
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
TouchPanel.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef TOUCHPANEL_H 00018 #define TOUCHPANEL_H 00019 00020 #include "bios.h" 00021 00022 /** 00023 * An abstract class that represents touch panels. 00024 */ 00025 class TouchPanel { 00026 public: 00027 00028 enum TouchError { 00029 TouchError_Ok = BiosError_Ok, 00030 TouchError_ConfigError = BiosError_ConfigError, 00031 TouchError_WrongBPP = BiosError_WrongBPP, 00032 TouchError_InvalidParam = BiosError_InvalidParam, 00033 TouchError_NoInit = BiosError_NoInit, 00034 TouchError_CalibrationError = BiosError_Calibration, 00035 TouchError_Timeout = BiosError_Timeout, 00036 TouchError_TouchNotSupported = BiosError_NotSupported, 00037 TouchError_MemoryError, 00038 }; 00039 00040 /** 00041 * Read coordinates from the touch panel. 00042 * 00043 * In case of multitouch (capacitive touch screen) only the first touch 00044 * will be returned. 00045 * 00046 * @param coord pointer to coordinate object. The read coordinates will be 00047 * written to this object. 00048 * 00049 * @returns 00050 * Ok on success 00051 * An error code on failure 00052 */ 00053 virtual TouchError read(touch_coordinate_t &coord) = 0; 00054 00055 /** 00056 * Read up to num coordinates from the touch panel. 00057 * 00058 * @param coords a list of at least num coordinates 00059 * @param num the number of coordinates to read 00060 * 00061 * @returns 00062 * Ok on success 00063 * An error code on failure 00064 */ 00065 virtual TouchError read(touch_coordinate_t* coord, int num) = 0; 00066 00067 /** 00068 * Returns information about the touch panel 00069 * 00070 * @param resistive true for Resistive, false for Capacitive 00071 * @param maxPoints the maximum number of simultaneous touches 00072 * @param calibration true if the controller can be calibrated 00073 * 00074 * @returns 00075 * Ok on success 00076 * An error code on failure 00077 */ 00078 virtual TouchError info(bool* resistive, int* maxPoints, bool* calibrated) = 0; 00079 00080 /** 00081 * Start to calibrate the display 00082 * 00083 * @returns 00084 * Ok on success 00085 * An error code on failure 00086 */ 00087 virtual TouchError calibrateStart() = 0; 00088 00089 /** 00090 * Get the next calibration point. Draw an indicator on the screen 00091 * at the coordinates and ask the user to press/click on the indicator. 00092 * Please note that waitForCalibratePoint() must be called after this 00093 * method. 00094 * 00095 * @param x the x coordinate is written to this argument 00096 * @param y the y coordinate is written to this argument 00097 * @param last true if this is the last coordinate in the series 00098 * 00099 * @returns 00100 * Ok on success 00101 * An error code on failure 00102 */ 00103 virtual TouchError getNextCalibratePoint(uint16_t* x, uint16_t* y, bool* last=NULL) = 0; 00104 00105 /** 00106 * Wait for a calibration point to have been pressed and recored. 00107 * This method must be called just after getNextCalibratePoint(). 00108 * 00109 * @param morePoints true is written to this argument if there 00110 * are more calibrations points available; otherwise it will be false 00111 * @param timeout maximum number of milliseconds to wait for 00112 * a calibration point. Set this argument to 0 to wait indefinite. 00113 * 00114 * @returns 00115 * Ok on success 00116 * An error code on failure 00117 */ 00118 virtual TouchError waitForCalibratePoint(bool* morePoints, uint32_t timeout) = 0; 00119 00120 virtual void setListener(Callback<void()> listener) = 0; 00121 }; 00122 00123 #endif
Generated on Tue Jul 12 2022 14:18:31 by
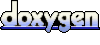