
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
HspLed.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include "HspLed.h" 00035 #include "Peripherals.h" 00036 00037 void hspled_int_handler(void); 00038 00039 //******************************************************************************* 00040 HspLed::HspLed(PinName ledPin) : 00041 timerInterval(500), timerIntervalLast(-1), redLed(LED_RED, 0), isStarted(false) { 00042 } 00043 00044 //******************************************************************************* 00045 void HspLed::state(int state) { 00046 redLed.write(state); 00047 } 00048 00049 //******************************************************************************* 00050 void HspLed::toggle(void) { 00051 state(!redLed.read()); 00052 } 00053 00054 //******************************************************************************* 00055 void HspLed::setMode(eMode mode) { 00056 this->mode = mode; 00057 } 00058 00059 //******************************************************************************* 00060 void HspLed::blink(uint32_t mSeconds) { 00061 mode = eLedPeriod; 00062 this->timerInterval = (float)mSeconds / 1000.0f; 00063 start(); 00064 } 00065 00066 //******************************************************************************* 00067 void HspLed::pattern(uint32_t bitPattern, uint32_t mSeconds) { 00068 mode = eLedPattern; 00069 this->bitPattern = bitPattern; 00070 this->timerInterval = (float)mSeconds / 1000.0f; 00071 start(); 00072 } 00073 00074 //******************************************************************************* 00075 void HspLed::on(void) { 00076 mode = eLedOn; 00077 state(HSP_LED_ON); 00078 start(); 00079 } 00080 00081 //******************************************************************************* 00082 void HspLed::off(void) { 00083 mode = eLedOff; 00084 state(HSP_LED_OFF); 00085 start(); 00086 } 00087 00088 //******************************************************************************* 00089 void HspLed::patternToLed(void) { 00090 uint32_t bit; 00091 bit = bitPattern & 1; 00092 state(bit); 00093 // rotate the pattern 00094 bitPattern = bitPattern >> 1; 00095 bitPattern = bitPattern | (bit << 31); 00096 } 00097 00098 //******************************************************************************* 00099 void HspLed::service(void) { 00100 switch (mode) { 00101 case eLedOn: 00102 state(HSP_LED_ON); 00103 break; 00104 case eLedOff: 00105 state(HSP_LED_OFF); 00106 break; 00107 case eLedPeriod: 00108 toggle(); 00109 break; 00110 case eLedPattern: 00111 patternToLed(); 00112 break; 00113 } 00114 } 00115 00116 //******************************************************************************* 00117 void HspLed::start(void) { 00118 if (timerInterval != timerIntervalLast && isStarted == true) { 00119 stop(); 00120 } 00121 ticker.attach(&hspled_int_handler, timerInterval); 00122 timerIntervalLast = timerInterval; 00123 00124 isStarted = true; 00125 } 00126 00127 //******************************************************************************* 00128 void HspLed::stop(void) { 00129 ticker.detach(); 00130 isStarted = false; 00131 } 00132 00133 //******************************************************************************* 00134 void hspled_int_handler(void) { 00135 HspLed *hspLed = Peripherals::hspLed(); 00136 hspLed->service(); 00137 }
Generated on Tue Jul 12 2022 17:59:19 by
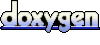