
version 1.0
Dependencies: CMSIS_DSP_401 GPS MPU9150_DMP PID QuaternionMath Servo mbed
Fork of SolarOnFoils_MainModule_20150518 by
systemVar.h
00001 #ifndef SYSTEMVAR_H_INCLUDED 00002 #define SYSTEMVAR_H_INCLUDED 00003 00004 #include "mbed.h" 00005 #include "string.h" 00006 #include "Quaternion.h" 00007 00008 //Solar on Foils display values 00009 enum VALUE_t 00010 { 00011 ////////////////////////////////////////////////////////////////////////////////////// 00012 // system measured values // 00013 ////////////////////////////////////////////////////////////////////////////////////// 00014 _ROLL = 0, // roll 00015 _PITCH, // pitch 00016 _PHEIGHT, // portside height 00017 _SHEIGHT, // starboard height 00018 _PCURRENT, // portside current 00019 _SCURRENT, // starboard current 00020 _SPEED, // speed 00021 ////////////////////////////////////////////////////////////////////////////////////// 00022 // system variables // 00023 ////////////////////////////////////////////////////////////////////////////////////// 00024 _MAXROLL, // variable max roll 00025 _MINPITCH, // variable min pitch 00026 _MAXPITCH, // variable max pitch 00027 _TAKEOFFSPEED, // variable take off speed 00028 _HEIGHTFOILBORNE, // variable flight height foilborne 00029 _HEIGHTFOILASSIST, // variable flight height foil assist 00030 _AOAFOILASSIST // variable AOA foil assist 00031 }; 00032 00033 class SystemVar 00034 { 00035 private: 00036 // variable 00037 00038 char cValue[4]; 00039 char cMessage; 00040 00041 VALUE_t value; 00042 00043 uint32_t uiCounter; 00044 uint32_t uiRoll[2]; 00045 uint32_t uiPitch[2]; 00046 uint32_t uiPHeight; 00047 uint32_t uiSHeight; 00048 uint32_t uiPCurrent; 00049 uint32_t uiSCurrent; 00050 uint32_t uiSpeed; 00051 uint32_t uiVarMaxRoll; 00052 uint32_t uiVarMinPitch; 00053 uint32_t uiVarMaxPitch; 00054 uint32_t uiVarTakeOffSpeed; 00055 uint32_t uiVarHeightFoilBorne; 00056 uint32_t uiVarHeightFoilAssist; 00057 uint32_t uiVarAOAFoilAssist; 00058 00059 bool bError; 00060 00061 public: 00062 // Constructor 00063 SystemVar(); 00064 // Destructor 00065 ~SystemVar(); 00066 00067 // Gets 00068 char* getValue(void); 00069 int iGetRollPolarity(void); 00070 int iGetPitchPolarity(void); 00071 uint32_t uiGetRoll(void); 00072 uint32_t uiGetPitch(void); 00073 int32_t iGetHeightFoilBorne(void); 00074 uint32_t uiGetVarMaxRoll(void); 00075 uint32_t uiGetVarMinPitch(void); 00076 uint32_t uiGetVarMaxPitch(void); 00077 uint32_t uiGetVarTakeOffSpeed(void); 00078 uint32_t uiGetVarHeightFoilBorne(void); 00079 uint32_t uiGetVarHeightFoilAssist(void); 00080 uint32_t uiGetVarAOAFoilAssist(void); 00081 00082 00083 // Sets 00084 void vSetRoll(Quaternion); 00085 void vSetPitch(Quaternion); 00086 void vSetPHeight(uint32_t); 00087 void vSetSHeight(uint32_t); 00088 void vSetPCurrent(uint32_t); 00089 void vSetSCurrent(uint32_t); 00090 void vSetSpeed(uint32_t); 00091 // Set variables 00092 void vVarMaxRoll(uint32_t); 00093 void vVarMinPitch(uint32_t); 00094 void vVarMaxPitch(uint32_t); 00095 void vVarTakeOffSpeed(uint32_t); 00096 void vVarHeightFoilBorne(uint32_t); 00097 void vVarHeightFoilAssist(uint32_t); 00098 void vVarAOAFoilAssist(uint32_t); 00099 00100 // Other functions 00101 void init(void); 00102 void itoa( uint32_t , char *); 00103 void cShowValue(VALUE_t); 00104 void vIncrease(VALUE_t); 00105 void vDecrease(VALUE_t); 00106 00107 }; 00108 #endif
Generated on Tue Jul 12 2022 21:09:14 by
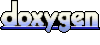