
Program to demonstrate how to configure the PMIC on the MAX32620HSP (MAXREFDES100#)
Fork of HSP_PMIC_Demo by
main.cpp
00001 #include "mbed.h" 00002 #include "MAX14720.h" 00003 00004 // I2C Master 2 00005 I2C i2c2(I2C2_SDA, I2C2_SCL); 00006 00007 #define I2C_ADDR_PMIC (0x54) 00008 MAX14720 max14720(&i2c2,I2C_ADDR_PMIC); 00009 00010 DigitalOut led(LED1); 00011 InterruptIn button(SW1); 00012 00013 void turnOff() 00014 { 00015 // Write the power down command to the PMIC 00016 max14720.shutdown(); 00017 } 00018 00019 int main() 00020 { 00021 // Assign turnOff function to falling edge of button 00022 button.fall(&turnOff); 00023 00024 // Turn LED signal on to make buck-boost output visible 00025 led = 0; 00026 00027 // Override the default value of boostEn to BOOST_ENABLED 00028 max14720.boostEn = MAX14720::BOOST_ENABLED; 00029 // Note that writing the local value does directly affect the part 00030 // The buck-boost regulator will remain off until init is called 00031 00032 // Call init to apply all settings to the PMIC 00033 if (max14720.init() == MAX14720_ERROR) { 00034 printf("Error initializing MAX14720"); 00035 } 00036 00037 // Wait 1 second to see the buck-boost regulator turn on 00038 wait(1); 00039 00040 while(1) { 00041 // Turn off the buck-boost regulators 00042 max14720.boostSetMode(MAX14720::BOOST_DISABLED); 00043 wait(0.5); 00044 00045 // Change the voltage of the buck-boost regulator and enable it 00046 max14720.boostSetVoltage(2500); 00047 max14720.boostSetMode(MAX14720::BOOST_ENABLED); 00048 wait(0.5); 00049 00050 // Change the voltage of the buck-boost regulator 00051 // Note that the MAX14720 cannot change the buck-boost voltage while 00052 // it is enabled so if boostEn is set to BOOST_ENABLED, this 00053 // function will disable it, change the voltage, and re-enable it. 00054 max14720.boostSetVoltage(5000); 00055 wait(0.5); 00056 } 00057 }
Generated on Thu Jul 14 2022 08:59:31 by
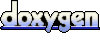