
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
LIS2DH_RPC.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include "mbed.h" 00035 #include "LIS2DH.h" 00036 #include "StringInOut.h" 00037 #include "StringHelper.h" 00038 #include "Peripherals.h" 00039 00040 #define LIS2DH_SLAVE_ADDRESS 0x32 00041 #define LIS2DH_REG_PART_ID 0x0F 00042 00043 //****************************************************************************** 00044 int LIS2DH_ReadReg(char argStrs[32][32], char replyStrs[32][32]) { 00045 uint8_t args[1]; 00046 uint8_t reply[1]; 00047 ProcessArgs(argStrs, args, sizeof(args)); 00048 LIS2DH *lis2dh = Peripherals::lis2dh(); 00049 lis2dh->readReg((LIS2DH::LIS2DH_REG_map_t)args[0], (char *)reply); 00050 FormatReply(reply, sizeof(reply), replyStrs); 00051 return 0; 00052 } 00053 00054 //****************************************************************************** 00055 int LIS2DH_WriteReg(char argStrs[32][32], char replyStrs[32][32]) { 00056 uint8_t args[2]; 00057 uint8_t reply[1]; 00058 ProcessArgs(argStrs, args, sizeof(args)); 00059 LIS2DH *lis2dh = Peripherals::lis2dh(); 00060 lis2dh->writeReg((LIS2DH::LIS2DH_REG_map_t)args[0], args[1]); ///< pass in the register address 00061 reply[0] = 0x80; 00062 FormatReply(reply, sizeof(reply), replyStrs); 00063 return 0; 00064 } 00065 00066 extern int highDataRate; 00067 //****************************************************************************** 00068 int LIS2DH_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00069 uint8_t args[2]; 00070 uint8_t reply[1]; 00071 ProcessArgs(argStrs, args, sizeof(args)); 00072 LIS2DH *lis2dh = Peripherals::lis2dh(); 00073 if (args[0] >= LIS2DH_DATARATE_200HZ) { 00074 highDataRate = 1; 00075 } 00076 lis2dh->initStart(args[0], args[1]); 00077 reply[0] = 0x80; 00078 FormatReply(reply, sizeof(reply), replyStrs); 00079 return 0; 00080 } 00081 00082 //****************************************************************************** 00083 int LIS2DH_Stop(char argStrs[32][32], char replyStrs[32][32]) { 00084 uint8_t reply[1]; 00085 LIS2DH *lis2dh = Peripherals::lis2dh(); 00086 lis2dh->stop(); 00087 reply[0] = 0x80; 00088 FormatReply(reply, sizeof(reply), replyStrs); 00089 return 0; 00090 }
Generated on Tue Jul 12 2022 17:59:19 by
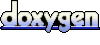