Sharp GP2 familly distance sensor library
Dependents: FRC_2018 0hackton_08_06_18 0hackton_08_06_18_publish Kenya_2019 ... more
GP2A.h
00001 /** 00002 * @author Hugues Angelis 00003 * 00004 * @section LICENSE 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 * 00024 * @section DESCRIPTION 00025 * 00026 * SHARP GP2 Analog sensor familly library 00027 * 00028 * Any GP2 analog sensor may be added to this library using the constructor : 00029 * minDistance : is the minimum distance for linear approximation (cm) 00030 * maxDistance : is the maximum distance for linear approximation (cm) 00031 * slope : is the slope of the linear part of the graph V = f(1/d) 00032 * take special care that we use the 1/d instead of d for x 00033 * axis. 00034 * origin : is the value of the origin (Y for X = 0) of the line of 00035 * V=f(1/d) 00036 * The slope parameter must be computed by user unless the GP2 reference is 00037 * listed in the list below : 00038 * - GP2Y0A02YK0F (Min = 30cm, Max = 150cm, Slope = 60, origin = 0) 00039 * - GP2Y0A21YK0F (Min = 7cm, Max = 80cm, Slope = 0.246, origin = -0.297) 00040 * 00041 * You may add others sensors if you wish it 00042 * 00043 * One must be aware that under the minimum distance the sensor will output 00044 * a false value of distance leading to strong errors. 00045 * @endsection 00046 */ 00047 00048 #ifndef GP2A_H 00049 #define GP2A_H 00050 00051 /** 00052 * Slope definitions for common GP2A sensors 00053 */ 00054 00055 #define GP2Y0A02YK0F_SLOPE 60.0 00056 #define GP2Y0A21YK0F_SLOPE 0.23625 00057 #define GP2Y0A02YK0F_ORIGIN 0.0 00058 #define GP2Y0A21YK0F_ORIGIN -0.297 00059 00060 /** 00061 * Includes : Mbed library 00062 */ 00063 #include "mbed.h" 00064 00065 /** 00066 * GP2A Sensor : See sharp website for more informations 00067 * http://www.sharp-world.com/products/device/lineup/selection/opto/haca/diagram.html 00068 * @param slope = 60 for a GP2Y0A02 and 0.236 for a GP2Y0A21 00069 * @param origin = 0 for a GP2Y0A02 and -0.297 for a GP2Y0A21 00070 */ 00071 class GP2A { 00072 00073 public : 00074 00075 /** 00076 * Constructor of a Sharp GP2 Familly object. 00077 * 00078 * @param vmes (PinName) : the Mbed pin used to connect with GP2A sensor 00079 * @param dMin (double) : the GP2A sensor min distance to mesure 00080 * @param dMax (double) : the GP2A sensor max distance to mesure 00081 * @param slope (double) : the slope of the linear part of the graph V = f(1/d) 00082 * @param origin (double) : the origin (Y for X=0) of the linear pat of the graph V = f(1/d) 00083 * @note you better use slope and origin value that I've put above as sybolic constants 00084 */ 00085 GP2A(PinName vmes, float dMin, float dMax, float slope, float origin = 0); 00086 00087 /** 00088 * Return the distance to target mesured by sensor in cm 00089 * 00090 * @return Distance in cm 00091 * @note Distance is (very) approximative, to have a better mesurment I recommand an averaging of at least 5 measures 00092 * @note you may get a new mesurment every 50ms 00093 */ 00094 double getDistance (void); 00095 00096 /** 00097 * Return the current voltage on GP2A output 00098 * 00099 * @return Voltage between 0 and 3.3V 00100 */ 00101 double getVoltage (void); 00102 00103 /** 00104 * A short hand of getDistance 00105 */ 00106 operator double(); 00107 00108 00109 00110 private : 00111 float m_dMin, m_dMax, m_slope, m_origin; 00112 00113 protected : 00114 00115 AnalogIn _sensor ; 00116 00117 }; 00118 #endif //GP2A_H
Generated on Tue Jul 19 2022 01:17:47 by
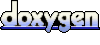