Lancaster University's fork of the mbed BLE API. Lives on github, https://github.com/lancaster-university/BLE_API
Dependents: microbit-dal microbit-dal microbit-ble-open microbit-dal ... more
Fork of BLE_API by
CallChainOfFunctionPointersWithContext< ContextType > Class Template Reference
Group one or more functions in an instance of a CallChainOfFunctionPointersWithContext, then call them in sequence using CallChainOfFunctionPointersWithContext::call(). More...
#include <CallChainOfFunctionPointersWithContext.h>
Inherits SafeBool< CallChainOfFunctionPointersWithContext< ContextType > >.
Public Member Functions | |
CallChainOfFunctionPointersWithContext () | |
Create an empty chain. | |
pFunctionPointerWithContext_t | add (void(*function)(ContextType context)) |
Add a function at the front of the chain. | |
template<typename T > | |
pFunctionPointerWithContext_t | add (T *tptr, void(T::*mptr)(ContextType context)) |
Add a function at the front of the chain. | |
pFunctionPointerWithContext_t | add (const FunctionPointerWithContext< ContextType > &func) |
Add a function at the front of the chain. | |
bool | detach (const FunctionPointerWithContext< ContextType > &toDetach) |
Detach a function pointer from a callchain. | |
void | clear (void) |
Clear the call chain (remove all functions in the chain). | |
void | call (ContextType context) |
Call all the functions in the chain in sequence. | |
void | call (ContextType context) const |
same as above but const | |
void | operator() (ContextType context) const |
same as above but with function call operator | |
bool | toBool () const |
bool conversion operation | |
operator BoolType_t () const | |
bool operator implementation, derived class has to provide bool toBool() const function. |
Detailed Description
template<typename ContextType>
class CallChainOfFunctionPointersWithContext< ContextType >
Group one or more functions in an instance of a CallChainOfFunctionPointersWithContext, then call them in sequence using CallChainOfFunctionPointersWithContext::call().
Used mostly by the interrupt chaining code, but can be used for other purposes.
Example:
CallChainOfFunctionPointersWithContext<void *> chain; void first(void *context) { printf("'first' function.\n"); } void second(void *context) { printf("'second' function.\n"); } class Test { public: void f(void *context) { printf("A::f (class member).\n"); } }; int main() { Test test; chain.add(second); chain.add_front(first); chain.add(&test, &Test::f); chain.call(); }
Definition at line 60 of file CallChainOfFunctionPointersWithContext.h.
Constructor & Destructor Documentation
Create an empty chain.
- Parameters:
-
size (optional) Initial size of the chain.
Definition at line 69 of file CallChainOfFunctionPointersWithContext.h.
Member Function Documentation
pFunctionPointerWithContext_t add | ( | void(*)(ContextType context) | function ) |
Add a function at the front of the chain.
- Parameters:
-
function A pointer to a void function.
- Returns:
- The function object created for 'function'.
Definition at line 84 of file CallChainOfFunctionPointersWithContext.h.
pFunctionPointerWithContext_t add | ( | T * | tptr, |
void(T::*)(ContextType context) | mptr | ||
) |
Add a function at the front of the chain.
- Parameters:
-
tptr Pointer to the object to call the member function on. mptr Pointer to the member function to be called.
- Returns:
- The function object created for 'tptr' and 'mptr'.
Definition at line 97 of file CallChainOfFunctionPointersWithContext.h.
pFunctionPointerWithContext_t add | ( | const FunctionPointerWithContext< ContextType > & | func ) |
Add a function at the front of the chain.
- Parameters:
-
func The FunctionPointerWithContext to add.
Definition at line 105 of file CallChainOfFunctionPointersWithContext.h.
void call | ( | ContextType | context ) | const |
same as above but const
Definition at line 170 of file CallChainOfFunctionPointersWithContext.h.
void call | ( | ContextType | context ) |
Call all the functions in the chain in sequence.
Definition at line 163 of file CallChainOfFunctionPointersWithContext.h.
void clear | ( | void | ) |
Clear the call chain (remove all functions in the chain).
Definition at line 146 of file CallChainOfFunctionPointersWithContext.h.
bool detach | ( | const FunctionPointerWithContext< ContextType > & | toDetach ) |
Detach a function pointer from a callchain.
toDetach FunctionPointerWithContext to detach from this callchain
- Returns:
- true if a function pointer has been detached and false otherwise
Definition at line 116 of file CallChainOfFunctionPointersWithContext.h.
operator BoolType_t | ( | ) | const [inherited] |
bool operator implementation, derived class has to provide bool toBool() const function.
Definition at line 94 of file SafeBool.h.
void operator() | ( | ContextType | context ) | const |
same as above but with function call operator
void first(bool); void second(bool); CallChainOfFunctionPointerWithContext<bool> foo; foo.attach(first); foo.attach(second); // call the callchain like a function foo(true);
Definition at line 201 of file CallChainOfFunctionPointersWithContext.h.
bool toBool | ( | ) | const |
bool conversion operation
Definition at line 208 of file CallChainOfFunctionPointersWithContext.h.
Generated on Tue Jul 12 2022 17:17:58 by
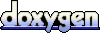