
teste 1 motor para Bruno
Dependencies: X_NUCLEO_IHM01A1 mbed
Fork of HelloWorld_IHM01A1 by
main.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file main.cpp 00004 * @author Davide Aliprandi, STMicroelectronics 00005 * @version V1.0.0 00006 * @date October 14th, 2015 00007 * @brief mbed test application for the STMicroelectronics X-NUCLEO-IHM01A1 00008 * Motor Control Expansion Board: control of 1 motor. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 00040 /* Includes ------------------------------------------------------------------*/ 00041 00042 /* mbed specific header files. */ 00043 #include "mbed.h" 00044 00045 /* Helper header files. */ 00046 #include "DevSPI.h" 00047 00048 /* Component specific header files. */ 00049 #include "L6474.h" 00050 00051 00052 /* Definitions ---------------------------------------------------------------*/ 00053 00054 /* Number of steps. */ 00055 #define STEPS_1 (400 * 8) /* 1 revolution given a 400 steps motor configured at 1/8 microstep mode. */ 00056 00057 /* Delay in milliseconds. */ 00058 #define DELAY_1 1000 00059 #define DELAY_2 2000 00060 #define DELAY_3 6000 00061 #define DELAY_4 8000 00062 00063 /* Speed in pps (Pulses Per Second). 00064 In Full Step mode: 1 pps = 1 step/s). 00065 In 1/N Step Mode: N pps = 1 step/s). */ 00066 #define SPEED_1 2400 00067 #define SPEED_2 1200 00068 00069 00070 /* Variables -----------------------------------------------------------------*/ 00071 00072 /* Initialization parameters. */ 00073 L6474_init_t init = { 00074 160, /* Acceleration rate in pps^2. Range: (0..+inf). */ 00075 160, /* Deceleration rate in pps^2. Range: (0..+inf). */ 00076 1600, /* Maximum speed in pps. Range: (30..10000]. */ 00077 800, /* Minimum speed in pps. Range: [30..10000). */ 00078 250, /* Torque regulation current in mA. Range: 31.25mA to 4000mA. */ 00079 L6474_OCD_TH_750mA, /* Overcurrent threshold (OCD_TH register). */ 00080 L6474_CONFIG_OC_SD_ENABLE, /* Overcurrent shutwdown (OC_SD field of CONFIG register). */ 00081 L6474_CONFIG_EN_TQREG_TVAL_USED, /* Torque regulation method (EN_TQREG field of CONFIG register). */ 00082 L6474_STEP_SEL_1_8, /* Step selection (STEP_SEL field of STEP_MODE register). */ 00083 L6474_SYNC_SEL_1_2, /* Sync selection (SYNC_SEL field of STEP_MODE register). */ 00084 L6474_FAST_STEP_12us, /* Fall time value (T_FAST field of T_FAST register). Range: 2us to 32us. */ 00085 L6474_TOFF_FAST_8us, /* Maximum fast decay time (T_OFF field of T_FAST register). Range: 2us to 32us. */ 00086 3, /* Minimum ON time in us (TON_MIN register). Range: 0.5us to 64us. */ 00087 21, /* Minimum OFF time in us (TOFF_MIN register). Range: 0.5us to 64us. */ 00088 L6474_CONFIG_TOFF_044us, /* Target Swicthing Period (field TOFF of CONFIG register). */ 00089 L6474_CONFIG_SR_320V_us, /* Slew rate (POW_SR field of CONFIG register). */ 00090 L6474_CONFIG_INT_16MHZ, /* Clock setting (OSC_CLK_SEL field of CONFIG register). */ 00091 L6474_ALARM_EN_OVERCURRENT | 00092 L6474_ALARM_EN_THERMAL_SHUTDOWN | 00093 L6474_ALARM_EN_THERMAL_WARNING | 00094 L6474_ALARM_EN_UNDERVOLTAGE | 00095 L6474_ALARM_EN_SW_TURN_ON | 00096 L6474_ALARM_EN_WRONG_NPERF_CMD /* Alarm (ALARM_EN register). */ 00097 }; 00098 00099 /* Motor Control Component. */ 00100 L6474 *motor; 00101 00102 00103 /* Functions -----------------------------------------------------------------*/ 00104 00105 /** 00106 * @brief This is an example of user handler for the flag interrupt. 00107 * @param None 00108 * @retval None 00109 * @note If needed, implement it, and then attach and enable it: 00110 * + motor->attach_flag_irq(&flag_irq_handler); 00111 * + motor->enable_flag_irq(); 00112 * To disable it: 00113 * + motor->disble_flag_irq(); 00114 */ 00115 void flag_irq_handler(void) 00116 { 00117 /* Set ISR flag. */ 00118 motor->isr_flag = TRUE; 00119 00120 /* Get the value of the status register. */ 00121 unsigned int status = motor->get_status(); 00122 00123 /* Check NOTPERF_CMD flag: if set, the command received by SPI can't be performed. */ 00124 /* This often occures when a command is sent to the L6474 while it is not in HiZ state. */ 00125 if ((status & L6474_STATUS_NOTPERF_CMD) == L6474_STATUS_NOTPERF_CMD) { 00126 printf(" WARNING: \"FLAG\" interrupt triggered. Non-performable command detected when updating L6474's registers while not in HiZ state.\r\n"); 00127 } 00128 00129 /* Reset ISR flag. */ 00130 motor->isr_flag = FALSE; 00131 } 00132 00133 00134 /* Main ----------------------------------------------------------------------*/ 00135 00136 int main() 00137 { 00138 /*----- Initialization. -----*/ 00139 00140 /* Initializing SPI bus. */ 00141 DevSPI dev_spi(D11, D12, D13); 00142 00143 /* Initializing Motor Control Component. */ 00144 motor = new L6474(D2, D8, D7, D9, D10, dev_spi); 00145 if (motor->init(&init) != COMPONENT_OK) { 00146 exit(EXIT_FAILURE); 00147 } 00148 00149 /* Attaching and enabling interrupt handlers. */ 00150 motor->attach_flag_irq(&flag_irq_handler); 00151 motor->enable_flag_irq(); 00152 00153 /* Printing to the console. */ 00154 printf("Motor Control Application Example for 1 Motor\r\n\n"); 00155 00156 00157 /*----- Moving. -----*/ 00158 00159 /* Printing to the console. */ 00160 printf("--> Moving forward %d steps.\r\n", STEPS_1); 00161 00162 /* Moving N steps in the forward direction. */ 00163 motor->move(StepperMotor::FWD, STEPS_1); 00164 00165 /* Waiting while the motor is active. */ 00166 motor->wait_while_active(); 00167 00168 /* Getting current position. */ 00169 int position = motor->get_position(); 00170 00171 /* Printing to the console. */ 00172 printf(" Position: %d.\r\n", position); 00173 00174 /* Waiting. */ 00175 wait_ms(DELAY_1); 00176 00177 00178 /*----- Changing the motor setting. -----*/ 00179 00180 /* Printing to the console. */ 00181 printf("--> Setting Torque Regulation Current to 500[mA].\r\n"); 00182 00183 /* Increasing the torque regulation current to 500[mA]. */ 00184 motor->set_parameter(L6474_TVAL, 500); 00185 00186 /* Printing to the console. */ 00187 printf("--> Doubling the microsteps.\r\n"); 00188 00189 /* Doubling the microsteps. */ 00190 if (!motor->set_step_mode((StepperMotor::step_mode_t) STEP_MODE_1_16)) { 00191 printf(" Step Mode not allowed.\r\n"); 00192 } 00193 00194 /* Waiting. */ 00195 wait_ms(DELAY_1); 00196 00197 /* Printing to the console. */ 00198 printf("--> Setting Home.\r\n"); 00199 00200 /* Setting the current position to be the home position. */ 00201 motor->set_home(); 00202 00203 /* Getting current position. */ 00204 position = motor->get_position(); 00205 00206 /* Printing to the console. */ 00207 printf(" Position: %d.\r\n", position); 00208 00209 /* Waiting. */ 00210 wait_ms(DELAY_2); 00211 00212 00213 /*----- Moving. -----*/ 00214 00215 /* Printing to the console. */ 00216 printf("--> Moving backward %d steps.\r\n", STEPS_1); 00217 00218 /* Moving N steps in the backward direction. */ 00219 motor->move(StepperMotor::BWD, STEPS_1); 00220 00221 /* Waiting while the motor is active. */ 00222 motor->wait_while_active(); 00223 00224 /* Getting current position. */ 00225 position = motor->get_position(); 00226 00227 /* Printing to the console. */ 00228 printf(" Position: %d.\r\n", position); 00229 00230 /* Waiting. */ 00231 wait_ms(DELAY_1); 00232 00233 00234 /*----- Going to a specified position. -----*/ 00235 00236 /* Printing to the console. */ 00237 printf("--> Going to position %d.\r\n", STEPS_1); 00238 00239 /* Requesting to go to a specified position. */ 00240 motor->go_to(STEPS_1); 00241 00242 /* Waiting while the motor is active. */ 00243 motor->wait_while_active(); 00244 00245 /* Getting current position. */ 00246 position = motor->get_position(); 00247 00248 /* Printing to the console. */ 00249 printf(" Position: %d.\r\n", position); 00250 00251 /* Waiting. */ 00252 wait_ms(DELAY_2); 00253 00254 00255 /*----- Going Home. -----*/ 00256 00257 /* Printing to the console. */ 00258 printf("--> Going Home.\r\n"); 00259 00260 /* Requesting to go to home. */ 00261 motor->go_home(); 00262 00263 /* Waiting while the motor is active. */ 00264 motor->wait_while_active(); 00265 00266 /* Getting current position. */ 00267 position = motor->get_position(); 00268 00269 /* Printing to the console. */ 00270 printf(" Position: %d.\r\n", position); 00271 00272 /* Waiting. */ 00273 wait_ms(DELAY_2); 00274 00275 00276 /*----- Running. -----*/ 00277 00278 /* Printing to the console. */ 00279 printf("--> Running backward for %d seconds.\r\n", DELAY_3 / 1000); 00280 00281 /* Requesting to run backward. */ 00282 motor->run(StepperMotor::BWD); 00283 00284 /* Waiting. */ 00285 wait_ms(DELAY_3); 00286 00287 /* Getting current speed. */ 00288 int speed = motor->get_speed(); 00289 00290 /* Printing to the console. */ 00291 printf(" Speed: %d.\r\n", speed); 00292 00293 /*----- Increasing the speed while running. -----*/ 00294 00295 /* Printing to the console. */ 00296 printf("--> Increasing the speed while running again for %d seconds.\r\n", DELAY_3 / 1000); 00297 00298 /* Increasing the speed. */ 00299 motor->set_max_speed(SPEED_1); 00300 00301 /* Waiting. */ 00302 wait_ms(DELAY_3); 00303 00304 /* Getting current speed. */ 00305 speed = motor->get_speed(); 00306 00307 /* Printing to the console. */ 00308 printf(" Speed: %d.\r\n", speed); 00309 00310 00311 /*----- Decreasing the speed while running. -----*/ 00312 00313 /* Printing to the console. */ 00314 printf("--> Decreasing the speed while running again for %d seconds.\r\n", DELAY_4 / 1000); 00315 00316 /* Decreasing the speed. */ 00317 motor->set_max_speed(SPEED_2); 00318 00319 /* Waiting. */ 00320 wait_ms(DELAY_4); 00321 00322 /* Getting current speed. */ 00323 speed = motor->get_speed(); 00324 00325 /* Printing to the console. */ 00326 printf(" Speed: %d.\r\n", speed); 00327 00328 00329 /*----- Hard Stop. -----*/ 00330 00331 /* Printing to the console. */ 00332 printf("--> Hard Stop.\r\n"); 00333 00334 /* Requesting to immediatly stop. */ 00335 motor->hard_stop(); 00336 00337 /* Waiting while the motor is active. */ 00338 motor->wait_while_active(); 00339 00340 /* Waiting. */ 00341 wait_ms(DELAY_2); 00342 00343 00344 /*----- Infinite Loop. -----*/ 00345 00346 /* Printing to the console. */ 00347 printf("--> Infinite Loop...\r\n"); 00348 00349 /* Setting the current position to be the home position. */ 00350 motor->set_home(); 00351 00352 /* Infinite Loop. */ 00353 while (true) { 00354 /* Requesting to go to a specified position. */ 00355 motor->go_to(STEPS_1 >> 1); 00356 00357 /* Waiting while the motor is active. */ 00358 motor->wait_while_active(); 00359 00360 /* Requesting to go to a specified position. */ 00361 motor->go_to(- (STEPS_1 >> 1)); 00362 00363 /* Waiting while the motor is active. */ 00364 motor->wait_while_active(); 00365 } 00366 }
Generated on Sat Jul 16 2022 02:41:08 by
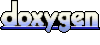