
DeepCover Embedded Security in IoT: Public-key Secured Data Paths
Dependencies: MaximInterface
nonos.c
00001 /* 00002 * nonos.c - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2015 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 00038 00039 /*****************************************************************************/ 00040 /* Include files */ 00041 /*****************************************************************************/ 00042 #include "simplelink.h" 00043 #include "protocol.h" 00044 #include "driver.h" 00045 00046 00047 #ifndef SL_PLATFORM_MULTI_THREADED 00048 00049 #include "nonos.h" 00050 00051 00052 _SlNonOsCB_t g__SlNonOsCB = {0}; 00053 00054 00055 _SlNonOsRetVal_t _SlNonOsSemSet(_SlNonOsSemObj_t* pSemObj , _SlNonOsSemObj_t Value) 00056 { 00057 *pSemObj = Value; 00058 return NONOS_RET_OK; 00059 } 00060 00061 _SlNonOsRetVal_t _SlNonOsSemGet(_SlNonOsSemObj_t* pSyncObj, _SlNonOsSemObj_t WaitValue, _SlNonOsSemObj_t SetValue, _SlNonOsTime_t Timeout) 00062 { 00063 #if (!defined (SL_TINY)) && (defined(sl_GetTimestamp)) 00064 _SlTimeoutParams_t TimeoutInfo={0}; 00065 #endif 00066 00067 /* If timeout 0 configured, just detect the value and return */ 00068 if ((Timeout ==0) && (WaitValue == *pSyncObj)) 00069 { 00070 *pSyncObj = SetValue; 00071 return NONOS_RET_OK; 00072 } 00073 00074 #if (!defined (SL_TINY)) && (defined(sl_GetTimestamp)) 00075 if ((Timeout != NONOS_WAIT_FOREVER) && (Timeout != NONOS_NO_WAIT)) 00076 { 00077 _SlDrvStartMeasureTimeout(&TimeoutInfo, Timeout); 00078 } 00079 #endif 00080 00081 #ifdef _SlSyncWaitLoopCallback 00082 _SlNonOsTime_t timeOutRequest = Timeout; 00083 #endif 00084 while (Timeout>0) 00085 { 00086 if (WaitValue == *pSyncObj) 00087 { 00088 *pSyncObj = SetValue; 00089 break; 00090 } 00091 #if (!defined (sl_GetTimestamp)) || (defined (SL_TINY_EXT)) 00092 if (Timeout != NONOS_WAIT_FOREVER) 00093 { 00094 Timeout--; 00095 } 00096 #else 00097 if ((Timeout != NONOS_WAIT_FOREVER) && (Timeout != NONOS_NO_WAIT)) 00098 { 00099 if (_SlDrvIsTimeoutExpired(&TimeoutInfo)) 00100 { 00101 return (_SlNonOsRetVal_t)NONOS_RET_ERR; 00102 } 00103 00104 } 00105 #endif 00106 00107 /* If we are in cmd context and waiting for its cmd response 00108 * do not handle spawn async events as the global lock was already taken */ 00109 if (FALSE == g_pCB->IsCmdRespWaited) 00110 { 00111 (void)_SlNonOsMainLoopTask(); 00112 } 00113 #ifdef _SlSyncWaitLoopCallback 00114 if( (__NON_OS_SYNC_OBJ_SIGNAL_VALUE == WaitValue) && (timeOutRequest != NONOS_NO_WAIT) ) 00115 { 00116 if (WaitValue == *pSyncObj) 00117 { 00118 *pSyncObj = SetValue; 00119 break; 00120 } 00121 _SlSyncWaitLoopCallback(); 00122 } 00123 #endif 00124 } 00125 00126 if (0 == Timeout) 00127 { 00128 return NONOS_RET_ERR; 00129 } 00130 else 00131 { 00132 return NONOS_RET_OK; 00133 } 00134 } 00135 00136 00137 _SlNonOsRetVal_t _SlNonOsSpawn(_SlSpawnEntryFunc_t pEntry , void* pValue , _u32 flags) 00138 { 00139 _i8 i = 0; 00140 00141 (void)flags; 00142 00143 #ifndef SL_TINY_EXT 00144 for (i=0 ; i<NONOS_MAX_SPAWN_ENTRIES ; i++) 00145 #endif 00146 { 00147 _SlNonOsSpawnEntry_t* pE = &g__SlNonOsCB.SpawnEntries[i]; 00148 00149 if (pE->IsAllocated == FALSE) 00150 { 00151 pE->pValue = pValue; 00152 pE->pEntry = pEntry; 00153 pE->IsAllocated = TRUE; 00154 #ifndef SL_TINY_EXT 00155 break; 00156 #endif 00157 } 00158 } 00159 00160 00161 return NONOS_RET_OK; 00162 } 00163 00164 00165 _SlNonOsRetVal_t _SlNonOsMainLoopTask(void) 00166 { 00167 _i8 i=0; 00168 void* pValue; 00169 00170 #ifndef SL_TINY_EXT 00171 for (i=0 ; i<NONOS_MAX_SPAWN_ENTRIES ; i++) 00172 #endif 00173 { 00174 _SlNonOsSpawnEntry_t* pE = &g__SlNonOsCB.SpawnEntries[i]; 00175 00176 00177 if (pE->IsAllocated == TRUE) 00178 { 00179 _SlSpawnEntryFunc_t pF = pE->pEntry; 00180 pValue = pE->pValue; 00181 00182 00183 /* Clear the entry */ 00184 pE->pEntry = NULL; 00185 pE->pValue = NULL; 00186 pE->IsAllocated = FALSE; 00187 00188 /* execute the spawn function */ 00189 pF(pValue); 00190 } 00191 } 00192 00193 return NONOS_RET_OK; 00194 } 00195 00196 00197 #endif /*(SL_PLATFORM != SL_PLATFORM_NON_OS)*/
Generated on Tue Jul 12 2022 12:06:48 by
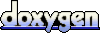