
DeepCover Embedded Security in IoT: Public-key Secured Data Paths
Dependencies: MaximInterface
meta.h
00001 // Tencent is pleased to support the open source community by making RapidJSON available. 00002 // 00003 // Copyright (C) 2015 THL A29 Limited, a Tencent company, and Milo Yip. All rights reserved. 00004 // 00005 // Licensed under the MIT License (the "License"); you may not use this file except 00006 // in compliance with the License. You may obtain a copy of the License at 00007 // 00008 // http://opensource.org/licenses/MIT 00009 // 00010 // Unless required by applicable law or agreed to in writing, software distributed 00011 // under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR 00012 // CONDITIONS OF ANY KIND, either express or implied. See the License for the 00013 // specific language governing permissions and limitations under the License. 00014 00015 #ifndef RAPIDJSON_INTERNAL_META_H_ 00016 #define RAPIDJSON_INTERNAL_META_H_ 00017 00018 #include "../rapidjson.h" 00019 00020 #ifdef __GNUC__ 00021 RAPIDJSON_DIAG_PUSH 00022 RAPIDJSON_DIAG_OFF(effc++) 00023 #endif 00024 #if defined(_MSC_VER) 00025 RAPIDJSON_DIAG_PUSH 00026 RAPIDJSON_DIAG_OFF(6334) 00027 #endif 00028 00029 #if RAPIDJSON_HAS_CXX11_TYPETRAITS 00030 #include <type_traits> 00031 #endif 00032 00033 //@cond RAPIDJSON_INTERNAL 00034 RAPIDJSON_NAMESPACE_BEGIN 00035 namespace internal { 00036 00037 // Helper to wrap/convert arbitrary types to void, useful for arbitrary type matching 00038 template <typename T> struct Void { typedef void Type; }; 00039 00040 /////////////////////////////////////////////////////////////////////////////// 00041 // BoolType, TrueType, FalseType 00042 // 00043 template <bool Cond> struct BoolType { 00044 static const bool Value = Cond; 00045 typedef BoolType Type; 00046 }; 00047 typedef BoolType<true> TrueType; 00048 typedef BoolType<false> FalseType; 00049 00050 00051 /////////////////////////////////////////////////////////////////////////////// 00052 // SelectIf, BoolExpr, NotExpr, AndExpr, OrExpr 00053 // 00054 00055 template <bool C> struct SelectIfImpl { template <typename T1, typename T2> struct Apply { typedef T1 Type; }; }; 00056 template <> struct SelectIfImpl<false> { template <typename T1, typename T2> struct Apply { typedef T2 Type; }; }; 00057 template <bool C, typename T1, typename T2> struct SelectIfCond : SelectIfImpl<C>::template Apply<T1,T2> {}; 00058 template <typename C, typename T1, typename T2> struct SelectIf : SelectIfCond<C::Value, T1, T2> {}; 00059 00060 template <bool Cond1, bool Cond2> struct AndExprCond : FalseType {}; 00061 template <> struct AndExprCond<true, true> : TrueType {}; 00062 template <bool Cond1, bool Cond2> struct OrExprCond : TrueType {}; 00063 template <> struct OrExprCond<false, false> : FalseType {}; 00064 00065 template <typename C> struct BoolExpr : SelectIf<C,TrueType,FalseType>::Type {}; 00066 template <typename C> struct NotExpr : SelectIf<C,FalseType,TrueType>::Type {}; 00067 template <typename C1, typename C2> struct AndExpr : AndExprCond<C1::Value, C2::Value>::Type {}; 00068 template <typename C1, typename C2> struct OrExpr : OrExprCond<C1::Value, C2::Value>::Type {}; 00069 00070 00071 /////////////////////////////////////////////////////////////////////////////// 00072 // AddConst, MaybeAddConst, RemoveConst 00073 template <typename T> struct AddConst { typedef const T Type; }; 00074 template <bool Constify, typename T> struct MaybeAddConst : SelectIfCond<Constify, const T, T> {}; 00075 template <typename T> struct RemoveConst { typedef T Type; }; 00076 template <typename T> struct RemoveConst<const T> { typedef T Type; }; 00077 00078 00079 /////////////////////////////////////////////////////////////////////////////// 00080 // IsSame, IsConst, IsMoreConst, IsPointer 00081 // 00082 template <typename T, typename U> struct IsSame : FalseType {}; 00083 template <typename T> struct IsSame<T, T> : TrueType {}; 00084 00085 template <typename T> struct IsConst : FalseType {}; 00086 template <typename T> struct IsConst<const T> : TrueType {}; 00087 00088 template <typename CT, typename T> 00089 struct IsMoreConst 00090 : AndExpr<IsSame<typename RemoveConst<CT>::Type, typename RemoveConst<T>::Type>, 00091 BoolType<IsConst<CT>::Value >= IsConst<T>::Value> >::Type {}; 00092 00093 template <typename T> struct IsPointer : FalseType {}; 00094 template <typename T> struct IsPointer<T*> : TrueType {}; 00095 00096 /////////////////////////////////////////////////////////////////////////////// 00097 // IsBaseOf 00098 // 00099 #if RAPIDJSON_HAS_CXX11_TYPETRAITS 00100 00101 template <typename B, typename D> struct IsBaseOf 00102 : BoolType< ::std::is_base_of<B,D>::value> {}; 00103 00104 #else // simplified version adopted from Boost 00105 00106 template<typename B, typename D> struct IsBaseOfImpl { 00107 RAPIDJSON_STATIC_ASSERT(sizeof(B) != 0); 00108 RAPIDJSON_STATIC_ASSERT(sizeof(D) != 0); 00109 00110 typedef char (&Yes)[1]; 00111 typedef char (&No) [2]; 00112 00113 template <typename T> 00114 static Yes Check(const D*, T); 00115 static No Check(const B*, int); 00116 00117 struct Host { 00118 operator const B*() const; 00119 operator const D*(); 00120 }; 00121 00122 enum { Value = (sizeof(Check(Host(), 0)) == sizeof(Yes)) }; 00123 }; 00124 00125 template <typename B, typename D> struct IsBaseOf 00126 : OrExpr<IsSame<B, D>, BoolExpr<IsBaseOfImpl<B, D> > >::Type {}; 00127 00128 #endif // RAPIDJSON_HAS_CXX11_TYPETRAITS 00129 00130 00131 ////////////////////////////////////////////////////////////////////////// 00132 // EnableIf / DisableIf 00133 // 00134 template <bool Condition, typename T = void> struct EnableIfCond { typedef T Type; }; 00135 template <typename T> struct EnableIfCond<false, T> { /* empty */ }; 00136 00137 template <bool Condition, typename T = void> struct DisableIfCond { typedef T Type; }; 00138 template <typename T> struct DisableIfCond<true, T> { /* empty */ }; 00139 00140 template <typename Condition, typename T = void> 00141 struct EnableIf : EnableIfCond<Condition::Value, T> {}; 00142 00143 template <typename Condition, typename T = void> 00144 struct DisableIf : DisableIfCond<Condition::Value, T> {}; 00145 00146 // SFINAE helpers 00147 struct SfinaeTag {}; 00148 template <typename T> struct RemoveSfinaeTag; 00149 template <typename T> struct RemoveSfinaeTag<SfinaeTag&(*)(T)> { typedef T Type; }; 00150 00151 #define RAPIDJSON_REMOVEFPTR_(type) \ 00152 typename ::RAPIDJSON_NAMESPACE::internal::RemoveSfinaeTag \ 00153 < ::RAPIDJSON_NAMESPACE::internal::SfinaeTag&(*) type>::Type 00154 00155 #define RAPIDJSON_ENABLEIF(cond) \ 00156 typename ::RAPIDJSON_NAMESPACE::internal::EnableIf \ 00157 <RAPIDJSON_REMOVEFPTR_(cond)>::Type * = NULL 00158 00159 #define RAPIDJSON_DISABLEIF(cond) \ 00160 typename ::RAPIDJSON_NAMESPACE::internal::DisableIf \ 00161 <RAPIDJSON_REMOVEFPTR_(cond)>::Type * = NULL 00162 00163 #define RAPIDJSON_ENABLEIF_RETURN(cond,returntype) \ 00164 typename ::RAPIDJSON_NAMESPACE::internal::EnableIf \ 00165 <RAPIDJSON_REMOVEFPTR_(cond), \ 00166 RAPIDJSON_REMOVEFPTR_(returntype)>::Type 00167 00168 #define RAPIDJSON_DISABLEIF_RETURN(cond,returntype) \ 00169 typename ::RAPIDJSON_NAMESPACE::internal::DisableIf \ 00170 <RAPIDJSON_REMOVEFPTR_(cond), \ 00171 RAPIDJSON_REMOVEFPTR_(returntype)>::Type 00172 00173 } // namespace internal 00174 RAPIDJSON_NAMESPACE_END 00175 //@endcond 00176 00177 #if defined(__GNUC__) || defined(_MSC_VER) 00178 RAPIDJSON_DIAG_POP 00179 #endif 00180 00181 #endif // RAPIDJSON_INTERNAL_META_H_
Generated on Tue Jul 12 2022 12:06:48 by
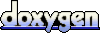