
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
LIS2DH.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include "LIS2DH.h" 00035 #include "Streaming.h" 00036 #include "Peripherals.h" 00037 00038 void lis2dh_int_handler(void); 00039 /** 00040 * @brief buffer array to hold fifo contents for packetizing 00041 */ 00042 uint32_t lis2dh_buffer[LIS2DH_MAX_DATA_SIZE]; 00043 00044 int16_t motion_cached[3]; 00045 LIS2DH *LIS2DH::instance = NULL; 00046 00047 //****************************************************************************** 00048 LIS2DH::LIS2DH(PinName sda, PinName scl, int slaveAddress) : 00049 slaveAddress(slaveAddress) { 00050 i2c = new I2C(sda, scl); 00051 i2c->frequency(400000); 00052 isOwner = true; 00053 instance = this; 00054 } 00055 00056 //****************************************************************************** 00057 LIS2DH::LIS2DH(I2C *i2c, int slaveAddress) : 00058 slaveAddress(slaveAddress) { 00059 this->i2c = i2c; 00060 i2c->frequency(400000); 00061 isOwner = false; 00062 instance = this; 00063 } 00064 00065 //****************************************************************************** 00066 LIS2DH::~LIS2DH(void) { 00067 if (isOwner == true) { 00068 delete i2c; 00069 } 00070 } 00071 00072 //****************************************************************************** 00073 int LIS2DH::writeReg(LIS2DH_REG_map_t reg, char value) { 00074 int result; 00075 char cmdData[2] = {(char)reg, value}; 00076 result = i2c->write(slaveAddress, cmdData, 2); 00077 if (result != 0) { 00078 return -1; 00079 } 00080 return 0; 00081 } 00082 00083 //****************************************************************************** 00084 int LIS2DH::readReg(LIS2DH_REG_map_t reg, char *value) { 00085 int result; 00086 char cmdData[1] = {(char)reg}; 00087 00088 result = i2c->write(slaveAddress, cmdData, 1); 00089 if (result != 0) { 00090 return -1; 00091 } 00092 result = i2c->read(slaveAddress, value, 1); 00093 if (result != 0) { 00094 return -1; 00095 } 00096 return 0; 00097 } 00098 00099 //****************************************************************************** 00100 static void I2c_Reset(uint8_t index, int speed) { 00101 mxc_i2cm_regs_t *regs = MXC_I2CM_GET_I2CM(index); 00102 /* reset module */ 00103 regs->ctrl = MXC_F_I2CM_CTRL_MSTR_RESET_EN; 00104 regs->ctrl = 0; 00105 /* enable tx_fifo and rx_fifo */ 00106 regs->ctrl |= (MXC_F_I2CM_CTRL_TX_FIFO_EN | MXC_F_I2CM_CTRL_RX_FIFO_EN); 00107 } 00108 00109 //****************************************************************************** 00110 /// Interrupt handler, this empties the hardware fifo and packetizes it for 00111 /// streaming 00112 void LIS2DH::int_handler(void) { 00113 char fifo_src; 00114 int16_t valueX; 00115 int16_t valueY; 00116 int16_t valueZ; 00117 int num; 00118 int index; 00119 00120 I2c_Reset(2, 1); 00121 num = 0; 00122 index = 0; 00123 fifo_src = 0; 00124 while ((fifo_src & 0x20) != 0x20) { 00125 get_motion_fifo(&valueX, &valueY, &valueZ); 00126 lis2dh_buffer[index++] = valueX; 00127 lis2dh_buffer[index++] = valueY; 00128 lis2dh_buffer[index++] = valueZ; 00129 readReg(LIS2DH_FIFO_SRC_REG, &fifo_src); 00130 num++; 00131 if (num >= 32) { 00132 break; 00133 } 00134 } 00135 motion_cached[0] = valueX; 00136 motion_cached[1] = valueY; 00137 motion_cached[2] = valueZ; 00138 00139 StreamPacketUint32(PACKET_LIS2DH, lis2dh_buffer, index); 00140 } 00141 00142 //****************************************************************************** 00143 void LIS2DH::init(void) { 00144 stop(); 00145 configure_interrupt(); 00146 } 00147 00148 //****************************************************************************** 00149 void LIS2DH::configure_interrupt(void) { 00150 00151 lis2dh_ctrl_reg6_t ctrl_reg6; 00152 ///< interrupt enabled on INT1, interrupt active low 00153 ctrl_reg6.all = 0; 00154 ctrl_reg6.bit.I2_INT1 = 1; ///< interrupt 1 function enabled on int1 pin 00155 ctrl_reg6.bit.H_LACTIVE = 1; ///< interrupt active low 00156 writeReg(LIS2DH_CTRL_REG6, ctrl_reg6.all); 00157 } 00158 00159 //****************************************************************************** 00160 int LIS2DH::initStart(int dataRate, int fifoThreshold) { 00161 00162 lis2dh_ctrl_reg5_t ctrl_reg5; 00163 lis2dh_fifo_ctrl_reg_t fifo_ctrl_reg; 00164 lis2dh_ctrl_reg1_t ctrl_reg1; 00165 lis2dh_ctrl_reg3_t ctrl_reg3; 00166 00167 __disable_irq(); 00168 00169 configure_interrupt(); 00170 /// 00171 /// enable FIFO 00172 /// 00173 ctrl_reg5.all = 0x0; 00174 ctrl_reg5.bit.FIFO_EN = 0x1; 00175 if (writeReg(LIS2DH_CTRL_REG5, ctrl_reg5.all) == -1) { 00176 __enable_irq(); 00177 return -1; 00178 } 00179 00180 /// 00181 /// set FIFO to stream mode, trigger select INT1 00182 /// 00183 fifo_ctrl_reg.all = 0x0; 00184 fifo_ctrl_reg.bit.FTH = fifoThreshold; 00185 fifo_ctrl_reg.bit.FM = LIS2DH_FIFOMODE_STREAM; 00186 fifo_ctrl_reg.bit.TR = 0x0; 00187 if (writeReg(LIS2DH_FIFO_CTRL_REG, fifo_ctrl_reg.all) == -1) { 00188 __enable_irq(); 00189 return -1; 00190 } 00191 00192 /// 00193 /// set HR (high resolution) 00194 /// 00195 if (writeReg(LIS2DH_CTRL_REG4, 0x8) == -1) { 00196 __enable_irq(); 00197 return -1; 00198 } 00199 00200 /// 00201 /// set the data rate, enable all axis 00202 /// 00203 dataRate = dataRate & 0xF; 00204 if (dataRate > 0x9) { 00205 dataRate = 0x9; 00206 } 00207 00208 ctrl_reg1.bit.ODR = dataRate; ///< set the data rate 00209 ctrl_reg1.bit.LPen = 0x0; ///< disable low power mode 00210 ctrl_reg1.bit.Zen = 0x1; ///< enable z 00211 ctrl_reg1.bit.Yen = 0x1; ///< enable y 00212 ctrl_reg1.bit.Xen = 0x1; ///< enable x 00213 if (writeReg(LIS2DH_CTRL_REG1, ctrl_reg1.all) == -1) { 00214 __enable_irq(); 00215 return -1; 00216 } 00217 00218 /// 00219 /// enable watermark interrupt 00220 /// 00221 ctrl_reg3.all = 0x00; 00222 ctrl_reg3.bit.I1_WTM = 0x1; 00223 if (writeReg(LIS2DH_CTRL_REG3, ctrl_reg3.all) == -1) { 00224 __enable_irq(); 00225 return -1; 00226 } 00227 __enable_irq(); 00228 00229 return 0; 00230 } 00231 00232 //****************************************************************************** 00233 int LIS2DH::detect(char *detected) { 00234 char val; 00235 *detected = 0; 00236 if (readReg(LIS2DH_WHO_AM_I, &val) == -1) { 00237 return -1; 00238 } 00239 00240 if (val == LIS2DH_ID) { 00241 *detected = 1; 00242 } 00243 00244 return 0; 00245 } 00246 00247 //****************************************************************************** 00248 int LIS2DH::get_motion_cached(int16_t *valueX, int16_t *valueY, 00249 int16_t *valueZ) { 00250 *valueX = motion_cached[0]; 00251 *valueY = motion_cached[1]; 00252 *valueZ = motion_cached[2]; 00253 return 0; 00254 } 00255 00256 //****************************************************************************** 00257 int LIS2DH::get_motion_fifo(int16_t *valueX, int16_t *valueY, int16_t *valueZ) { 00258 char reg = LIS2DH_OUT_X_L | 0x80; 00259 char values[6]; 00260 int i; 00261 00262 reg = LIS2DH_OUT_X_L; 00263 for (i = 0; i < 6; i++) { 00264 if (readReg((LIS2DH_REG_map_t)reg, &values[i]) != 0) { 00265 return -1; 00266 } 00267 reg++; 00268 } 00269 00270 *valueX = ((short)values[1] << 8) + values[0]; 00271 *valueY = ((short)values[3] << 8) + values[2]; 00272 *valueZ = ((short)values[5] << 8) + values[4]; 00273 motion_cached[0] = *valueX; 00274 motion_cached[1] = *valueY; 00275 motion_cached[2] = *valueZ; 00276 return 0; 00277 } 00278 00279 //****************************************************************************** 00280 char LIS2DH::readId(void) { 00281 char val; 00282 readReg(LIS2DH_WHO_AM_I, &val); 00283 return val; 00284 } 00285 00286 //****************************************************************************** 00287 void LIS2DH::stop(void) { 00288 __disable_irq(); 00289 writeReg(LIS2DH_CTRL_REG3, 0x00); // Disable watermark interrupt 00290 writeReg(LIS2DH_CTRL_REG1, 0x00); // Data rate = 0Hz 00291 writeReg(LIS2DH_FIFO_CTRL_REG, 00292 0x00); // set to bypass mode... clears FIFO_SRC_REG 00293 __enable_irq(); 00294 } 00295 00296 //****************************************************************************** 00297 void LIS2DHIntHandler(void) { 00298 char value; 00299 /// 00300 /// read the data rate axis enable register, if this is zero then just return, 00301 /// we are not ready for interrupts 00302 /// 00303 LIS2DH::instance->readReg(LIS2DH::LIS2DH_CTRL_REG1, &value); 00304 if (value == 0x0) { 00305 return; 00306 } 00307 LIS2DH::instance->int_handler(); 00308 }
Generated on Tue Jul 12 2022 17:59:19 by
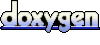