
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
InternalEEPROM.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef INTERNAL_EEPROM_H 00018 #define INTERNAL_EEPROM_H 00019 00020 #include "mbed.h" 00021 00022 /** 00023 * Internal EEPROM Driver 00024 */ 00025 class InternalEEPROM { 00026 public: 00027 00028 enum Constants { 00029 EEPROM_MEMORY_SIZE = 4032, 00030 EEPROM_PAGE_SIZE = 64, 00031 EEPROM_NUM_PAGES = EEPROM_MEMORY_SIZE/EEPROM_PAGE_SIZE, 00032 }; 00033 00034 InternalEEPROM(); 00035 ~InternalEEPROM(); 00036 00037 /** Initializes the EEPROM 00038 * 00039 * @returns 00040 * Ok on success 00041 * An error code on failure 00042 */ 00043 void init(); 00044 00045 /** Put the internal EEPROM in a low power state 00046 */ 00047 void powerDown(); 00048 00049 /** Reads size bytes from offset addr 00050 * 00051 * Note that this function will power up the EEPROM so it is 00052 * recommended to call powerDown() when finished reading. 00053 * 00054 * @param addr the offset to read from 00055 * @param data buffer to store the read data in 00056 * @param size number of bytes to read 00057 * 00058 * @returns 00059 * The number of bytes read 00060 */ 00061 int read(uint32_t addr, uint8_t* data, uint32_t size); 00062 00063 /** Writes size bytes to offset addr 00064 * 00065 * Note that this function will power up the EEPROM so it is 00066 * recommended to call powerDown() when finished writing. 00067 * 00068 * @param addr the offset to write to 00069 * @param data the data to write 00070 * @param size number of bytes to write 00071 * 00072 * @returns 00073 * The number of bytes written 00074 */ 00075 int write(uint32_t addr, const uint8_t* data, uint32_t size); 00076 00077 /** Returns the size (in bytes) of the internal EEPROM 00078 * 00079 * @returns 00080 * The size in bytes 00081 */ 00082 uint32_t memorySize() { return EEPROM_MEMORY_SIZE; } 00083 00084 private: 00085 00086 bool _initialized; 00087 00088 void powerUp(); 00089 void clearInterrupt(uint32_t mask); 00090 void waitForInterrupt(uint32_t mask); 00091 void setAddr(uint32_t pageAddr, uint32_t pageOffset); 00092 void setCmd(uint32_t cmd); 00093 void readPage(uint32_t pageAddr, uint32_t pageOffset, uint8_t* buf, uint32_t size); 00094 void writePage(uint32_t pageAddr, uint32_t pageOffset, const uint8_t* buf, uint32_t size); 00095 void eraseOrProgramPage(uint32_t pageAddr); 00096 }; 00097 00098 #endif
Generated on Tue Jul 12 2022 14:18:31 by
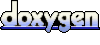