
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
dm_rtc.cpp
00001 /* 00002 * Copyright 2019 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 /****************************************************************************** 00019 * Includes 00020 *****************************************************************************/ 00021 00022 #include "mbed.h" 00023 #include "dm_rtc.h" 00024 #include "mbed_mktime.h" 00025 00026 /****************************************************************************** 00027 * Defines and typedefs 00028 *****************************************************************************/ 00029 00030 /****************************************************************************** 00031 * External global variables 00032 *****************************************************************************/ 00033 00034 /****************************************************************************** 00035 * Local variables 00036 *****************************************************************************/ 00037 00038 /****************************************************************************** 00039 * Local Functions 00040 *****************************************************************************/ 00041 00042 00043 /****************************************************************************** 00044 * Public Functions 00045 *****************************************************************************/ 00046 00047 void dm_init_rtc(void) 00048 { 00049 LPC_SC->PCONP |= 0x200; // Ensure power is on 00050 LPC_RTC->CCR = 0x00; 00051 00052 LPC_RTC->CCR |= 1 << 0; // Ensure the RTC is enabled 00053 } 00054 00055 time_t dm_read_rtc(void) 00056 { 00057 // Setup a tm structure based on the RTC 00058 struct tm timeinfo; 00059 timeinfo.tm_sec = LPC_RTC->SEC; 00060 timeinfo.tm_min = LPC_RTC->MIN; 00061 timeinfo.tm_hour = LPC_RTC->HOUR; 00062 timeinfo.tm_mday = LPC_RTC->DOM; 00063 timeinfo.tm_mon = LPC_RTC->MONTH - 1; 00064 timeinfo.tm_year = LPC_RTC->YEAR - 1900; 00065 00066 // Convert to timestamp 00067 time_t t; 00068 if (_rtc_maketime(&timeinfo, &t, RTC_4_YEAR_LEAP_YEAR_SUPPORT) == false) { 00069 return 0; 00070 } 00071 00072 return t; 00073 } 00074 00075 void dm_write_rtc(time_t t) 00076 { 00077 // Convert the time in to a tm 00078 struct tm timeinfo; 00079 if (_rtc_localtime(t, &timeinfo, RTC_4_YEAR_LEAP_YEAR_SUPPORT) == false) { 00080 return; 00081 } 00082 00083 // Pause clock, and clear counter register (clears us count) 00084 LPC_RTC->CCR |= 2; 00085 00086 // Set the RTC 00087 LPC_RTC->SEC = timeinfo.tm_sec; 00088 LPC_RTC->MIN = timeinfo.tm_min; 00089 LPC_RTC->HOUR = timeinfo.tm_hour; 00090 LPC_RTC->DOM = timeinfo.tm_mday; 00091 LPC_RTC->MONTH = timeinfo.tm_mon + 1; 00092 LPC_RTC->YEAR = timeinfo.tm_year + 1900; 00093 00094 // Restart clock 00095 LPC_RTC->CCR &= ~((uint32_t)2); 00096 } 00097 00098 int dm_isenabled_rtc(void) 00099 { 00100 return(((LPC_RTC->CCR) & 0x01) != 0); 00101 } 00102 00103
Generated on Tue Jul 12 2022 14:18:31 by
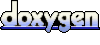