
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
RAMFileSystem.cpp
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "RAMFileSystem.h" 00018 #include "DMBoard.h" 00019 00020 00021 #define RAMFS_DBG(...) 00022 //#define RAMFS_DBG(...) DMBoard::instance().logger()->isr_printf(__VA_ARGS__) 00023 00024 #define SECTOR_SIZE 512 00025 00026 RAMFileSystem::RAMFileSystem(uint32_t addr, uint32_t size, const char* name) : 00027 FATFileSystem(name) { 00028 memStart = addr; 00029 memSize = size - (size % SECTOR_SIZE); 00030 status = 1; //1: disk not initialized 00031 } 00032 00033 int RAMFileSystem::disk_initialize() { 00034 RAMFS_DBG("init RAM fs\n"); 00035 status = 0; //OK 00036 return status; 00037 } 00038 00039 int RAMFileSystem::disk_write(const uint8_t *buffer, uint64_t sector, uint8_t count) { 00040 RAMFS_DBG("write to sector(s) %llu..%llu\n", sector, sector+count); 00041 if ((sector+count-1) >= disk_sectors()) { 00042 return 1; 00043 } 00044 00045 memcpy((uint8_t*)(memStart + SECTOR_SIZE*((uint32_t)sector)), buffer, SECTOR_SIZE*count); 00046 return 0; 00047 } 00048 00049 int RAMFileSystem::disk_read(uint8_t *buffer, uint64_t sector, uint8_t count) { 00050 RAMFS_DBG("read from sector(s) %llu..%llu\n", sector, sector+count); 00051 if ((sector+count-1) >= disk_sectors()) { 00052 return 1; 00053 } 00054 00055 memcpy(buffer, (uint8_t*)(memStart + SECTOR_SIZE*((uint32_t)sector)), SECTOR_SIZE*count); 00056 return 0; 00057 } 00058 00059 int RAMFileSystem::disk_status() { 00060 RAMFS_DBG("disk status %d\n", status); 00061 return status; 00062 } 00063 int RAMFileSystem::disk_sync() { 00064 return 0; 00065 } 00066 uint64_t RAMFileSystem::disk_sectors() { 00067 RAMFS_DBG("returning fs has %u sectors\n", memSize/SECTOR_SIZE); 00068 return memSize/SECTOR_SIZE; 00069 } 00070 00071 uint64_t RAMFileSystem::disk_size() { 00072 return memSize; 00073 }
Generated on Tue Jul 12 2022 14:18:31 by
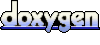