
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
I2C_RPC.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include <stdint.h> 00034 #include "StringHelper.h" 00035 #include "I2C_RPC.h" 00036 #include "Peripherals.h" 00037 00038 //****************************************************************************** 00039 // input 00040 // [instance] [slaveAddress] [writeNumber] [](data to write) [readNumber] 00041 // output data to read 00042 int I2C_WriteRead(char argStrs[32][32], char replyStrs[32][32]) { 00043 uint8_t args[16]; 00044 uint8_t reply[16]; 00045 uint8_t writeNumber; 00046 uint8_t readNumber; 00047 uint8_t instance; 00048 uint8_t slaveAddress; 00049 int ret1; 00050 int ret2; 00051 I2C *i2c; 00052 // get the number of bytes to write 00053 ProcessArgs(argStrs, args, 3); // parse [instance] [slaveAddress] [writeNumber] 00054 instance = args[0]; 00055 slaveAddress = args[1]; 00056 writeNumber = args[2]; 00057 // parse [instance] [slaveAddress] [writeNumber] [](data to write) 00058 // [readNumber] 00059 ProcessArgs(argStrs, args, writeNumber + 4); 00060 readNumber = args[writeNumber + 3]; 00061 00062 i2c = Peripherals::i2c1(); 00063 if (instance == 2) { 00064 i2c = Peripherals::i2c2(); 00065 } 00066 ret1 = 0; 00067 ret2 = 0; 00068 00069 if (writeNumber != 0) { 00070 ret1 = i2c->write((int)slaveAddress, (char *)&args[3], (int)writeNumber); 00071 } 00072 if (readNumber != 0) { 00073 ret2 = i2c->read((int)slaveAddress, (char *)reply, (int)readNumber); 00074 } 00075 00076 if (ret1 != 0) reply[0] = 0xFF; 00077 if (ret2 != 0) reply[0] = 0xFF; 00078 00079 00080 // reply[0] = 0x80; 00081 FormatReply(reply, readNumber, replyStrs); 00082 return 0; 00083 }
Generated on Tue Jul 12 2022 17:59:19 by
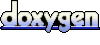