
Code for the nRF51822. The BLE advertises, connects to a master, sends information about the status of the LDRs and receives inputs from the Master
Dependencies: BLE_API mbed nRF51822
Fork of BLE_UART1 by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "BLEDevice.h" 00019 #include <string.h> 00020 00021 #include "UARTService.h" 00022 00023 #define NEED_CONSOLE_OUTPUT 1 /* Set this if you need debug messages on the console; 00024 * it will have an impact on code-size and power consumption. */ 00025 00026 #if NEED_CONSOLE_OUTPUT 00027 #define DEBUG(...) { printf(__VA_ARGS__); } 00028 #else 00029 #define DEBUG(...) /* nothing */ 00030 #endif /* #if NEED_CONSOLE_OUTPUT */ 00031 00032 #define ON 1960 00033 #define OFF 40 00034 #define PULSE_WIDTH 2000 00035 #define OUTPUT_LENGTH 35 00036 00037 BLEDevice ble; 00038 DigitalOut led1(LED1); 00039 DigitalOut led2(LED2); 00040 DigitalOut pwmOut(P0_30); 00041 00042 AnalogIn ldr0(P0_1); 00043 AnalogIn ldr1(P0_2); 00044 AnalogIn ldr2(P0_3); 00045 AnalogIn ldr3(P0_4); 00046 00047 int threshold = 0; 00048 00049 //Output string to indicate the status of the LDRs 00050 char output[50] = "LDR0 = w x y z"; 00051 00052 UARTService *uartServicePtr; 00053 #define TIMER 0 00054 //Creating timer Instance 00055 00056 Ticker ticker; 00057 #if TIMER 00058 long int timerCount = 0; 00059 #endif 00060 00061 void disconnectionCallback(Gap::Handle_t handle, Gap::DisconnectionReason_t reason) 00062 { 00063 DEBUG("Disconnected!\n\r"); 00064 DEBUG("Restarting the advertising process\n\r"); 00065 ble.startAdvertising(); 00066 } 00067 00068 //Thresholding the values of the LDRs 00069 void getValuesofLDR() 00070 { 00071 if (ldr0.read() < 0.52f) 00072 output[7] = '0'; 00073 else 00074 output[7] = '1'; 00075 if (ldr0.read() < 0.52f) 00076 output[9] = '0'; 00077 else 00078 output[9] = '1'; 00079 if (ldr0.read() < 0.52f) 00080 output[11] = '0'; 00081 else 00082 output[11] = '1'; 00083 if (ldr0.read() < 0.52f) 00084 output[13] = '0'; 00085 else 00086 output[13] = '1'; 00087 00088 } 00089 00090 //Preparation of output string 00091 void onDataWritten(const GattCharacteristicWriteCBParams *params) 00092 { 00093 char *str = 0; 00094 int count = 0; 00095 00096 if ((uartServicePtr != NULL) && (params->charHandle == uartServicePtr->getTXCharacteristicHandle())) { 00097 uint16_t bytesRead = params->len; 00098 DEBUG("received %u bytes\n\r", bytesRead); 00099 00100 //Obtaining user command 00101 str = (char *)malloc((sizeof(char) * bytesRead) + 1); 00102 while(count <= bytesRead) 00103 { 00104 *(str + count) = *(params->data + count); 00105 count++; 00106 } 00107 count--; 00108 *(str + count) = '\0'; 00109 DEBUG("payload = %s\n\r",str); 00110 00111 //Comparing user input against keywords 00112 if (strncmp(str,"On",bytesRead) == 0) 00113 { 00114 led2 = 0; 00115 pwmOut = 0; 00116 bytesRead = 7; 00117 strncpy((char *)params->data,"Lamp On", bytesRead); 00118 } 00119 else if (strncmp(str,"Off",bytesRead) == 0) 00120 { 00121 led2 = 1; 00122 pwmOut = 1; 00123 bytesRead = 8; 00124 strncpy((char *)params->data,"Lamp Off", bytesRead); 00125 } 00126 else if (strncmp(str,"Read",bytesRead) == 0) 00127 { 00128 bytesRead = 13; 00129 getValuesofLDR(); 00130 strncpy((char *)params->data,output,bytesRead); 00131 00132 } 00133 else//None of the commands match. Invalid entry 00134 { 00135 bytesRead = 14; 00136 strncpy((char *)params->data,"Invalid Entry", bytesRead); 00137 } 00138 free(str); 00139 ble.updateCharacteristicValue(uartServicePtr->getRXCharacteristicHandle(), params->data, bytesRead); 00140 } 00141 } 00142 00143 void periodicCallback(void) 00144 { 00145 #if TIMER 00146 timerCount++; 00147 if ((timerCount < threshold)) 00148 { 00149 led1 = 1; 00150 pwm_out = 1; 00151 } 00152 else 00153 { 00154 led1 = 0; 00155 pwm_out = 0; 00156 } 00157 if (timerCount == PULSE_WIDTH) 00158 timerCount = 0; 00159 #else 00160 led1 = !led1; 00161 #endif 00162 00163 } 00164 00165 int main(void) 00166 { 00167 led1 = 1; 00168 DEBUG("Initialising the nRF51822\n\r"); 00169 //ticker.attach(periodicCallback, 0.00001f); 00170 ble.init(); 00171 00172 ble.onDisconnection(disconnectionCallback); 00173 00174 ble.onDataWritten(onDataWritten); 00175 00176 /* setup advertising */ 00177 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00178 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00179 ble.accumulateAdvertisingPayload(GapAdvertisingData::SHORTENED_LOCAL_NAME, 00180 (const uint8_t *)"AdarshBLE UART", sizeof("AdarshBLE UART") - 1); 00181 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS, 00182 (const uint8_t *)UARTServiceUUID_reversed, sizeof(UARTServiceUUID_reversed)); 00183 00184 ble.setAdvertisingInterval(160); /* 100ms; in multiples of 0.625ms. */ 00185 ble.startAdvertising(); 00186 00187 UARTService uartService(ble); 00188 uartServicePtr = &uartService; 00189 00190 while (true) { 00191 ble.waitForEvent(); 00192 } 00193 }
Generated on Sun Jul 17 2022 07:23:55 by
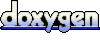