
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
bios.h
00001 #ifndef BIOS_H 00002 #define BIOS_H 00003 00004 #include <stdint.h> 00005 #include <stdbool.h> 00006 00007 #define BIOS_MAGIC 0xEA0123EA 00008 #define BIOS_VER 0x000500 // MAJOR.MINOR.BUILD 00009 #define BIOS_VER_MASK 0xffffff 00010 00011 typedef enum { 00012 BiosError_Ok = 0, 00013 BiosError_ConfigError = 1, 00014 BiosError_WrongBPP = 2, 00015 BiosError_InvalidParam = 3, 00016 BiosError_NoInit = 4, 00017 BiosError_Calibration = 5, 00018 BiosError_Timeout = 6, 00019 BiosError_CommError = 7, 00020 BiosError_NotSupported = 8, 00021 } BiosError_t; 00022 00023 typedef enum { 00024 Res_16bit_rgb565 = 1<<0, 00025 Res_18bit_rgb666 = 1<<1, 00026 Res_24bit_rgb888 = 1<<2, 00027 } Resolution_t; 00028 00029 typedef enum { 00030 FrameRate_Low, 00031 FrameRate_Normal, 00032 FrameRate_High, 00033 } FrameRate_t; 00034 00035 typedef struct { 00036 uint16_t x; 00037 uint16_t y; 00038 uint16_t z; 00039 } touch_coordinate_t; 00040 00041 typedef enum { 00042 TOUCH_IRQ_RISING_EDGE, 00043 TOUCH_IRQ_FALLING_EDGE, 00044 TOUCH_IRQ_HIGH_LEVEL, 00045 TOUCH_IRQ_LOW_LEVEL, 00046 } touch_irq_trigger_t; 00047 00048 typedef void (*delayUsFunc)(int us); 00049 typedef uint32_t (*readTimeMsFunc)(void); 00050 00051 typedef BiosError_t (*initParamFunc)(void* data, uint32_t SystemCoreClock, uint32_t PeripheralClock, delayUsFunc delay, readTimeMsFunc readMs); 00052 typedef BiosError_t (*simpleFunc)(void* data); 00053 typedef BiosError_t (*macFunc)(void* data, char* mac); 00054 00055 typedef BiosError_t (*powerUpFunc)(void* data, void* framebuffer, Resolution_t res, FrameRate_t rate); 00056 typedef BiosError_t (*backlightFunc)(void* data, int percent); 00057 typedef BiosError_t (*infoFuncD)(void* data, 00058 uint16_t* width, 00059 uint16_t* height, 00060 uint16_t* bytesPerPixel, 00061 bool* landscape, 00062 uint16_t* supportedResolutions, 00063 Resolution_t* currentResolution); 00064 typedef BiosError_t (*infoFuncT)(void* data, 00065 bool* supportsTouch, 00066 bool* supportsCalibration, 00067 uint8_t* numPoints); 00068 00069 typedef void (*touchIrqFunc)(uint32_t arg, bool enable, touch_irq_trigger_t trigger); 00070 typedef void (*touchNewDataFunc)(uint32_t arg, touch_coordinate_t* coords, int num); 00071 typedef BiosError_t (*touchInitFunc)(void* data, 00072 touchIrqFunc irqEnabler, uint32_t enablerArg, 00073 touchNewDataFunc newData, uint32_t newDataArg); 00074 typedef BiosError_t (*readFunc)(void* data, touch_coordinate_t* coords, int num); 00075 typedef BiosError_t (*nextFunc)(void* data, uint16_t* x, uint16_t* y, bool* last); 00076 typedef BiosError_t (*waitCalibFunc)(void* data, bool* morePoints, uint32_t timeoutMs); 00077 typedef BiosError_t (*spifiFunc)(void* data, uint8_t mfgr, uint8_t devType, uint8_t devID, uint32_t memSize, bool* known, uint32_t* eraseBlockSize); 00078 00079 typedef struct { 00080 initParamFunc initParams; 00081 simpleFunc i2cIRQHandler; 00082 spifiFunc spifiIsSupported; 00083 macFunc ethernetMac; 00084 00085 simpleFunc displayInit; 00086 powerUpFunc displayPowerUp; 00087 simpleFunc displayPowerDown; 00088 backlightFunc displayBacklight; 00089 infoFuncD displayInformation; 00090 00091 touchInitFunc touchInit; 00092 simpleFunc touchPowerUp; 00093 simpleFunc touchPowerDown; 00094 readFunc touchRead; 00095 simpleFunc touchCalibrateStart; 00096 nextFunc touchGetNextCalibPoint; 00097 waitCalibFunc touchWaitForCalibratePoint; 00098 simpleFunc touchIrqHandler; 00099 infoFuncT touchInformation; 00100 00101 } bios_header_t; 00102 00103 typedef struct { 00104 uint32_t magic; 00105 uint32_t size; 00106 uint32_t crc; 00107 uint32_t version; 00108 uint32_t paramSize; 00109 uint32_t headerSize; 00110 bios_header_t header; 00111 } file_header_t; 00112 00113 #endif /* BIOS_H */
Generated on Tue Jul 12 2022 14:18:31 by
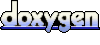