
Check refresh speed by transfermode 4bit,3bit,1bit with MIP8F_SPI_Ver50
Dependencies: MIP8F_SPI_Ver50 MIP8f_FRDM_LineBuffer_sample mbed
Dependents: MIP8f_FRDM_Animation_sample
Adafruit_GFX.h
00001 /*********************************** 00002 This is a our graphics core library, for all our displays. 00003 We'll be adapting all the 00004 existing libaries to use this core to make updating, support 00005 and upgrading easier! 00006 00007 Adafruit invests time and resources providing this open source code, 00008 please support Adafruit and open-source hardware by purchasing 00009 products from Adafruit! 00010 00011 Written by Limor Fried/Ladyada for Adafruit Industries. 00012 BSD license, check license.txt for more information 00013 All text above must be included in any redistribution 00014 ****************************************/ 00015 00016 /* 00017 * Modified by Neal Horman 7/14/2012 for use in LPC1768 00018 */ 00019 00020 #ifndef _ADAFRUIT_GFX_H_ 00021 #define _ADAFRUIT_GFX_H_ 00022 00023 static inline void swap(int16_t &a, int16_t &b) 00024 { 00025 int16_t t = a; 00026 00027 a = b; 00028 b = t; 00029 } 00030 00031 #ifndef _BV 00032 #define _BV(bit) (1<<(bit)) 00033 #endif 00034 00035 #define BLACK 0 00036 #define WHITE 1 00037 00038 class Adafruit_GFX : public Stream 00039 { 00040 public: 00041 Adafruit_GFX(int16_t w, int16_t h) 00042 : _rawWidth(w) 00043 , _rawHeight(h) 00044 , _width(w) 00045 , _height(h) 00046 , cursor_x(0) 00047 , cursor_y(0) 00048 , textcolor(WHITE) 00049 , textbgcolor(BLACK) 00050 , textsize(1) 00051 , rotation(0) 00052 , wrap(true) 00053 {}; 00054 00055 // this must be defined by the subclass 00056 virtual void drawPixel(int16_t x, int16_t y, uint16_t color) = 0; 00057 // this is optional 00058 virtual void invertDisplay(bool i) {}; 00059 00060 // Stream implementation - provides printf() interface 00061 // You would otherwise be forced to use writeChar() 00062 virtual int _putc(int value) { return writeChar(value); }; 00063 virtual int _getc() { return -1; }; 00064 00065 #ifdef WANT_ABSTRACTS 00066 // these are 'generic' drawing functions, so we can share them! 00067 virtual void drawLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t color); 00068 virtual void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color); 00069 virtual void drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color); 00070 virtual void drawRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color); 00071 virtual void fillRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color); 00072 virtual void fillScreen(uint16_t color); 00073 00074 void drawCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color); 00075 void drawCircleHelper(int16_t x0, int16_t y0, int16_t r, uint8_t cornername, uint16_t color); 00076 void fillCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color); 00077 void fillCircleHelper(int16_t x0, int16_t y0, int16_t r, uint8_t cornername, int16_t delta, uint16_t color); 00078 00079 void drawTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color); 00080 void fillTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color); 00081 void drawRoundRect(int16_t x0, int16_t y0, int16_t w, int16_t h, int16_t radius, uint16_t color); 00082 void fillRoundRect(int16_t x0, int16_t y0, int16_t w, int16_t h, int16_t radius, uint16_t color); 00083 #endif 00084 00085 void drawBitmap(int16_t x, int16_t y, const uint8_t *bitmap, int16_t w, int16_t h, uint16_t color); 00086 void drawChar(int16_t x, int16_t y, unsigned char c, uint16_t color, uint16_t bg, uint8_t size); 00087 size_t writeChar(uint8_t); 00088 00089 int16_t width(void) { return _width; }; 00090 int16_t height(void) { return _height; }; 00091 00092 void setCursor(int16_t x, int16_t y) { cursor_x = x; cursor_y = y; }; 00093 void setTextSize(uint8_t s) { textsize = (s > 0) ? s : 1; }; 00094 void setTextColor(uint16_t c) { textcolor = c; textbgcolor = c; } 00095 void setTextColor(uint16_t c, uint16_t b) { textcolor = c; textbgcolor = b; }; 00096 void setTextWrap(bool w) { wrap = w; }; 00097 00098 void setRotation(uint8_t r); 00099 uint8_t getRotation(void) { rotation %= 4; return rotation; }; 00100 00101 protected: 00102 int16_t _rawWidth, _rawHeight; // this is the 'raw' display w/h - never changes 00103 int16_t _width, _height; // dependent on rotation 00104 int16_t cursor_x, cursor_y; 00105 uint16_t textcolor, textbgcolor; 00106 uint8_t textsize; 00107 uint8_t rotation; 00108 bool wrap; // If set, 'wrap' text at right edge of display 00109 }; 00110 00111 #endif
Generated on Tue Jul 12 2022 21:16:32 by
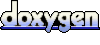