
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
Logging_RPC.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ****************************************************************************** 00032 */ 00033 #include "StringHelper.h" 00034 #include <stdint.h> 00035 #include "Streaming.h" 00036 #include "StringInOut.h" 00037 #include "DataLoggingService.h" 00038 #include "Peripherals.h" 00039 #include "Logging.h" 00040 #include "S25FS512.h" 00041 00042 extern char loggingMissionCmds[4096]; 00043 uint32_t missionCmdIndex; 00044 00045 //****************************************************************************** 00046 int Logging_RPC_StartMissionDefine(char argStrs[32][32], 00047 char replyStrs[32][32]) { 00048 uint32_t i; 00049 uint32_t reply[1]; 00050 00051 // reset the missionCmdIndex to the beginning of the cmd buffer 00052 missionCmdIndex = 0; 00053 // clear the mission command buffer, fill with zeros 00054 for (i = 0; i < sizeof(loggingMissionCmds); i++) { 00055 loggingMissionCmds[i] = 0; 00056 } 00057 00058 reply[0] = 0x80; 00059 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00060 return 0; 00061 } 00062 00063 //****************************************************************************** 00064 int Logging_RPC_AppendMissionCmd(char argStrs[32][32], char replyStrs[32][32]) { 00065 uint32_t reply[1]; 00066 char *strPtr; 00067 uint32_t count = 0; 00068 uint8_t result = 0x80; 00069 // append the string to the mission cmd log 00070 strPtr = argStrs[0]; 00071 while (*strPtr != 0) { 00072 loggingMissionCmds[missionCmdIndex] = *strPtr; 00073 missionCmdIndex++; 00074 strPtr++; 00075 // do not overrun buffer 00076 if (missionCmdIndex > (sizeof(loggingMissionCmds) - 2)) { 00077 result = 0xFF; 00078 break; 00079 } 00080 count++; 00081 // do not read more than max count in incoming string 00082 if (count > (32 * 32)) { 00083 result = 0xFF; 00084 break; 00085 } 00086 } 00087 if (result != 0x80) { 00088 reply[0] = 0xFF; 00089 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00090 return 0; 00091 } 00092 // add cr/lf to the end of this cmd string 00093 loggingMissionCmds[missionCmdIndex++] = 13; 00094 loggingMissionCmds[missionCmdIndex++] = 10; 00095 00096 reply[0] = 0x80; 00097 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00098 return 0; 00099 } 00100 00101 //****************************************************************************** 00102 int Logging_RPC_EndMissionDefine(char argStrs[32][32], char replyStrs[32][32]) { 00103 uint32_t reply[1]; 00104 reply[0] = 0x80; 00105 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00106 return 0; 00107 } 00108 00109 //****************************************************************************** 00110 int Logging_RPC_WriteMission(char argStrs[32][32], char replyStrs[32][32]) { 00111 uint8_t page; 00112 char *ptr; 00113 uint32_t reply[1]; 00114 00115 Peripherals::s25FS512()->parameterSectorErase_Helper(0x0); 00116 ptr = loggingMissionCmds; 00117 for (page = 0; page < 16; page++) { 00118 Peripherals::s25FS512()->writePage_Helper(page, (uint8_t *)ptr, 0); 00119 ptr += 256; 00120 } 00121 00122 reply[0] = 0x80; 00123 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00124 return 0; 00125 } 00126 00127 //****************************************************************************** 00128 int Logging_RPC_ReadMission(char argStrs[32][32], char replyStrs[32][32]) { 00129 char *ptr; 00130 uint32_t i; 00131 // read sector 0 00132 ptr = loggingMissionCmds; 00133 for (i = 0; i < 16; i++) { 00134 Peripherals::s25FS512()->readPages_Helper(i, i, (uint8_t *)ptr, 0); 00135 ptr += 256; 00136 } 00137 // strip header by shifting payload left 00138 ptr = loggingMissionCmds; 00139 for (i = 0; i < sizeof(loggingMissionCmds); i++) { 00140 if (*ptr == 13) { 00141 *ptr = ':'; 00142 } else if (*ptr == 10) { 00143 *ptr = ' '; 00144 } 00145 ptr++; 00146 } 00147 if (loggingMissionCmds[0] == 0xFF || loggingMissionCmds[0] == 0x0) { 00148 sprintf(loggingMissionCmds, "%s", "null "); 00149 } 00150 // send it out via uart 00151 putStr(loggingMissionCmds); 00152 replyStrs[0][0] = 0; 00153 return 0; 00154 } 00155 00156 //****************************************************************************** 00157 int Logging_RPC_EraseMission(char argStrs[32][32], char replyStrs[32][32]) { 00158 uint32_t reply[1]; 00159 Peripherals::s25FS512()->parameterSectorErase_Helper(0x0); 00160 reply[0] = 0x80; 00161 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00162 return 0; 00163 } 00164 00165 // 00166 // small helper function to determine if the X number of a page are FF (empty) 00167 // 00168 static bool isPartialPageEmpty(uint8_t *ptr, int count) { 00169 int i; 00170 for (i = 0; i < count; i++) { 00171 if (ptr[i] != 0xFF) return false; 00172 } 00173 return true; 00174 } 00175 00176 // 00177 // define the different sector sizes in flash 00178 // 00179 typedef enum { 00180 e4K, 00181 e32K, 00182 e64K 00183 } loggingFlashArea_t; 00184 00185 //****************************************************************************** 00186 int Logging_EraseWrittenSectors(char argStrs[32][32], char replyStrs[32][32]) { 00187 uint32_t i; 00188 uint8_t data[512]; 00189 uint32_t address; 00190 uint32_t pageNumber; 00191 bool pageEmpty; 00192 uint32_t pagesToScan; 00193 loggingFlashArea_t currentArea; 00194 uint32_t currentAreaSize; 00195 //Peripherals::s25FS512()->bulkErase_Helper(); 00196 00197 // 00198 // quickly scan through the first few bytes of a page and continue this through a sector 00199 // this will determine if the sector needs to be erased or can be skipped 00200 // 00201 currentArea = e4K; 00202 address = 0; 00203 // interate through the entire flash 00204 while (address < SIZE_OF_EXTERNAL_FLASH) { 00205 // determine the size of the current area 00206 switch (currentArea) { 00207 case e4K: 00208 currentAreaSize = ADDRESS_INC_4K; 00209 break; 00210 case e32K: 00211 currentAreaSize = ADDRESS_INC_32K; 00212 break; 00213 default: 00214 currentAreaSize = ADDRESS_INC_64K; 00215 break; 00216 } 00217 pagesToScan = currentAreaSize / SIZE_OF_PAGE; 00218 pageNumber = address >> 8; 00219 pageEmpty = true; 00220 // scan the first few bytes in each page for this area 00221 for (i = 0; i < pagesToScan; i++) { 00222 Peripherals::s25FS512()->readPartialPage_Helper(pageNumber + i, data, 4); 00223 pageEmpty = isPartialPageEmpty(data, 4); 00224 if (pageEmpty == false) break; 00225 } 00226 // if we detected data then erase this sector 00227 if (pageEmpty == false) { 00228 switch (currentArea) { 00229 case e4K: 00230 Peripherals::s25FS512()->parameterSectorErase_Helper(address); 00231 break; 00232 default: 00233 Peripherals::s25FS512()->sectorErase_Helper(address); 00234 break; 00235 } 00236 } 00237 // update the address with the current area size 00238 address += currentAreaSize; 00239 // determine the new area in flash 00240 if (address < ADDRESS_4K_END) { 00241 currentArea = e4K; 00242 } else if (address == ADDRESS_32K_START) { 00243 currentArea = e32K; 00244 } else { 00245 currentArea = e64K; 00246 } 00247 } 00248 return 0; 00249 } 00250 00251 //****************************************************************************** 00252 int Logging_Start(char argStrs[32][32], char replyStrs[32][32]) { 00253 uint32_t reply[1]; 00254 Logging_SetStart(true); 00255 reply[0] = 0x80; // indicate success 00256 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00257 return 0; 00258 } 00259 00260 //****************************************************************************** 00261 int Logging_GetLastWrittenPage(char argStrs[32][32], char replyStrs[32][32]) { 00262 uint32_t reply[1]; 00263 00264 uint32_t page; 00265 uint32_t lastPage; 00266 uint32_t pageEmpty; 00267 uint8_t data[512]; 00268 00269 printf("Logging_GetLastWrittenPage "); 00270 fflush(stdout); 00271 lastPage = Logging_GetLoggingEndPage(); 00272 for (page = 2; page <= lastPage; page++) { 00273 // Peripherals::serial()->printf("checking page %d ",page); fflush(stdout); 00274 // sample the page 00275 Peripherals::s25FS512()->readPages_Helper(page, page, data, 0); 00276 pageEmpty = Peripherals::s25FS512()->isPageEmpty(data); 00277 if (pageEmpty != 0) { 00278 break; 00279 } 00280 } 00281 if (page > lastPage) 00282 page = lastPage; 00283 printf("last page %d, 0x%X ", (unsigned int)page, (unsigned int)page); 00284 fflush(stdout); 00285 reply[0] = page; 00286 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00287 return 0; 00288 } 00289 00290 extern int highDataRate; 00291 //****************************************************************************** 00292 int Logging_StartLoggingUsb(char argStrs[32][32], char replyStrs[32][32]) { 00293 uint32_t reply[1]; 00294 // highDataRate = 0; 00295 LoggingService_StartLoggingUsb(); 00296 reply[0] = 0x80; 00297 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00298 return 0; 00299 } 00300 //****************************************************************************** 00301 int Logging_StartLoggingFlash(char argStrs[32][32], char replyStrs[32][32]) { 00302 uint32_t reply[1]; 00303 // highDataRate = 0; 00304 LoggingService_StartLoggingFlash(); 00305 reply[0] = 0x80; 00306 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00307 return 0; 00308 }
Generated on Tue Jul 12 2022 17:59:19 by
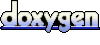