
This a fork of https://developer.mbed.org/teams/mbed-os-examples/code/mbed-os-example-blinky/
Embed:
(wiki syntax)
Show/hide line numbers
stats_report.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2018 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 */ 00005 00006 #ifndef STATS_REPORT_H 00007 #define STATS_REPORT 00008 00009 #include "mbed.h" 00010 00011 /** 00012 * System Reporting library. Provides runtime information on device: 00013 * - CPU sleep, idle, and wake times 00014 * - Heap and stack usage 00015 * - Thread information 00016 * - Static system information 00017 */ 00018 class SystemReport { 00019 mbed_stats_heap_t heap_stats; 00020 mbed_stats_cpu_t cpu_stats; 00021 mbed_stats_sys_t sys_stats; 00022 00023 mbed_stats_thread_t *thread_stats; 00024 uint8_t thread_count; 00025 uint8_t max_thread_count; 00026 uint32_t sample_time_ms; 00027 00028 public: 00029 /** 00030 * SystemReport - Sample rate in ms is required to handle the CPU percent awake logic 00031 */ 00032 SystemReport(uint32_t sample_rate) : max_thread_count(8), sample_time_ms(sample_rate) 00033 { 00034 thread_stats = new mbed_stats_thread_t[max_thread_count]; 00035 00036 // Collect the static system information 00037 mbed_stats_sys_get(&sys_stats); 00038 00039 printf("=============================== SYSTEM INFO ================================\r\n"); 00040 printf("Mbed OS Version: %ld \r\n", sys_stats.os_version); 00041 printf("CPU ID: 0x%lx \r\n", sys_stats.cpu_id); 00042 printf("Compiler ID: %d \r\n", sys_stats.compiler_id); 00043 printf("Compiler Version: %ld \r\n", sys_stats.compiler_version); 00044 00045 for (int i = 0; i < MBED_MAX_MEM_REGIONS; i++) { 00046 if (sys_stats.ram_size[i] != 0) { 00047 printf("RAM%d: Start 0x%lx Size: 0x%lx \r\n", i, sys_stats.ram_start[i], sys_stats.ram_size[i]); 00048 } 00049 } 00050 for (int i = 0; i < MBED_MAX_MEM_REGIONS; i++) { 00051 if (sys_stats.rom_size[i] != 0) { 00052 printf("ROM%d: Start 0x%lx Size: 0x%lx \r\n", i, sys_stats.rom_start[i], sys_stats.rom_size[i]); 00053 } 00054 } 00055 } 00056 00057 ~SystemReport(void) 00058 { 00059 free(thread_stats); 00060 } 00061 00062 /** 00063 * Report on each Mbed OS Platform stats API 00064 */ 00065 void report_state(void) 00066 { 00067 report_cpu_stats(); 00068 report_heap_stats(); 00069 report_thread_stats(); 00070 00071 // Clear next line to separate subsequent report logs 00072 printf("\r\n"); 00073 } 00074 00075 /** 00076 * Report CPU idle and awake time in terms of percentage 00077 */ 00078 void report_cpu_stats(void) 00079 { 00080 static uint64_t prev_idle_time = 0; 00081 00082 printf("================= CPU STATS =================\r\n"); 00083 00084 // Collect and print cpu stats 00085 mbed_stats_cpu_get(&cpu_stats); 00086 00087 uint64_t diff = (cpu_stats.idle_time - prev_idle_time); 00088 uint8_t idle = (diff * 100) / (sample_time_ms * 1000); // usec; 00089 uint8_t usage = 100 - ((diff * 100) / (sample_time_ms * 1000)); // usec;; 00090 prev_idle_time = cpu_stats.idle_time; 00091 00092 printf("Idle: %d%% Usage: %d%% \r\n", idle, usage); 00093 } 00094 00095 /** 00096 * Report current heap stats. Current heap refers to the current amount of 00097 * allocated heap. Max heap refers to the highest amount of heap allocated 00098 * since reset. 00099 */ 00100 void report_heap_stats(void) 00101 { 00102 printf("================ HEAP STATS =================\r\n"); 00103 00104 // Collect and print heap stats 00105 mbed_stats_heap_get(&heap_stats); 00106 00107 printf("Current heap: %lu\r\n", heap_stats.current_size); 00108 printf("Max heap size: %lu\r\n", heap_stats.max_size); 00109 } 00110 00111 /** 00112 * Report active thread stats 00113 */ 00114 void report_thread_stats(void) 00115 { 00116 printf("================ THREAD STATS ===============\r\n"); 00117 00118 // Collect and print running thread stats 00119 int count = mbed_stats_thread_get_each(thread_stats, max_thread_count); 00120 00121 for (int i = 0; i < count; i++) { 00122 printf("ID: 0x%lx \r\n", thread_stats[i].id); 00123 printf("Name: %s \r\n", thread_stats[i].name); 00124 printf("State: %ld \r\n", thread_stats[i].state); 00125 printf("Priority: %ld \r\n", thread_stats[i].priority); 00126 printf("Stack Size: %ld \r\n", thread_stats[i].stack_size); 00127 printf("Stack Space: %ld \r\n", thread_stats[i].stack_space); 00128 } 00129 } 00130 }; 00131 00132 #endif // STATS_REPORT_H
Generated on Thu Jul 14 2022 00:45:43 by
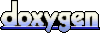