This library can be used to control a low-cost Adafruit 358 TFT display. It has basic functionality but is a starting point for others trying to control this type of display using the FRDM-K64F.
Adafruit_358.h
00001 /* Display library for Adafruit 358 TFT display written for the FRDM-K64F 00002 * 00003 * Copyright (c) 2014 Brian Mazzeo 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 * 00006 * This 1.8" display has 128x160 color pixels 00007 */ 00008 00009 #ifndef ADAFRUIT_358_H 00010 #define ADAFRUIT_358_H 00011 00012 #include "mbed.h" 00013 #include "GraphicsDisplay.h" 00014 00015 // These controls are very useful and retrived from Paul's Adafruit_ST7735.h library 00016 #define ST7735_TFTWIDTH 128 00017 #define ST7735_TFTHEIGHT 160 00018 00019 #define ST7735_NOP 0x00 00020 #define ST7735_SWRESET 0x01 00021 #define ST7735_RDDID 0x04 00022 #define ST7735_RDDST 0x09 00023 00024 #define ST7735_SLPIN 0x10 00025 #define ST7735_SLPOUT 0x11 00026 #define ST7735_PTLON 0x12 00027 #define ST7735_NORON 0x13 00028 00029 #define ST7735_INVOFF 0x20 00030 #define ST7735_INVON 0x21 00031 #define ST7735_DISPOFF 0x28 00032 #define ST7735_DISPON 0x29 00033 #define ST7735_CASET 0x2A 00034 #define ST7735_RASET 0x2B 00035 #define ST7735_RAMWR 0x2C 00036 #define ST7735_RAMRD 0x2E 00037 00038 #define ST7735_PTLAR 0x30 00039 #define ST7735_COLMOD 0x3A 00040 #define ST7735_MADCTL 0x36 00041 00042 #define ST7735_FRMCTR1 0xB1 00043 #define ST7735_FRMCTR2 0xB2 00044 #define ST7735_FRMCTR3 0xB3 00045 #define ST7735_INVCTR 0xB4 00046 #define ST7735_DISSET5 0xB6 00047 00048 #define ST7735_PWCTR1 0xC0 00049 #define ST7735_PWCTR2 0xC1 00050 #define ST7735_PWCTR3 0xC2 00051 #define ST7735_PWCTR4 0xC3 00052 #define ST7735_PWCTR5 0xC4 00053 #define ST7735_VMCTR1 0xC5 00054 00055 #define ST7735_RDID1 0xDA 00056 #define ST7735_RDID2 0xDB 00057 #define ST7735_RDID3 0xDC 00058 #define ST7735_RDID4 0xDD 00059 00060 #define ST7735_PWCTR6 0xFC 00061 00062 #define ST7735_GMCTRP1 0xE0 00063 #define ST7735_GMCTRN1 0xE1 00064 00065 // Color definitions 00066 #define ST7735_BLACK 0x0000 00067 #define ST7735_GREEN 0x07E0 00068 #define ST7735_CYAN 0x07FF 00069 #define ST7735_MAGENTA 0xF81F 00070 #define ST7735_WHITE 0xFFFF 00071 #define ST7735_BLUE 0xFFE0 00072 #define ST7735_RED 0x001F 00073 #define ST7735_YELLOW 0x07FF 00074 00075 00076 class Adafruit_358 : public GraphicsDisplay { 00077 public: 00078 00079 Adafruit_358(PinName MOSI, PinName MISO, PinName SCLK, PinName CS, PinName RESET, PinName DC, const char* name ="TFT"); 00080 00081 void screen_reset(void); // for ST7735B displays 00082 void wr_cmd(unsigned char cmd); 00083 void fillrect(int x0, int y0, int x1, int y1, uint16_t color); 00084 00085 virtual int width(void); 00086 virtual int height(void); 00087 virtual void pixel(int x, int y, int color); 00088 00089 virtual void cls(void); 00090 00091 void set_font(unsigned char* f); 00092 00093 SPI _spi; 00094 DigitalOut _cs; 00095 DigitalOut _reset; 00096 DigitalOut _dc; 00097 unsigned char* font; 00098 00099 private: 00100 00101 unsigned int char_x; 00102 unsigned int char_y; 00103 00104 void WindowMax (void); 00105 00106 }; 00107 00108 #endif
Generated on Wed Jul 20 2022 18:22:44 by
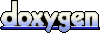