @param global max30001_ACLeadOff 01061 * @param global max30001_LeadON 01062 * @returns 0-if no error. A non-zero value indicates an error. 01063 * 01064 */ 01065 int FIFO_LeadONOff_Read(void); 01066 01067 /** 01068 * @brief This function allows writing to a register. 01069 * @param addr: Address of the register to write to 01070 * @param data: 24-bit data read from the register. 01071 * @returns 0-if no error. A non-zero value indicates an error. 01072 * 01073 */ 01074 int reg_write(MAX30001_REG_map_t addr, uint32_t data); 01075 01076 /** 01077 * @brief This function allows reading from a register 01078 * @param addr: Address of the register to read from. 01079 * @param *return_data: pointer to the value read from the register. 01080 * @returns 0-if no error. A non-zero value indicates an error. 01081 * 01082 */ 01083 int reg_read(MAX30001_REG_map_t addr, uint32_t *return_data); 01084 01085 /** 01086 * @brief This function enables the DC Lead Off detection. Either ECG or BIOZ can be detected, one at a time. 01087 * @brief Registers Used: CNFG_GEN-0x10 01088 * @param En_dcloff: BIOZ Digital Lead Off Detection Enable 01089 * @param Ipol: DC Lead Off Current Polarity (if current sources are enabled/connected) 01090 * @param Imag: DC Lead off current Magnitude Selection 01091 * @param Vth: DC Lead Off Voltage Threshold Selection 01092 * @returns 0-if no error. A non-zero value indicates an error. 01093 * 01094 */ 01095 int Enable_DcLeadOFF_Init(int8_t En_dcloff, int8_t Ipol, int8_t Imag, 01096 int8_t Vth); 01097 01098 /** 01099 * @brief This function disables the DC Lead OFF feature, whichever is active. 01100 * @returns 0-if no error. A non-zero value indicates an error. 01101 * 01102 */ 01103 int Disable_DcLeadOFF(void); 01104 01105 /** 01106 * @brief This function sets up the BIOZ for AC Lead Off test. 01107 * @brief Registers Used: CNFG_GEN-0x10, MNGR_DYN-0x05 01108 * @param En_bloff: BIOZ Digital Lead Off Detection Enable <CNFG_GEN register> 01109 * @param Bloff_hi_it: DC Lead Off Current Polarity (if current sources are enabled/connected) <MNGR_DYN register> 01110 * @param Bloff_lo_it: DC Lead off current Magnitude Selection <MNGR_DYN register> 01111 * @returns 0-if no error. A non-zero value indicates an error. 01112 * 01113 */ 01114 int BIOZ_Enable_ACLeadOFF_Init(uint8_t En_bloff, uint8_t Bloff_hi_it, 01115 uint8_t Bloff_lo_it); 01116 01117 /** 01118 * @brief This function Turns of the BIOZ AC Lead OFF feature 01119 * @brief Registers Used: CNFG_GEN-0x10 01120 * @returns 0-if no error. A non-zero value indicates an error. 01121 * 01122 */ 01123 int BIOZ_Disable_ACleadOFF(void); 01124 01125 /** 01126 * @brief This function enables the Current Gnerator Monitor 01127 * @brief Registers Used: CNFG_BIOZ-0x18 01128 * @returns 0-if no error. A non-zero value indicates an error. 01129 * 01130 */ 01131 int BIOZ_Enable_BCGMON(void); 01132 01133 /** 01134 * 01135 * @brief This function enables the Lead ON detection. Either ECG or BIOZ can be detected, one at a time. 01136 * @brief Also, the en_bioz, en_ecg, en_pace setting is saved so that when this feature is disabled through the 01137 * @brief max30001_Disable_LeadON() function (or otherwise) the enable/disable state of those features can be retrieved. 01138 * @param Channel: ECG or BIOZ detection 01139 * @returns 0-if everything is good. A non-zero value indicates an error. 01140 * 01141 */ 01142 int Enable_LeadON(int8_t Channel); 01143 01144 /** 01145 * @brief This function turns off the Lead ON feature, whichever one is active. Also, retrieves the en_bioz, 01146 * @brief en_ecg, en_pace and sets it back to as it was. 01147 * @param 0-if everything is good. A non-zero value indicates an error. 01148 * 01149 */ 01150 int Disable_LeadON(void); 01151 01152 /** 01153 * 01154 * @brief This function is toggled every 2 seconds to switch between ECG Lead ON and BIOZ Lead ON detect 01155 * @brief Adjust LEADOFF_SERVICE_TIME to determine the duration between the toggles. 01156 * @param CurrentTime - This gets fed the time by RTC_GetValue function 01157 * 01158 */ 01159 void ServiceLeadON(uint32_t currentTime); 01160 01161 /** 01162 * 01163 * @brief This function is toggled every 2 seconds to switch between ECG DC Lead Off and BIOZ DC Lead Off 01164 * @brief Adjust LEADOFF_SERVICE_TIME to determine the duration between the toggles. 01165 * @param CurrentTime - This gets fed the time by RTC_GetValue function 01166 * 01167 */ 01168 void ServiceLeadoff(uint32_t currentTime); 01169 01170 /** 01171 * 01172 * @brief This function sets current RtoR values and fmstr values in a pointer structure 01173 * @param hspValMax30001 - Pointer to a structure where to store the values 01174 * 01175 */ 01176 void ReadHeartrateData(max30001_bledata_t *_hspValMax30001); 01177 01178 /** 01179 * @brief type definition for data interrupt 01180 */ 01181 typedef void (*PtrFunction)(uint32_t id, uint32_t *buffer, uint32_t length); 01182 01183 /** 01184 * @brief Used to connect a callback for when interrupt data is available 01185 */ 01186 void onDataAvailable(PtrFunction _onDataAvailable); 01187 01188 01189 01190 /** 01191 * @brief Preventive measure used to dismiss interrupts that fire too early during 01192 * @brief initialization on INTB line 01193 * 01194 */ 01195 static void Mid_IntB_Handler(void); 01196 01197 /** 01198 * @brief Preventive measure used to dismiss interrupts that fire too early during 01199 * @brief initialization on INT2B line 01200 * 01201 */ 01202 static void Mid_Int2B_Handler(void); 01203 01204 /** 01205 * @brief Allows Interrupts to be accepted as valid. 01206 * @param state: 1-Allow interrupts, Any-Don't allow interrupts. 01207 * 01208 */ 01209 void AllowInterrupts(int state); 01210 01211 01212 /// @brief function pointer to the async callback 01213 static event_callback_t functionpointer; 01214 01215 /// @brief flag used to indicate an async xfer has taken place 01216 static volatile int xferFlag; 01217 01218 /** 01219 * @brief Callback handler for SPI async events 01220 * @param events description of event that occurred 01221 */ 01222 static void spiHandler(int events); 01223 01224 01225 static MAX30001 *instance; 01226 01227 private: 01228 01229 /** 01230 * @brief Used to notify an external function that interrupt data is available 01231 * @param id type of data available 01232 * @param buffer 32-bit buffer that points to the data 01233 * @param length length of 32-bit elements available 01234 */ 01235 void dataAvailable(uint32_t id, uint32_t *buffer, uint32_t length); 01236 01237 /** 01238 * @brief Transmit and recieve QUAD SPI data 01239 * @param tx_buf pointer to transmit byte buffer 01240 * @param tx_size number of bytes to transmit 01241 * @param rx_buf pointer to the recieve buffer 01242 * @param rx_size number of bytes to recieve 01243 */ 01244 int SPI_Transmit(const uint8_t *tx_buf, uint32_t tx_size, uint8_t *rx_buf, 01245 uint32_t rx_size); 01246 01247 /// pointer to mbed SPI object 01248 SPI *spi; 01249 /// is this object the owner of the spi object 01250 bool spi_owner; 01251 /// buffer to use for async transfers 01252 uint8_t buffer[ASYNC_SPI_BUFFER_SIZE]; 01253 /// function pointer to the async callback 01254 // event_callback_t functionpointer; 01255 /// callback function when interrupt data is available 01256 PtrFunction onDataAvailableCallback; 01257 01258 }; // End of MAX30001 Class 01259 01260 01261 #endif /* MAX30001_H_ */
Generated on Tue Jul 12 2022 17:59:19 by
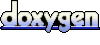