
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
ff_rtos_helpers.cpp
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /****************************************************************************** 00018 * Includes 00019 *****************************************************************************/ 00020 00021 #include "ff.h" 00022 #include "mbed.h" 00023 #include "rtos.h" 00024 00025 /****************************************************************************** 00026 * Defines and typedefs 00027 *****************************************************************************/ 00028 00029 /****************************************************************************** 00030 * External global variables 00031 *****************************************************************************/ 00032 00033 /****************************************************************************** 00034 * Local variables 00035 *****************************************************************************/ 00036 00037 /****************************************************************************** 00038 * Global Functions 00039 *****************************************************************************/ 00040 00041 #if _FS_REENTRANT 00042 /*------------------------------------------------------------------------*/ 00043 /* Create a Synchronization Object */ 00044 /*------------------------------------------------------------------------*/ 00045 /* This function is called in f_mount function to create a new 00046 / synchronization object, such as semaphore and mutex. When a zero is 00047 / returned, the f_mount function fails with FR_INT_ERR. 00048 */ 00049 00050 int ff_cre_syncobj ( /* TRUE:Function succeeded, FALSE:Could not create due to any error */ 00051 BYTE vol, /* Corresponding logical drive being processed */ 00052 _SYNC_t *sobj /* Pointer to return the created sync object */ 00053 ) 00054 { 00055 int ret; 00056 00057 // *sobj = CreateMutex(NULL, FALSE, NULL); /* Win32 */ 00058 // ret = (*sobj != INVALID_HANDLE_VALUE); 00059 00060 // *sobj = SyncObjects[vol]; /* uITRON (give a static sync object) */ 00061 // ret = 1; /* The initial value of the semaphore must be 1. */ 00062 00063 // *sobj = OSMutexCreate(0, &err); /* uC/OS-II */ 00064 // ret = (err == OS_NO_ERR); 00065 00066 // *sobj = xSemaphoreCreateMutex(); /* FreeRTOS */ 00067 // ret = (*sobj != NULL); 00068 00069 *sobj = new Mutex(); /* MBED RTOS */ 00070 // *sobj = new Semaphore(1); /* MBED RTOS */ 00071 ret = (*sobj != NULL); 00072 00073 return ret; 00074 } 00075 00076 00077 00078 /*------------------------------------------------------------------------*/ 00079 /* Delete a Synchronization Object */ 00080 /*------------------------------------------------------------------------*/ 00081 /* This function is called in f_mount function to delete a synchronization 00082 / object that created with ff_cre_syncobj function. When a zero is 00083 / returned, the f_mount function fails with FR_INT_ERR. 00084 */ 00085 00086 int ff_del_syncobj ( /* TRUE:Function succeeded, FALSE:Could not delete due to any error */ 00087 _SYNC_t sobj /* Sync object tied to the logical drive to be deleted */ 00088 ) 00089 { 00090 BOOL ret; 00091 00092 // ret = CloseHandle(sobj); /* Win32 */ 00093 00094 // ret = 1; /* uITRON (nothing to do) */ 00095 00096 // OSMutexDel(sobj, OS_DEL_ALWAYS, &err); /* uC/OS-II */ 00097 // ret = (err == OS_NO_ERR); 00098 00099 // ret = 1; /* FreeRTOS (nothing to do) */ 00100 00101 if (sobj != NULL) { /* MBED RTOS */ 00102 free(sobj); 00103 } 00104 ret = 1; 00105 00106 return ret; 00107 } 00108 00109 00110 00111 /*------------------------------------------------------------------------*/ 00112 /* Request Grant to Access the Volume */ 00113 /*------------------------------------------------------------------------*/ 00114 /* This function is called on entering file functions to lock the volume. 00115 / When a zero is returned, the file function fails with FR_TIMEOUT. 00116 */ 00117 00118 int ff_req_grant ( /* TRUE:Got a grant to access the volume, FALSE:Could not get a grant */ 00119 _SYNC_t sobj /* Sync object to wait */ 00120 ) 00121 { 00122 int ret; 00123 00124 // ret = (WaitForSingleObject(sobj, _FS_TIMEOUT) == WAIT_OBJECT_0); /* Win32 */ 00125 00126 // ret = (wai_sem(sobj) == E_OK); /* uITRON */ 00127 00128 // OSMutexPend(sobj, _FS_TIMEOUT, &err)); /* uC/OS-II */ 00129 // ret = (err == OS_NO_ERR); 00130 00131 // ret = (xSemaphoreTake(sobj, _FS_TIMEOUT) == pdTRUE); /* FreeRTOS */ 00132 00133 ret = ((*((Mutex*)sobj)).lock(_FS_TIMEOUT) == osOK); /* MBED RTOS */ 00134 // static volatile osThreadId lastid = Thread::gettid(); 00135 // ret = ((*((Semaphore*)sobj)).wait(_FS_TIMEOUT) == 1); /* MBED RTOS */ 00136 00137 return ret; 00138 } 00139 00140 00141 00142 /*------------------------------------------------------------------------*/ 00143 /* Release Grant to Access the Volume */ 00144 /*------------------------------------------------------------------------*/ 00145 /* This function is called on leaving file functions to unlock the volume. 00146 */ 00147 00148 void ff_rel_grant ( 00149 _SYNC_t sobj /* Sync object to be signaled */ 00150 ) 00151 { 00152 // ReleaseMutex(sobj); /* Win32 */ 00153 00154 // sig_sem(sobj); /* uITRON */ 00155 00156 // OSMutexPost(sobj); /* uC/OS-II */ 00157 00158 // xSemaphoreGive(sobj); /* FreeRTOS */ 00159 00160 (*((Mutex*)sobj)).unlock(); /* MBED RTOS */ 00161 // (*((Semaphore*)sobj)).release(); /* MBED RTOS */ 00162 } 00163 00164 #endif 00165 00166 00167 00168 00169 #if _USE_LFN == 3 /* LFN with a working buffer on the heap */ 00170 /*------------------------------------------------------------------------*/ 00171 /* Allocate a memory block */ 00172 /*------------------------------------------------------------------------*/ 00173 /* If a NULL is returned, the file function fails with FR_NOT_ENOUGH_CORE. 00174 */ 00175 00176 void* ff_memalloc ( /* Returns pointer to the allocated memory block */ 00177 UINT size /* Number of bytes to allocate */ 00178 ) 00179 { 00180 return malloc(size); 00181 } 00182 00183 00184 /*------------------------------------------------------------------------*/ 00185 /* Free a memory block */ 00186 /*------------------------------------------------------------------------*/ 00187 00188 void ff_memfree( 00189 void* mblock /* Pointer to the memory block to free */ 00190 ) 00191 { 00192 free(mblock); 00193 } 00194 00195 #endif 00196 00197
Generated on Tue Jul 12 2022 14:18:31 by
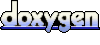