
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
Characteristic.h
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #ifndef _CHARACTERISTIC_H_ 00034 #define _CHARACTERISTIC_H_ 00035 00036 #include "mbed.h" 00037 #include "BLE.h" 00038 #include "GattCharacteristic.h" 00039 00040 class Characteristic { 00041 public: 00042 /** 00043 * @brief ticker handler static method 00044 */ 00045 Characteristic(uint16_t length, const UUID &uuid, 00046 uint8_t additionalProperties = 00047 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NONE, 00048 GattAttribute *descriptors[] = NULL, 00049 unsigned numDescriptors = 0); 00050 00051 void setIndex(int index) { this->index = index; } 00052 /** 00053 * @brief Set a pointer reference to the mbed BLE framework 00054 */ 00055 void setBLE(BLE *ble) { this->ble = ble; } 00056 /** 00057 * @brief Get the pointer to the byte payload 00058 */ 00059 uint8_t *getPayloadPtr(void) { return payload.getPointer(); } 00060 /** 00061 * @brief Get the handle of the characteristic 00062 */ 00063 GattAttribute::Handle_t getValueHandle(); 00064 /** 00065 * @brief Update the characteristic 00066 */ 00067 void update(void); 00068 /** 00069 * @brief Get the GattCharacteristic 00070 */ 00071 GattCharacteristic *getGattCharacteristic(); 00072 /** 00073 * @brief Get the length of the payload 00074 */ 00075 int getPayloadLength(void); 00076 00077 uint8_t *getPayloadBytes(void) { return payload.bytes; } 00078 00079 void copyDataWritten(const GattWriteCallbackParams *params) { 00080 unsigned int i = 0; 00081 while (i < params->len && i < sizeof(dataWritten)) { 00082 dataWritten[i] = params->data[i]; 00083 i++; 00084 } 00085 dataWrittenLength = params->len; 00086 } 00087 00088 uint8_t *getDataWritten(int *length) { 00089 *length = dataWrittenLength; 00090 return dataWritten; 00091 } 00092 00093 private: 00094 int payloadLength; 00095 struct PayloadStruct { 00096 uint8_t bytes[32]; 00097 uint8_t *getPointer(void) { return bytes; } 00098 }; 00099 /// local copy of data written to this characteristic 00100 uint8_t dataWritten[64]; 00101 int dataWrittenLength; 00102 /// payload structure for this characteristic 00103 PayloadStruct payload; 00104 /// gatt characteristic 00105 GattCharacteristic gattCharacteristic; 00106 /// mbed BLE framework pointer 00107 BLE *ble; 00108 /// index of this characteristic 00109 int index; 00110 }; 00111 00112 #endif /* _CHARACTERISTIC_H_ */
Generated on Tue Jul 12 2022 17:59:19 by
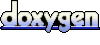